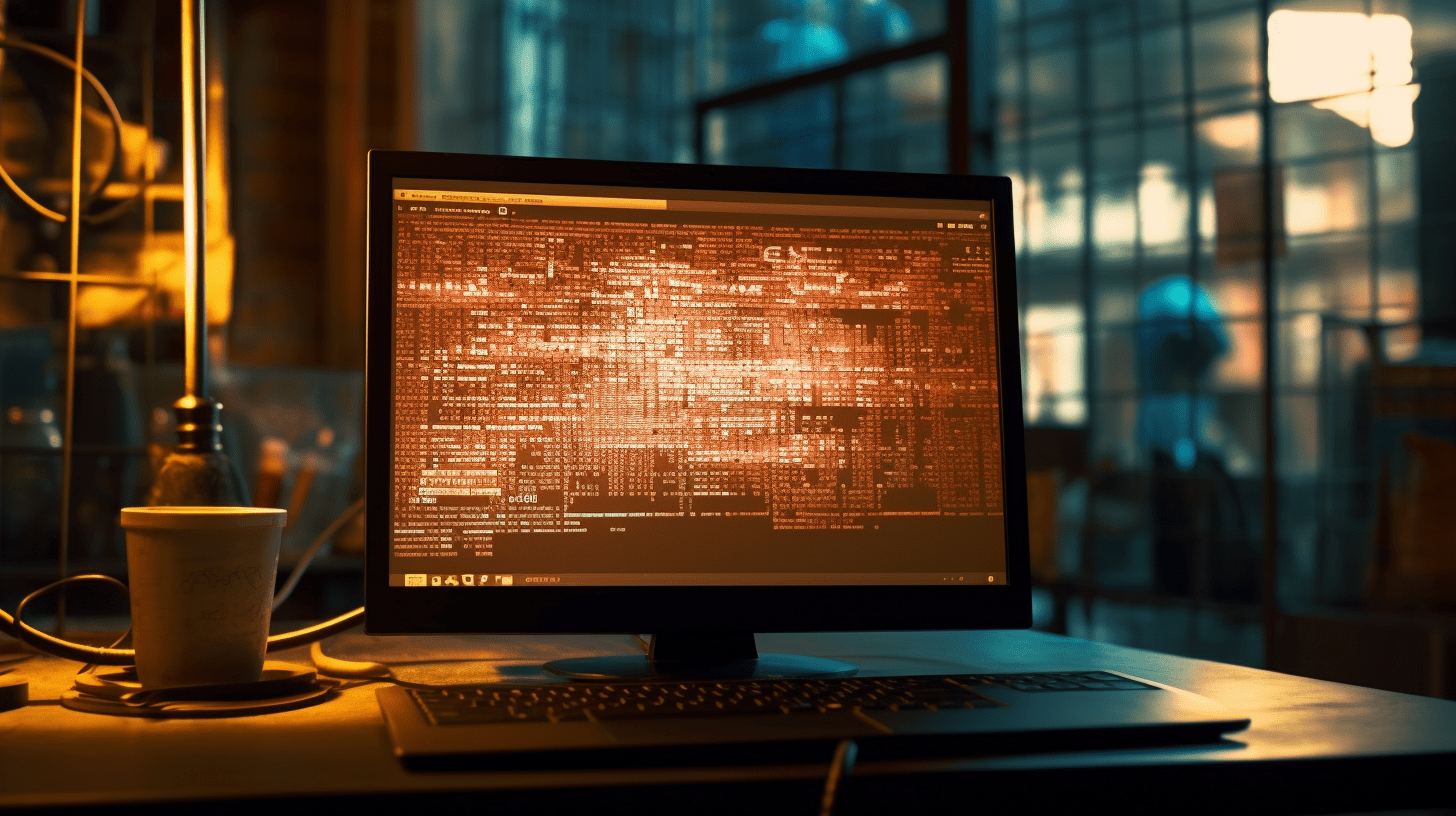
Swift and PDFKit
PDFKit is a powerful framework provided by Apple, allowing developers to work with PDF documents in a seamless and efficient manner. It offers a comprehensive set of tools for rendering, displaying, and manipulating PDF files, making it an essential resource for any macOS or iOS application that handles documents. Understanding the core components of PDFKit very important for using its capabilities effectively.
At the heart of PDFKit is the PDFDocument class, which represents a PDF file. This class encapsulates the entire document, enabling you to load, render, and manipulate its contents. Once you have a PDFDocument, you can access its pages using the page(at:) method, which returns a PDFPage instance for the specified page index.
let pdfURL = Bundle.main.url(forResource: "sample", withExtension: "pdf")! if let pdfDocument = PDFDocument(url: pdfURL) { // Successfully loaded PDF document let pageCount = pdfDocument.pageCount print("This document has (pageCount) pages.") } else { print("Failed to load PDF document.") }
The PDFPage class serves as the individual pages of the PDF document. Each page can be rendered onto a view, allowing users to interact with the PDF visually. PDFKit provides a default view, PDFView, which can display the document content conveniently.
let pdfView = PDFView(frame: self.view.bounds) pdfView.autoScales = true pdfView.document = pdfDocument self.view.addSubview(pdfView)
Moreover, PDFKit supports text selection, annotation, and even form filling, significantly enhancing user interaction with documents. The framework includes classes such as PDFAnnotation, which can be added to pages for highlights, notes, or other markup purposes.
Another critical aspect of PDFKit is its support for accessibility. By integrating PDF annotations and ensuring that text can be read by screen readers, developers can make their applications more inclusive. In this regard, understanding how to manipulate annotations becomes essential.
With PDFKit, developers can also dive deeper into PDF properties, such as metadata, outline, and document permissions. The PDFDocument class provides methods to access and modify these properties easily, allowing for a more dynamic interaction with the PDF files.
Getting Started: Setting Up Your First PDF Document
To set up your first PDF document using PDFKit, you need to follow a few simpler steps. First, ensure you have imported PDFKit into your Swift file. This allows you to access the classes and methods provided by the framework seamlessly.
import PDFKit
The next step is to create a PDFDocument instance. You’ll typically load a PDF file from your app bundle or another source. Here’s how you can do that:
guard let pdfURL = Bundle.main.url(forResource: "sample", withExtension: "pdf") else { print("PDF file not found.") return } guard let pdfDocument = PDFDocument(url: pdfURL) else { print("Failed to load PDF document.") return }
Once you have your PDFDocument, it is crucial to render it for display. PDFKit provides a convenient way to do this with the PDFView class. You can instantiate a PDFView, set its document property to your loaded PDFDocument, and add it to your view hierarchy. Here’s a simple example of how to achieve this:
let pdfView = PDFView(frame: self.view.bounds) pdfView.autoScales = true pdfView.document = pdfDocument self.view.addSubview(pdfView)
This code snippet sets the PDFView to fill the entire view of your app’s screen while automatically scaling the PDF content to fit. This auto-scaling feature is particularly useful for ensuring that users can easily read the text and view the images within the PDF, regardless of the original dimensions of the document.
PDFKit also allows you to listen for user interactions with the PDFView. For example, you can respond to events such as page changes or user selection of text. This capability is vital for creating interactive applications that require user engagement with the PDF content. To demonstrate how to track page changes, you can implement the following delegate method:
extension YourViewController: PDFViewDelegate { func pdfViewWillChangePage(_ sender: PDFView) { let currentPage = sender.currentPage print("Current page is now: (currentPage?.pageLabel ?? "Unknown")") } }
Don’t forget to set your ViewController as the delegate of your PDFView:
Manipulating PDFs: Essential Techniques and Best Practices
Manipulating PDFs with PDFKit involves a range of techniques that can significantly enhance user experience and functionality within your applications. One of the essential operations is adding or modifying annotations on PDF pages, which allows users to interact with the content meaningfully. PDFKit provides the PDFAnnotation class to facilitate this.
To add an annotation, you first need to create an instance of PDFAnnotation and specify its properties such as type, bounds, and content. PDFKit supports various annotation types, including highlights, notes, and free text. Here’s an example of how to create a simple highlight annotation:
let highlight = PDFAnnotation(bounds: CGRect(x: 100, y: 100, width: 200, height: 50), forType: .highlight, withProperties: nil) highlight.color = UIColor.yellow.withAlphaComponent(0.5) highlight.contents = "Important Note" if let page = pdfDocument.page(at: 0) { page.addAnnotation(highlight) }
In this code snippet, we create a highlight annotation positioned at specific coordinates on the first page of the PDF document. The highlight’s color is set to a semi-transparent yellow, making it visually distinct while not obscuring the underlying text.
Another critical manipulation technique is extracting text from the PDF. This is particularly useful for applications that need to search through text or display it in a more interactive format. PDFDocument provides the method string(from:), which retrieves text from a specified rectangle within a page. Here’s an example:
if let page = pdfDocument.page(at: 0) { let textRect = CGRect(x: 50, y: 50, width: 300, height: 200) let extractedText = page.string(from: textRect) print("Extracted Text: (extractedText ?? "No text found")") }
This snippet extracts text within a defined rectangle on the first page of the PDF. It is a simpler way to access specific content, which can then be processed or displayed elsewhere in your app.
Additionally, managing document permissions and metadata especially important for ensuring compliance and enhancing the user experience. You can manipulate PDF metadata, such as title, author, and subject, by modifying the document attributes. Here’s how to retrieve and update the title:
if var attributes = pdfDocument.documentAttributes { attributes[.title] = "New Document Title" pdfDocument.documentAttributes = attributes }
In this example, we retrieve the current document attributes, modify the title, and then set the updated attributes back to the PDFDocument. This capability is vital when you need to provide context or branding for your documents.
Form filling is another powerful feature within PDFKit that allows you to interact with interactive PDF forms. You can access form fields and set their values programmatically. Here’s a basic example of how to fill a text field:
if let page = pdfDocument.page(at: 0) { let field = page.annotation(at: 0) as? PDFAnnotation field?.stringValue = "Your Name" }
This snippet assumes that the first annotation on the first page is a text field. By setting the stringValue property, we dynamically populate the form field, enhancing user interaction.
Lastly, don’t overlook the importance of handling user interactions effectively. PDFKit allows you to implement custom behaviors based on user actions, such as tapping on annotations or changing pages. Using the PDFViewDelegate protocol, you can tailor these interactions to fit your app’s flow. For instance, responding to selection changes can provide immediate feedback:
extension YourViewController: PDFViewDelegate { func pdfViewDidChangeSelection(_ sender: PDFView) { if let selectedText = sender.currentSelection?.string { print("Selected Text: (selectedText)") } } }
Advanced Features: An Exploration of PDF Annotations and Interactivity
One of the most compelling aspects of PDFKit is its robust support for annotations and interactivity, which can dramatically enhance the user experience when interacting with PDF documents. Annotations allow users to mark up documents, add notes, and create interactive elements, making PDFKit a versatile tool for developers looking to create rich document experiences.
PDF annotations are represented by the PDFAnnotation class, which provides a variety of annotation types, such as highlights, text notes, stamps, and free text. Each annotation type has its own specific properties that can be customized to suit your application’s needs. For example, a highlight annotation can be used to emphasize important sections of text, while a free text annotation can allow users to add their own notes anywhere on the page.
To create an annotation, you instantiate a PDFAnnotation object, define its bounds, and then add it to the desired PDFPage. Here’s how you can create a text note annotation:
let noteBounds = CGRect(x: 200, y: 200, width: 150, height: 50) let textNote = PDFAnnotation(bounds: noteBounds, forType: .freeText, withProperties: nil) textNote.contents = "This is a note." textNote.color = UIColor.lightGray textNote.font = UIFont.systemFont(ofSize: 12) textNote.border = PDFBorder(lineWidth: 1.0, style: .solid) if let page = pdfDocument.page(at: 0) { page.addAnnotation(textNote) }
In this example, a free text annotation is created at specific coordinates on the first page. You can customize the text content, color, font, and even the border style to make it visually distinct. Once added, users can click on the annotation to see or edit its content, enhancing the interactivity of the document.
Annotations can also include multimedia elements, such as audio or video files, which can be leveraged to create more engaging experiences. This can be particularly useful in educational applications where audio explanations accompany specific sections of a document.
Another important feature of PDFKit is the ability to handle interactions with annotations. For instance, when a user taps on an annotation, you can implement a delegate method to respond to this action. The following example shows how you can track taps on annotations:
extension YourViewController: PDFViewDelegate { func pdfView(_ pdfView: PDFView, didSelect annotation: PDFAnnotation) { if annotation.type == .freeText { print("Selected Note: (annotation.contents ?? "No content")") } } }
This delegate method allows you to react when an annotation is selected, providing the opportunity to display additional information or options to the user. By doing so, you can create a more interactive and engaging document experience.
Furthermore, PDFKit supports the manipulation of existing annotations, enabling you to edit, remove, or change their properties. For example, if you wanted to change the color of all highlight annotations on a page, you could iterate through the annotations as follows:
if let page = pdfDocument.page(at: 0) { for annotation in page.annotations { if annotation.type == .highlight { annotation.color = UIColor.red.withAlphaComponent(0.5) } } }
This snippet loops through all annotations on the specified page, checking for highlight types and changing their color to red. Such capabilities allow you to implement features like annotation management tools, providing users with the ability to organize and customize their document interactions.
Moreover, PDFKit includes built-in support for form fields. This allows users to interact with PDF forms seamlessly. You can programmatically set values for form fields using the same PDFAnnotation class. For instance, filling a checkbox or a text field can enhance the form-filling experience without users needing to print or manually edit documents:
if let page = pdfDocument.page(at: 0) { if let textField = page.annotation(at: 0) as? PDFAnnotation { textField.stringValue = "Filled in Programmatically" } }
This example assumes the first annotation is a text field. By setting its stringValue, you programmatically fill in the form, making it easier for users to interact with complex forms directly within the PDF.