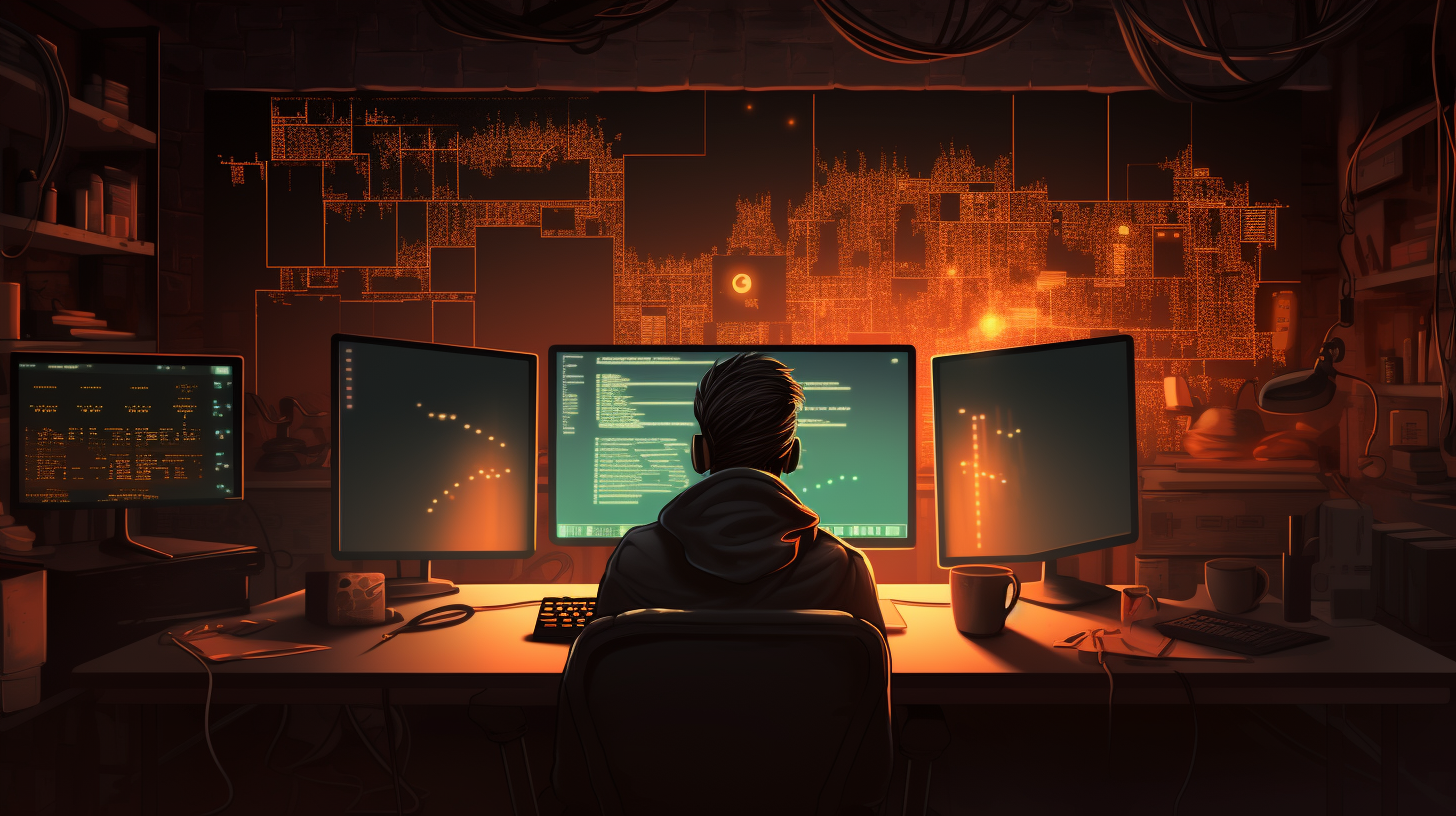
Using Arrays in Bash Scripts
In Bash, arrays are powerful tools that enable you to store and manipulate collections of data. To declare an array, you simply assign values to it using parentheses. This method is simpler but requires attention to detail, particularly with regard to spacing and quotes.
To declare a simple indexed array, you can do the following:
my_array=(value1 value2 value3)
In this example, my_array
is an indexed array with three elements. It’s important to note that array indices start at zero, so ${my_array[0]}
would yield value1
.
If your array contains strings with spaces, you need to quote the individual elements:
my_array=("First value" "Second value" "Third value")
In this scenario, each string is treated as a single element of the array, preserving the internal spaces. When you declare an array this way, it’s essential to use double quotes around each value.
Alternatively, you can also initialize an empty array and populate it later. This technique allows for dynamic array growth:
my_array=() # Initialize an empty array my_array+=("First value") # Add first element my_array+=("Second value") # Add second element
This appending method is particularly useful when building arrays from the output of commands or loops, so that you can capture data dynamically as your script runs.
For associative arrays, which store key-value pairs, the declaration is slightly different. You must first declare the array using the declare
command:
declare -A my_assoc_array # Declare an associative array my_assoc_array[Key1]="Value1" # Assign values my_assoc_array[Key2]="Value2"
With associative arrays, you can access elements using their keys:
echo ${my_assoc_array[Key1]} # Outputs: Value1
Accessing Array Elements
Accessing elements in an array in Bash is a simpler yet critical task that can significantly enhance your scripting capabilities. Once you have declared and initialized an array, you can retrieve its elements using the syntax ${array_name[index]}, where index is the position of the element you want to access. Remember that indexing starts at 0, which means the first element of the array is accessed with index 0.
For instance, if we have already declared an indexed array named my_array as follows:
my_array=("First value" "Second value" "Third value")
You can access each element like this:
echo ${my_array[0]} # Outputs: First value echo ${my_array[1]} # Outputs: Second value echo ${my_array[2]} # Outputs: Third value
When accessing elements, if you try to access an index that doesn’t exist, Bash will simply return an empty string, which can be useful for avoiding errors in certain conditions but might also require careful handling to avoid unintended behavior in your scripts.
For those working with associative arrays, the access pattern is similar, but instead of an index, you will use a key. If we take the associative array example from before:
declare -A my_assoc_array my_assoc_array[Key1]="Value1" my_assoc_array[Key2]="Value2"
You can access the values in the associative array by using the keys:
echo ${my_assoc_array[Key1]} # Outputs: Value1 echo ${my_assoc_array[Key2]} # Outputs: Value2
It is also worth noting that if you attempt to access a key that doesn’t exist in an associative array, Bash will again return an empty string. This characteristic can be utilized in control structures to manage data flow based on the existence of certain keys.
When working with arrays, you might want to check the length of the array, especially in loops or conditional statements. You can easily do this by using the syntax ${#array_name[@]} for indexed arrays or ${#array_name[key]} for associative arrays. Here’s how you can find the length of my_array:
length=${#my_array[@]} echo "The array contains $length elements." # Outputs: The array contains 3 elements.
Looping Through Arrays
# Looping through indexed arrays for i in "${!my_array[@]}"; do echo "Element at index $i is: ${my_array[$i]}" done
Looping through arrays in Bash is a fundamental skill that allows you to process each element of the array systematically. This can be particularly useful for operations that require you to manipulate or evaluate every item within an array.
For indexed arrays, the standard way to loop through the elements is to use a `for` loop combined with the array’s indices. Here’s how you can iterate over an indexed array:
my_array=("First value" "Second value" "Third value") for index in "${!my_array[@]}"; do echo "Element at index $index: ${my_array[$index]}" done
In this code snippet, `${!my_array[@]}` retrieves all the indices of the array, and the loop iterates over each index. The syntax `${my_array[$index]}` is then used to access the value at that index. This method is efficient and allows you to handle dynamic-sized arrays without any extra calculations.
For those who prefer a more simpler approach without directly using indices, you can loop through the array values directly, like so:
for value in "${my_array[@]}"; do echo "Value: $value" done
This method is cleaner when you only need the values and don’t care about the indices. It iterates over each element of the array, giving you direct access to the values.
When working with associative arrays, the looping mechanism is slightly different but follows a similar pattern. You can loop through the keys of the associative array using the following syntax:
declare -A my_assoc_array my_assoc_array[Key1]="Value1" my_assoc_array[Key2]="Value2" my_assoc_array[Key3]="Value3" for key in "${!my_assoc_array[@]}"; do echo "Key: $key, Value: ${my_assoc_array[$key]}" done
Here, `${!my_assoc_array[@]}` retrieves all the keys from the associative array, and the loop iterates over each key. The corresponding value can then be accessed using `${my_assoc_array[$key]}`. This structure is particularly powerful when dealing with datasets that require key-value pair manipulation.
Modifying Arrays
my_array=("First value" "Second value" "Third value") my_array[1]="Modified value" # Change second element my_array+=("Fourth value") # Add another element
Modifying arrays in Bash is a simpler process that can enhance your script’s functionality significantly. Once an array is declared and initialized, you can change existing elements or add new ones with ease. This capability allows your scripts to adapt dynamically as they run, accommodating various data needs.
To modify an existing element in an indexed array, you can simply assign a new value to the desired index. For example, if you want to change the second element of the array my_array, you can do so with the following command:
my_array[1]="Modified value"
After executing this line, if you were to echo out the second element, you would see the update reflected:
echo ${my_array[1]} # Outputs: Modified value
In addition to modifying existing elements, you can also append new elements to an array. That is accomplished using the += operator. For instance, to add a fourth value to my_array, you would use the following syntax:
my_array+=("Fourth value")
Now, if you were to access the new element, you would do:
echo ${my_array[3]} # Outputs: Fourth value
It’s worth noting that appending elements this way allows the array to grow dynamically, accommodating varying amounts of data as your script executes.
When working with associative arrays, the process of modifying values is similarly simpler. You specify the key and assign a new value, just like you would with indexed arrays. As an example:
declare -A my_assoc_array my_assoc_array[Key1]="Value1" my_assoc_array[Key2]="Value2" my_assoc_array[Key1]="Modified Value1" # Modify existing value
In this case, if you echo the value associated with Key1 after the modification, you will see the updated value:
echo ${my_assoc_array[Key1]} # Outputs: Modified Value1
Adding new key-value pairs to an associative array follows the same principle. You can simply assign a value to a new key:
my_assoc_array[Key3]="Value3"
Once you add this new key, it can be accessed just like the others:
echo ${my_assoc_array[Key3]} # Outputs: Value3
Associative Arrays in Bash
Associative arrays in Bash are a powerful feature that allows you to store data in the form of key-value pairs, providing a more intuitive way to manage related data than traditional indexed arrays. To use associative arrays, you must declare them explicitly using the declare -A
command, which sets them apart from regular indexed arrays.
The syntax for declaring an associative array is as follows:
declare -A my_assoc_array
Once declared, you can assign values to specific keys using the following syntax:
my_assoc_array[Key1]="Value1" my_assoc_array[Key2]="Value2"
Accessing values in an associative array is just as simpler. Instead of using an index, you use the key associated with the value you want to retrieve:
echo ${my_assoc_array[Key1]} # Outputs: Value1
This flexibility allows for a more meaningful organization of data, especially when dealing with datasets that rely on unique identifiers. For instance, you can store user information or configuration settings in a way that makes it easy to reference each element by its descriptive key.
Manipulating associative arrays is also simple. You can modify existing values or add new key-value pairs as needed. If you wanted to change the value of Key1
to something else, you would do it like this:
my_assoc_array[Key1]="Modified Value1"
And to add a new entry:
my_assoc_array[Key3]="Value3"
Retrieving the new value is simpler as well:
echo ${my_assoc_array[Key3]} # Outputs: Value3
One of the key advantages of associative arrays is their ability to store complex data relationships in a clean and manageable format. For example, you can easily create a mapping of usernames to user IDs or settings to their respective values, making your scripts much more organized.
When looping through associative arrays, you can access both keys and their corresponding values efficiently. Think the following example:
for key in "${!my_assoc_array[@]}"; do echo "Key: $key, Value: ${my_assoc_array[$key]}" done
In this loop, `${!my_assoc_array[@]}` retrieves all the keys in the associative array, so that you can process each key-value pair seamlessly. This capability is particularly useful for generating reports, configuration validations, or any task that necessitates the evaluation of each entry in the associative array.
Despite their advantages, working with associative arrays in Bash requires attention to detail. For instance, remember that keys are case-sensitive, and using special characters in keys can lead to unexpected behaviors. Always validate input and test your scripts to ensure they handle edge cases gracefully.
Common Pitfalls and Best Practices
When working with arrays in Bash, it’s essential to be aware of common pitfalls and best practices that can save you from headaches down the line. While Bash’s array capabilities are powerful, certain nuances can trip up even seasoned scripters. Here’s a rundown of some key points to think, ensuring that your array manipulation is both efficient and error-free.
1. Quoting Matters
One of the most significant pitfalls involves quoting when dealing with array elements that contain spaces or special characters. If you don’t quote your array accesses properly, you might end up with unexpected results. Always use double quotes when accessing array elements to prevent word splitting and globbing issues. For instance:
my_array=("First Value" "Second Value" "Third Value") echo "${my_array[0]}" # Correctly outputs: First Value
Using the syntax without quotes can lead to errors, especially if the value contains spaces:
echo ${my_array[0]} # Outputs: First Value (but may cause issues in other contexts)
2. Array Indexing
Remember that Bash arrays are zero-indexed. This means that the first element of the array is accessed using index 0. A common mistake is assuming that arrays start from index 1, leading to off-by-one errors:
echo "${my_array[1]}" # Will output: Second Value echo "${my_array[3]}" # Will output an empty string if my_array only has 3 elements
3. Associative Array Keys
When using associative arrays, it is crucial to remember that keys are case-sensitive. “Key1” and “key1” are considered different keys. Always check your key names for consistency, and be cautious if you’re working with user-generated input.
declare -A my_assoc_array my_assoc_array[Key1]="Value1" my_assoc_array[key1]="Value2" echo "${my_assoc_array[Key1]}" # Outputs: Value1 echo "${my_assoc_array[key1]}" # Outputs: Value2
4. Modifying Arrays
When modifying an array, be mindful of how you append new elements or change existing ones. Using the `+=` operator allows you to add new elements dynamically. However, if you mistakenly overwrite an index, you may lose existing data:
my_array=("First" "Second") my_array[1]="Modified" # This modifies the second element echo "${my_array[@]}" # Outputs: First Modified
To append safely, especially when you don’t want to overwrite existing indices, use:
my_array+=("Third") # This adds "Third" to the end of the array
5. Length of Arrays
To work effectively with loops, it’s essential to know the length of your arrays. Use the correct syntax to determine the number of elements:
length=${#my_array[@]} echo "Array length: $length" # Outputs: Array length: 3 for my_array with three values
This can prevent out-of-bounds errors when iterating through the array elements.
6. Use of Indices in Loops
When looping through arrays, especially indexed ones, ensure you’re using the correct syntax to avoid unexpected results. Using `${!array[@]}` to get all indices is standard practice:
for i in "${!my_array[@]}"; do echo "Index $i: ${my_array[$i]}" done
However, remember that if you were to use just `${my_array[@]}` inside a loop, you would be iterating directly over values rather than indices, which can lead to confusion.
7. Debugging Arrays
Finally, when things go wrong, printing the entire array can be a great help for debugging. You can display all elements in an indexed array like this:
echo "${my_array[@]}"
And for associative arrays, you can loop through keys and values to inspect your data structure:
for key in "${!my_assoc_array[@]}"; do echo "Key: $key, Value: ${my_assoc_array[$key]}" done