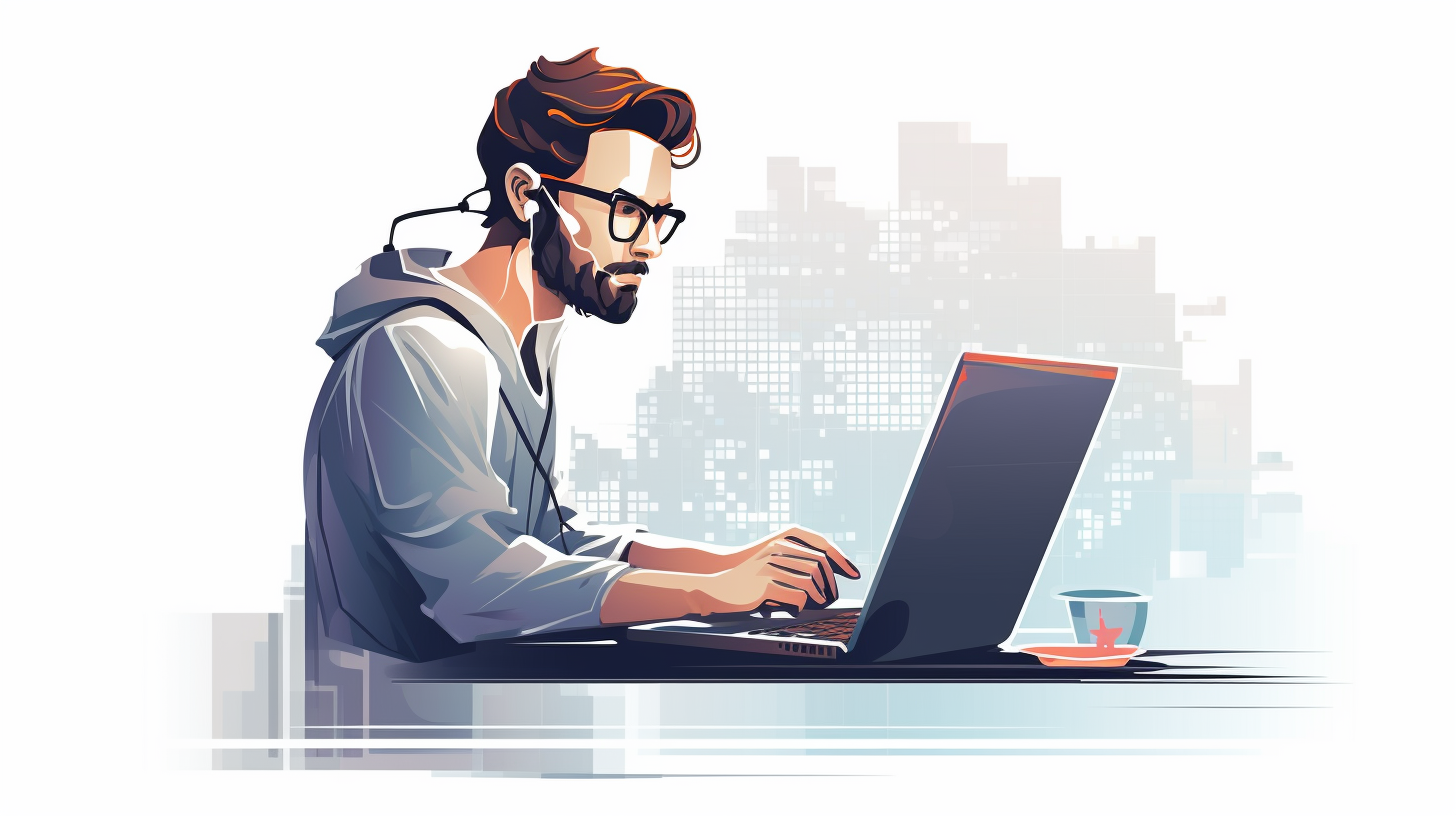
Using UNION and UNION ALL in SQL
The UNION and UNION ALL operators in SQL are pivotal tools for combining the results of two or more SELECT queries. While they serve a similar purpose, there are fundamental differences in their behavior concerning duplicate records and performance.
The UNION operator is used to combine the result sets of two or more SELECT statements into a single result set, automatically removing any duplicate rows. This means that if the same row appears in both result sets, it will only be displayed once in the final output. Here is a basic example:
SELECT city FROM customers UNION SELECT city FROM suppliers;
In this example, the query combines the list of cities from the customers table and the suppliers table, ensuring that each city appears only once in the output.
On the other hand, the UNION ALL operator performs a similar task but retains all duplicate rows. This means that if a city appears in both the customers and suppliers tables, it will show up in the results as many times as it appears in the source tables. For instance:
SELECT city FROM customers UNION ALL SELECT city FROM suppliers;
In this scenario, if “New York” appears in both the customers and suppliers tables, it will be listed twice in the final result set.
To summarize:
- Combines results and eliminates duplicates.
- Combines results and keeps all duplicates.
Differences Between UNION and UNION ALL
The differences between UNION and UNION ALL extend beyond just handling duplicates; they also influence performance and resource consumption during query execution. When you choose between these two operators, it’s essential to recognize how each behaves in various scenarios.
One of the most significant differences lies in the execution logic. The UNION operator must perform additional operations to identify and eliminate duplicate records from the combined result set. This process involves sorting the data and potentially using algorithms that can increase computational overhead, especially with larger datasets. Thus, UNION is typically slower than UNION ALL because of this added complexity.
To illustrate the performance implications, ponder the following example that uses both operators on a hypothetical dataset of employee records:
SELECT employee_id FROM full_time_employees UNION SELECT employee_id FROM part_time_employees;
In this case, SQL Server will need to inspect the combined result set of employee IDs and remove any duplicates, which can be resource-intensive. Conversely, if you use UNION ALL:
SELECT employee_id FROM full_time_employees UNION ALL SELECT employee_id FROM part_time_employees;
SQL Server can directly append the results from the two SELECT statements without any additional overhead for duplicate checking. As a result, the execution time can be significantly reduced, particularly when dealing with large volumes of data.
Another consideration is the type of data returned. When using UNION, the database engine must ensure that the data types across the queries are compatible and that any necessary conversions take place. This can add further complexity and processing time to the execution plan. With UNION ALL, since you are preserving all records as they are, the engine doesn’t need to perform these additional data type checks.
Usage Scenarios for UNION and UNION ALL
The choice between using UNION and UNION ALL can significantly affect the outcome of your SQL queries, depending on the specific context and requirements of your data retrieval tasks. Each operator has its unique scenarios where it shines, and understanding these can help you harness their potential effectively.
When to Use UNION:
Ponder using UNION when you need a distinct list of entries from multiple tables or queries. That is particularly useful in reporting situations where you want to present a clean, unique dataset without any redundant information. For example, if you are generating a report on all the unique product IDs sold by various branches of a retail company, you might structure your query as follows:
SELECT product_id FROM store_a_sales UNION SELECT product_id FROM store_b_sales UNION SELECT product_id FROM store_c_sales;
In this case, the UNION operator will ensure that each product ID appears only once in the final report, regardless of how many stores sold the same product.
When to Use UNION ALL:
On the other hand, UNION ALL is your go-to option when you want to maintain all occurrences of records, including duplicates. That is especially valuable in scenarios where the frequency of data is significant. For example, if you are conducting an analysis of user activity logs across different applications, you might want to retain every instance of a user action:
SELECT user_id, action FROM app_a_activity UNION ALL SELECT user_id, action FROM app_b_activity UNION ALL SELECT user_id, action FROM app_c_activity;
Here, using UNION ALL allows you to capture the full scope of user interactions, providing a richer dataset for analysis. Each user action will be reported in the result set, showing the complete picture of user engagement across applications.
Combining Different Sources:
Another scenario where these operators come into play is when you are combining results from different databases or external data sources. If you are pulling customer data from multiple regions and need to ensure that your final output only includes unique entries, you would use UNION:
SELECT customer_email FROM regional_office_a UNION SELECT customer_email FROM regional_office_b UNION SELECT customer_email FROM regional_office_c;
On the contrary, if you are aggregating transaction logs from these regional offices and need all records for detailed analysis, you would opt for UNION ALL:
SELECT transaction_id FROM regional_office_a_transactions UNION ALL SELECT transaction_id FROM regional_office_b_transactions UNION ALL SELECT transaction_id FROM regional_office_c_transactions;
In this situation, retaining duplicates might reveal patterns and insights that could be lost if you only used UNION.
Performance Considerations in Using UNIONs
When working with UNION and UNION ALL in SQL, performance considerations are paramount, especially in environments where speed and resource efficiency are critical. The choice between these two operators can significantly impact not only the execution time of your queries but also the overall performance of your database system.
One of the primary performance factors to think is the overhead associated with duplicate elimination in UNION. As mentioned previously, UNION necessitates an internal process to identify and remove duplicates from the combined result set. This often involves sorting the data, which can be computationally expensive, particularly with larger datasets. To illustrate this, ponder the following query:
SELECT order_id FROM online_orders UNION SELECT order_id FROM in_store_orders;
Here, SQL must examine the combined set of order IDs, sort them, and then remove any duplicates, which can lead to increased execution time as the size of the dataset grows. This performance hit may not be noticeable with smaller tables, but it can become significant as the volume of records increases.
On the other hand, when you employ UNION ALL, the database is freed from the burden of duplicate checking. This operator simply appends the results of the SELECT statements together, allowing for a more simpler and faster execution process:
SELECT order_id FROM online_orders UNION ALL SELECT order_id FROM in_store_orders;
In this example, the database engine efficiently concatenates the results without the additional sorting and duplicate filtering step. This approach can yield noticeable performance improvements, especially when dealing with large datasets, making UNION ALL the preferred choice in many scenarios.
It’s also important to ponder the execution plan generated by your SQL queries. The SQL optimizer will create different plans for UNION and UNION ALL, which can further affect performance. A query using UNION might result in a more complex execution plan that requires additional resources, whereas a query using UNION ALL can lead to simpler plans that are generally faster to execute.
Another aspect of performance to ponder is the impact on I/O operations. Since UNION requires the database to read the data, sort it, and then write the unique results, it involves a higher number of read and write operations compared to UNION ALL. This can lead to increased disk I/O, which may slow down your overall database performance. For example:
SELECT product_id FROM warehouse_a UNION SELECT product_id FROM warehouse_b;
In this case, the database must perform additional operations to deliver a unique set of product IDs. In contrast, the UNION ALL version:
SELECT product_id FROM warehouse_a UNION ALL SELECT product_id FROM warehouse_b;
requires fewer I/O operations, thus enhancing performance, especially during peak usage times when multiple queries are executed concurrently.