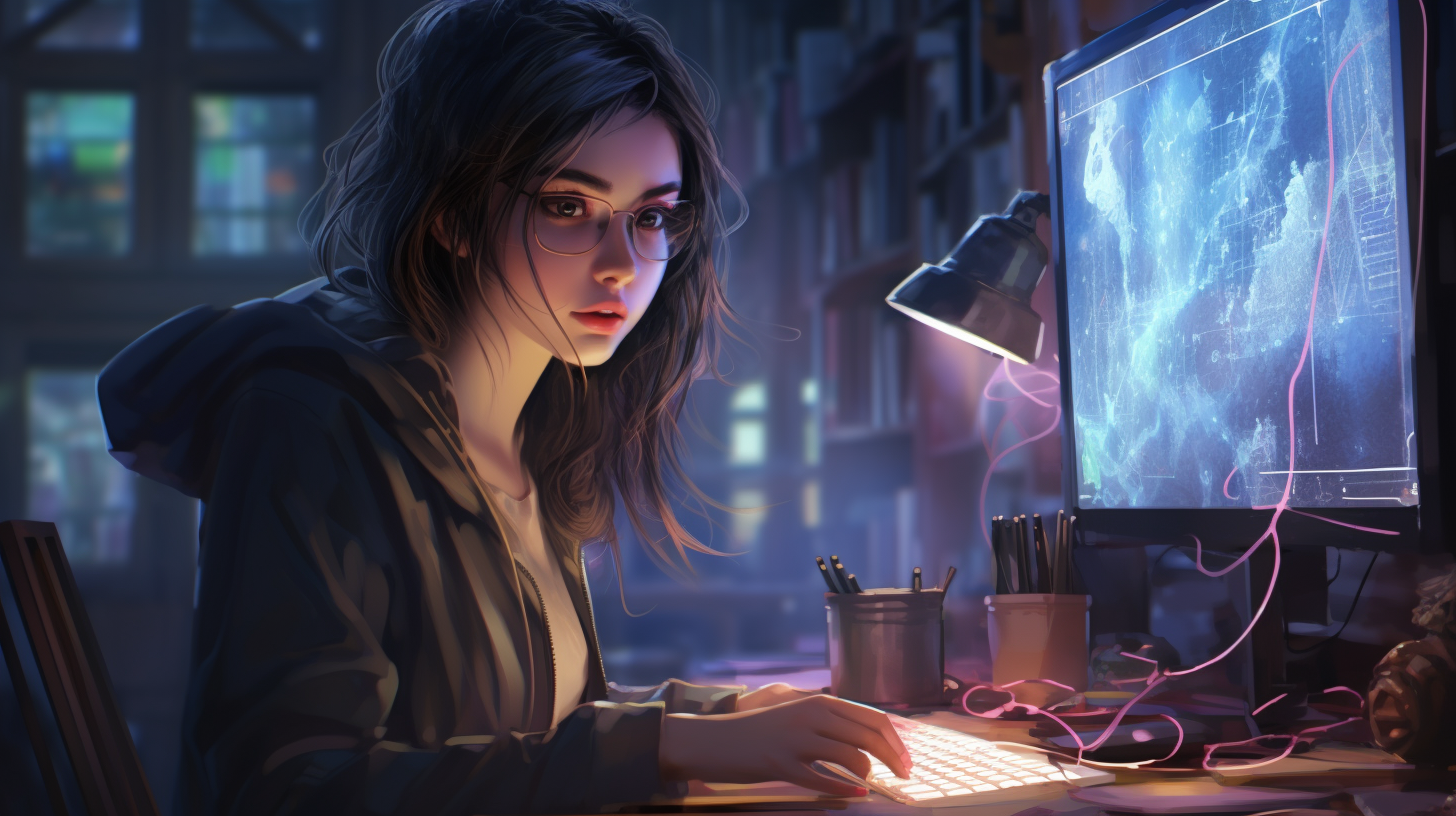
Advanced Bash Redirection
In Bash, redirection is a powerful tool that allows us to control the input and output of commands and scripts. It allows us to manipulate how data flows through the shell and interact with files, streams, and other processes. In this tutorial, we’ll explore advanced redirection techniques in Bash.
Pipes:
Pipes, denoted by the | symbol, are one of the primary tools for redirection in Bash. They allow us to redirect the output of one command as the input to another command.
command1 | command2
For example, let’s say we have a file called data.txt
with the following contents:
apple banana cherry
If we want to sort these lines in alphabetical order, we can use the sort
command in conjunction with a pipe:
cat data.txt | sort
This will send the contents of data.txt
to the standard input of sort
, which will then sort the lines. The output will be:
apple banana cherry
Tees:
The tee command is another useful tool for advanced redirection in Bash. It allows us to split the output of a command or script and send it both to a file and to the standard output.
command | tee output.txt
For example, let’s say we have a script called process.sh
that performs some complex calculations and displays the results. If we want to save both the output and the display of the script, we can use tee:
./process.sh | tee output.txt
This will execute process.sh
and save its output to output.txt
, while still displaying the output in the terminal.
Appending to Files:
In addition to overwriting files, we can also use redirection to append data to an existing file. That is useful when we want to continuously add output to a log file, for example.
command >> file.txt
The command above appends the output of command
to file.txt
. If file.txt
doesn’t exist, it will be created. If it exists, the output will be added to the end of the file without overwriting its existing contents.
Redirecting Standard Error:
By default, standard error (stderr) is not redirected and is displayed in the terminal alongside standard output (stdout). However, we can redirect stderr to a separate file or combine it with stdout using the following syntax:
command 2> error.txt
command > output.txt 2>&1
The first command redirects stderr to error.txt
, while the second command combines both stdout and stderr and redirects them to output.txt
. The 2>&1
notation indicates that stderr should be directed to the same location as stdout.
Null Device:
In some cases, we may want to discard the output of a command completely. We can accomplish this by redirecting the output to the null device. The null device is a special file that discards whatever is written to it.
command > /dev/null
The output of command
will be discarded and not displayed in the terminal or saved to a file.
In this tutorial, we explored advanced redirection techniques in Bash. We learned about pipes, which allow us to redirect the output of one command as the input to another. We also explored tees, which enable us to split the output of a command or script and send it both to a file and to the standard output. Additionally, we covered appending data to files, redirecting standard error, and discarding output using the null device. These techniques give us fine-grained control over how data flows through the shell and greatly enhance our scripting capabilities in Bash.