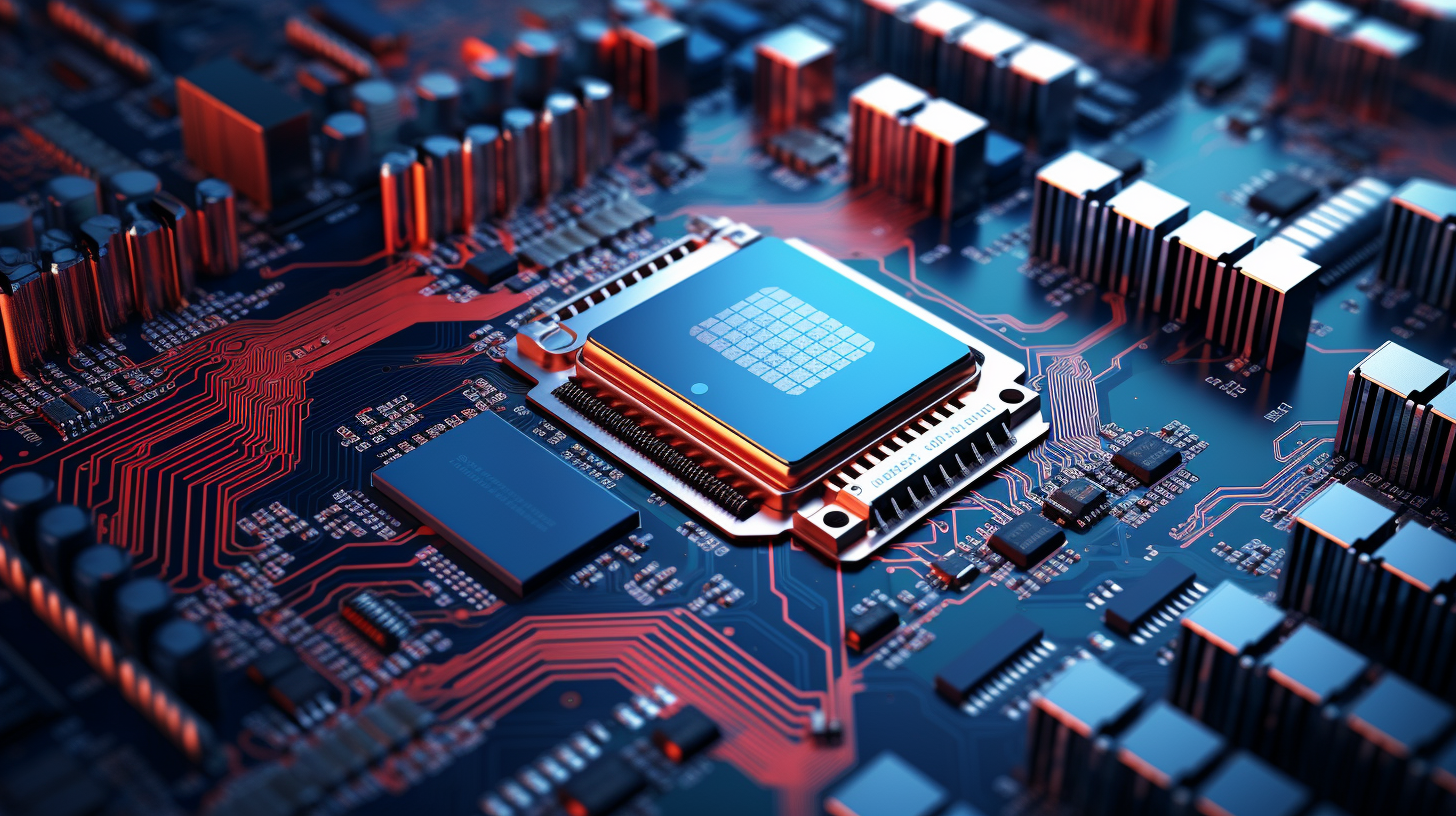
Bash Scripting for E-mail Notifications
Bash scripting is a powerful tool for automating tasks on a Linux system. One common task that you may encounter is the need to send email notifications from your Bash scripts. In this tutorial, we will explore how to accomplish this using the sendmail
command.
The sendmail
command is a standard Unix command-line utility for sending emails. It works by connecting to a mail server and sending the message directly. In order to use sendmail
, you need to have a local mail server configured on your system.
Prerequisites
Before we begin, ensure that you have the following prerequisites:
- A Linux system with Bash installed.
- A local mail server set up and running.
Send a Simple Email
Let’s start by sending a simple email using the sendmail
command. Open your text editor and create a new file called send_email.sh
. Add the following code to the file:
#!/bin/bash TO="[email protected]" SUBJECT="Test Email" BODY="This is a test email." echo -e "Subject:$SUBJECTn$BODY" | sendmail $TO
In this script, we have defined three variables: TO
, SUBJECT
, and BODY
. These variables contain the email recipient, subject, and body respectively. The -e
option is used with echo to enable interpretation of backslash escape sequences, such as the newline character.
The final line uses the echo command to create the email message and pipes it into the sendmail
command. The $TO
variable is used to specify the recipient’s email address. You can change this value to the desired recipient’s email address.
Save the file and make it executable by running the following command:
chmod +x send_email.sh
To execute the script, simply run:
./send_email.sh
If your email server is properly configured, the recipient should receive the email with the specified subject and body.
Send an Email with Attachment
The sendmail
command also allows us to send emails with attachments. Let’s modify our script to send an email with an attachment. Replace the content of send_email.sh
with the following code:
#!/bin/bash TO="[email protected]" SUBJECT="Test Email with Attachment" BODY="Please find the attached file." ATTACHMENT="/path/to/file.txt" echo -e "Subject:$SUBJECTn$BODY" | (cat - && uuencode $ATTACHMENT file.txt) | sendmail $TO
In this modified script, we have added a new variable called ATTACHMENT
, which contains the path to the file you want to attach. Make sure to replace /path/to/file.txt
with the actual path to your file.
The (cat - && uuencode $ATTACHMENT file.txt)
segment is used to concatenate the email message generated by echo with the attachment. We use the cat
command with a hyphen (-
) to read the email message from standard input and combine it with the output of the uuencode
command. The uuencode
command is responsible for encoding the attachment and providing it with a filename (file.txt
in this example).
Save the file and make it executable using the same command as before: chmod +x send_email.sh
. Finally, execute the script to send an email with the attachment:
./send_email.sh
If all goes well, the recipient will receive an email with the specified subject, body, and attached file.
In this tutorial, you have learned how to send email notifications from your Bash scripts using the sendmail
command. By leveraging the power of Bash scripting, you can automate the process of sending emails and streamline your workflow.
Remember, always sanitize any user input before incorporating it into email messages to prevent potential security vulnerabilities.
Explore the various options and capabilities of the sendmail
command to further enhance your email notifications. Happy scripting!