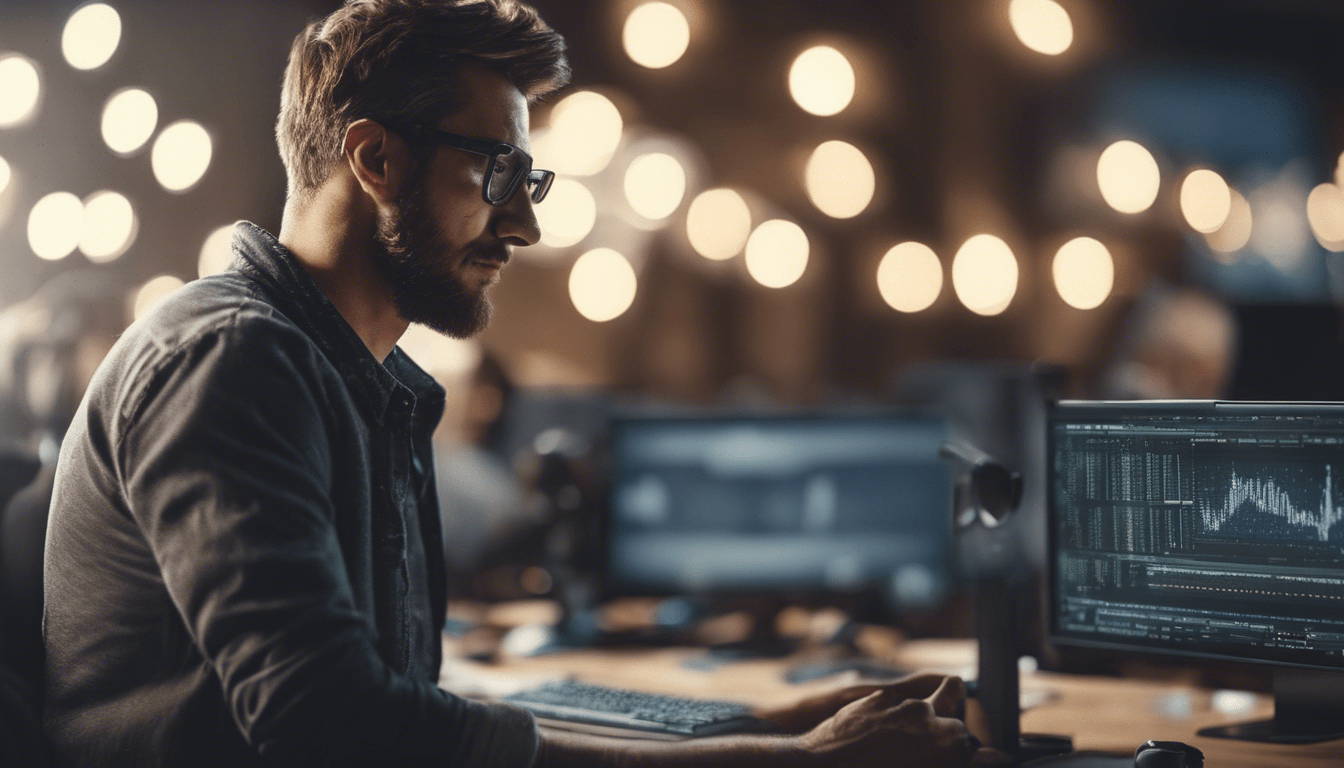
Extensions in Swift
An extension in Swift is a powerful feature that allows you to add new functionality to an existing class, structure, enumeration, or protocol. With extensions, you can write new methods, computed properties, initializers, and even add conformance to protocols for types that you don’t own.
Detailed Explanation of Concepts
Extensions serve as a way to extend the behavior of an existing type without modifying its original source code. That’s particularly useful when you want to provide additional functionality to classes, structs, enums, or protocols that you don’t have direct access to.
Extensions can:
- Add computed properties and convenience initializers to existing types.
- Define new methods for types.
- Make types conform to new protocols.
An extension is defined using the extension
keyword. It follows the syntax:
extension ExistingType { // New functionality goes here }
For example, let’s say you have a class Person
that represents a person with a name. You can extend it to add a computed property to get the person’s initials:
class Person { var firstName: String var lastName: String init(firstName: String, lastName: String) { self.firstName = firstName self.lastName = lastName } } extension Person { var initials: String { return "(firstName.first ?? "")(lastName.first ?? "")" } }
With this extension in place, you can now access the initials property on any instance of the Person
class:
let person = Person(firstName: "John", lastName: "Doe") print(person.initials) // Output: JD
Step-by-Step Guide
Here’s a step-by-step guide on how to implement and use extensions in Swift:
- Create a new Swift file or open an existing one in Xcode.
- Define the type you want to extend (class, struct, enum, or protocol).
- Write the extension block, starting with the
extension
keyword followed by the name of the type. - Inside the extension block, add the new functionality you want to provide.
- Use the extended type as you normally would, now with the added functionality from the extension.
This is it! You have successfully added new functionality to an existing type using extensions in Swift.
Common Pitfalls and Troubleshooting Tips
When working with extensions in Swift, keep the following things in mind to avoid potential pitfalls:
- You cannot override existing functionality or properties with extensions. Extensions only add new functionality.
- Extensions can only add computed properties, not stored properties. This means you cannot add new instance variables using extensions.
- If you want to add new functionality to a class this is declared as
final
, you won’t be able to do so using extensions. In this case, you might ponder subclassing instead.
If you encounter difficulties while working with extensions in Swift, consider the following troubleshooting tips:
- Make sure you are declaring the extension in the correct file and scope. Extensions must be declared in the same file where the type being extended is defined.
- Check if you have any conflicting methods or properties between the original type and the extension. Names of methods or properties cannot be duplicated within the same type.
- If your extension is not being recognized by Xcode, try cleaning and rebuilding your project.
Further Learning Resources
If you want to dive deeper into extensions in Swift, ponder checking out the following resources:
- Swift in Two Hours by Paul Hudson
- iOS 13 & Swift 5 – The Complete iOS App Development Bootcamp on Udemy
- Swift Extensions Tutorial: Syntax and Practice on raywenderlich.com
Extensions are a powerful feature in Swift that allow you to add functionality to existing types without modifying their original source code. They provide a way to keep your code organized, reusable, and easy to read. By using extensions, you can enhance the capabilities of existing types, conform them to new protocols, and increase code maintainability. As you continue your learning journey in Swift, understanding and utilizing extensions will surely make you a more efficient and skilled developer.