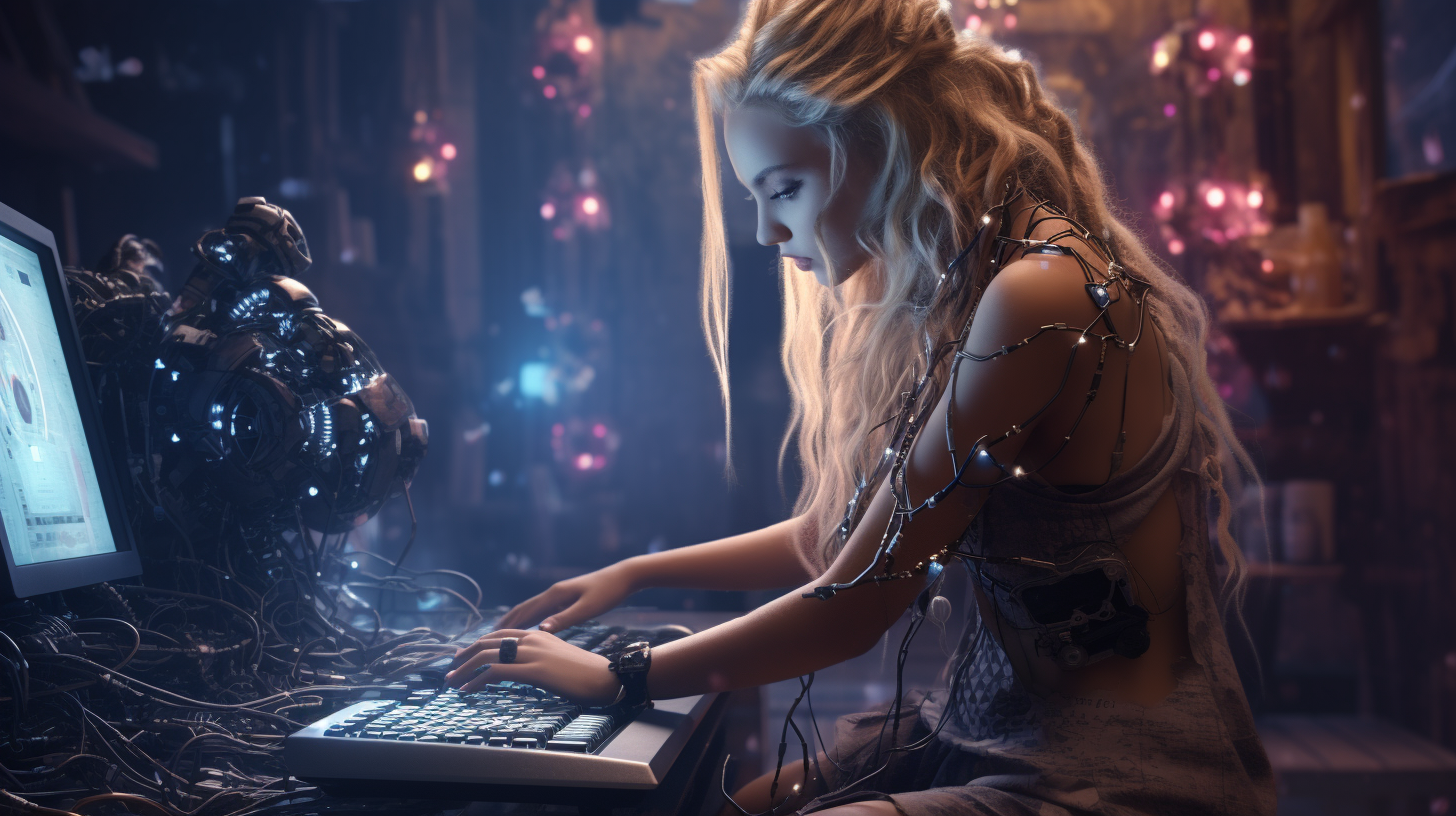
Java and Continuous Deployment: Strategies and Tools
Implementing Continuous Deployment in Java Projects
Continuous Deployment (CD) is a software development practice where code changes are automatically prepared, tested, and deployed to production. Implementing CD in Java projects requires a systematic approach that integrates with the existing development workflow. The following steps outline the process to set up Continuous Deployment for a Java project.
Step 1: Version Control System Integration
Firstly, ensure that your Java project is integrated with a Version Control System (VCS) like Git. This allows for tracking changes and collaboration among team members. A typical setup might look like this:
git init git add . git commit -m "Initial commit"
Step 2: Automated Build Configuration
Next, set up an automated build process using tools like Maven or Gradle. This step ensures that your project can be built with a single command, which is essential for automation:
// For Maven mvn clean install // For Gradle gradle build
Step 3: Continuous Integration Server Setup
Integrate your project with a Continuous Integration (CI) server like Jenkins or Travis CI. These tools monitor your VCS for changes and trigger the build process automatically. Configure the CI server to execute your build command and run tests with each commit:
// Jenkinsfile example pipeline { agent any stages { stage('Build') { steps { sh 'mvn clean install' } } stage('Test') { steps { sh 'mvn test' } } } }
Step 4: Automated Testing
Ensure that your Java project has a comprehensive suite of automated tests. These tests are crucial for CD as they validate the functionality of the code before deployment. Utilize testing frameworks like JUnit or TestNG:
// JUnit example import org.junit.jupiter.api.Test; import static org.junit.jupiter.api.Assertions.assertEquals; public class CalculatorTests { @Test public void testAddition() { Calculator calculator = new Calculator(); assertEquals(5, calculator.add(2, 3)); } }
Step 5: Deployment Automation
Once the build and tests are successful, the next step is to automate the deployment process. This can be done using scripts or deployment tools like Ansible, Chef, or Puppet. Configure these tools to deploy the built artifacts to the production environment:
// Ansible playbook example - hosts: production tasks: - name: Deploy application copy: src: /path/to/artifact.war dest: /path/to/deployment/directory
Step 6: Monitoring and Feedback Loop
Finally, set up monitoring tools to track the application’s performance in production. Tools like New Relic or AppDynamics can provide insights into the application’s health. Establish a feedback loop to quickly address any issues that arise post-deployment:
“The key to successful Continuous Deployment is not just automation, but also the ability to quickly respond to feedback and make necessary adjustments.” – Anonymous DevOps Engineer
Tools for Automating Continuous Deployment in Java
When it comes to automating Continuous Deployment (CD) in Java, there are several tools that can help streamline the process. These tools are designed to work seamlessly with Java projects, ensuring that code changes are automatically built, tested, and deployed to production environments. Below is a list of some of the most popular and effective tools for automating CD in Java:
- An open-source automation server that can be used to automate all sorts of tasks related to building, testing, and deploying software.
- A hosted continuous integration service used to build and test software projects hosted on GitHub and Bitbucket.
- A CI/CD platform that automates the development process using Docker containers or virtual machines.
- A continuous integration and deployment tool that ties automated builds, tests, and releases together in a single workflow.
- A part of GitLab, a web-based DevOps lifecycle tool that provides a CI/CD pipeline for your projects.
- An open-source, multi-cloud continuous delivery platform for releasing software changes with high velocity and confidence.
Each of these tools has its own set of features and advantages. For instance, Jenkins, being open-source, has a large community and a wide array of plugins which makes it highly customizable. Here’s an example of how you can configure a Jenkins pipeline for a Java project:
pipeline { agent any stages { stage('Checkout') { steps { git 'https://github.com/your-repo/java-project.git' } } stage('Build') { steps { sh 'mvn clean install' } } stage('Test') { steps { sh 'mvn test' } } stage('Deploy') { steps { sh 'ansible-playbook -i inventory/production deploy.yml' } } } }
On the other hand, GitLab CI/CD is integrated directly into the GitLab workflow, making it easier for teams already using GitLab to adopt CD practices. Here’s a simple GitLab CI/CD configuration example:
stages: - build - test - deploy build_job: stage: build script: - mvn clean install test_job: stage: test script: - mvn test deploy_job: stage: deploy script: - ansible-playbook -i inventory/production deploy.yml
Best Practices for Successful Continuous Deployment in Java
Continuous Deployment (CD) is an important practice for Java development teams aiming to reduce the time it takes to deliver changes to production. However, simply automating the deployment process is not enough. To ensure successful CD, it is important to adhere to certain best practices:
- Always maintain a clean and deployable master branch. Any code that gets merged into master should have passed all tests and be ready for production.
- From code integration to deployment, every step should be automated to minimize human error and speed up the process.
- Have a robust suite of automated tests (unit, integration, and end-to-end) that run with every build to catch issues early. Here’s an example of an automated unit test in Java using JUnit:
import org.junit.jupiter.api.Test; import static org.junit.jupiter.api.Assertions.*; class StringUtilityTests { @Test void testStringReversal() { assertEquals("dcba", StringUtility.reverse("abcd")); } }
- Manage your infrastructure with code tools like Terraform or AWS CloudFormation to maintain consistency and version control.
- Deploy small changes more frequently to minimize the impact and improve the recovery time in case of issues.
- Use feature toggles to enable or disable features without deploying new code. This allows for easier rollback and canary testing.
- Implement monitoring tools to keep an eye on the application’s performance and set up alerts for any anomalies.
- Have an easy and fast way to rollback to the previous version if something goes wrong during deployment.
- Keep documentation up-to-date and ensure clear communication among team members, especially during deployments.
By following these best practices, Java development teams can maximize the benefits of Continuous Deployment, leading to more efficient and reliable software delivery. Remember, CD is not just about automation; it’s about creating a culture of continuous improvement and collaboration.
It might be worth mentioning the significance of a comprehensive security strategy as part of the Continuous Deployment process. As deployments become more frequent, integrating security measures like automated static code analysis and dynamic application security testing can help catch vulnerabilities early. Additionally, incorporating steps for dependency management to regularly update libraries can further shield Java applications from potential threats. Emphasizing security as part of the CD pipeline could help teams deliver not just quickly, but safely.