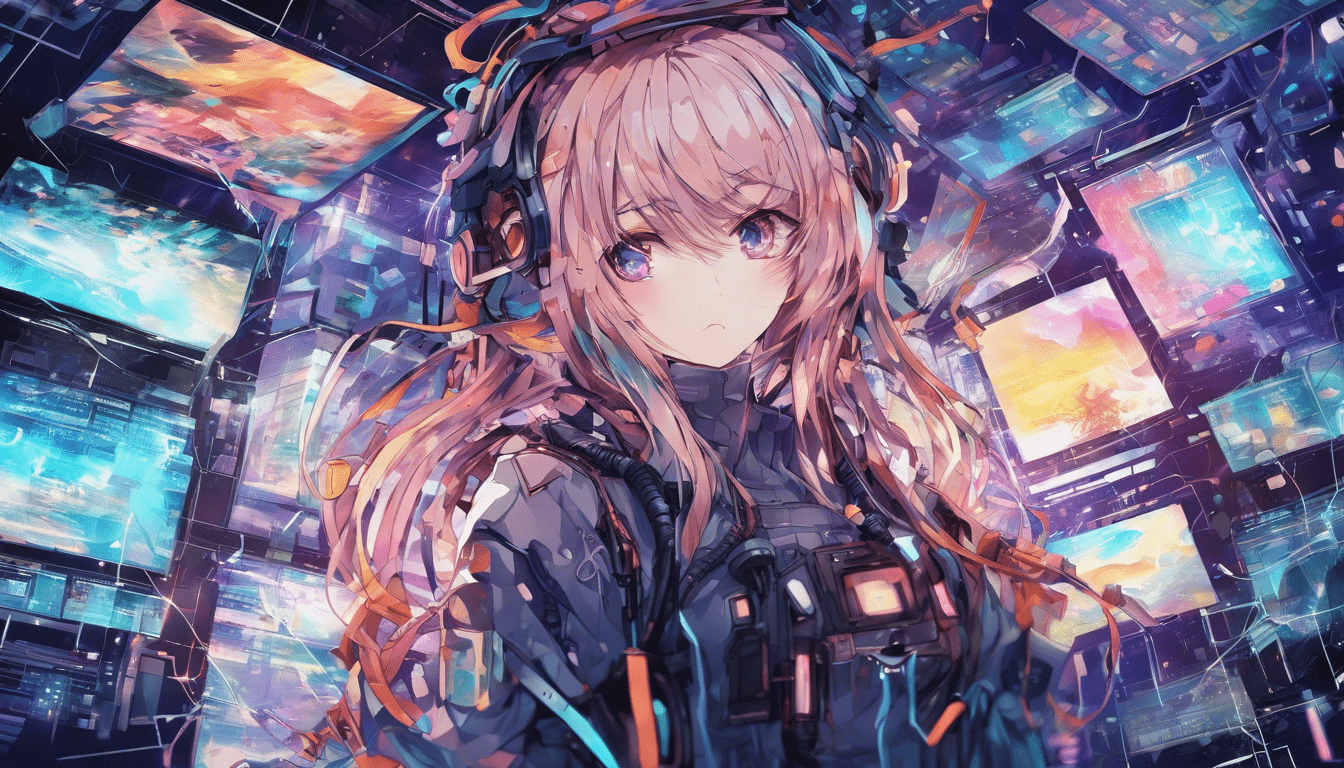
Java and Social Media Integration
In today’s digital age, social media is an integral part of our lives. Many applications and websites integrate social media platforms to provide a more seamless user experience. As a Java developer, you can integrate social media platforms like Facebook and Twitter into your Java applications using their respective APIs. In this article, we will guide you through the process of integrating social media platforms into your Java applications.
Understanding Social Media APIs
API stands for Application Programming Interface, which is a set of rules that allows one software application to interact with another. Social media platforms like Facebook and Twitter provide APIs that developers can use to integrate their features into other applications. For example, you can use the Facebook API to authenticate users, access their profiles, post messages, and more.
Setting Up Your Development Environment
To get started with social media integration in Java, you will need to set up your development environment. You will need to have Java installed on your computer and have access to an integrated development environment (IDE) like IntelliJ IDEA or Eclipse. You will also need to register your application with the social media platform to obtain API keys.
Integrating Facebook API
To integrate the Facebook API into your Java application, you can use the Facebook4J library. This library provides a wrapper around the Facebook API and makes it easier to interact with Facebook from Java code. Here is an example of how to authenticate a user with the Facebook API:
facebook = new FacebookFactory().getInstance(); facebook.setOAuthAppId("YOUR_APP_ID", "YOUR_APP_SECRET"); facebook.setOAuthPermissions("email, public_profile"); facebook.setOAuthAccessToken(new AccessToken("YOUR_ACCESS_TOKEN", null)); User user = facebook.getMe(); System.out.println("User Name: " + user.getName());
Integrating Twitter API