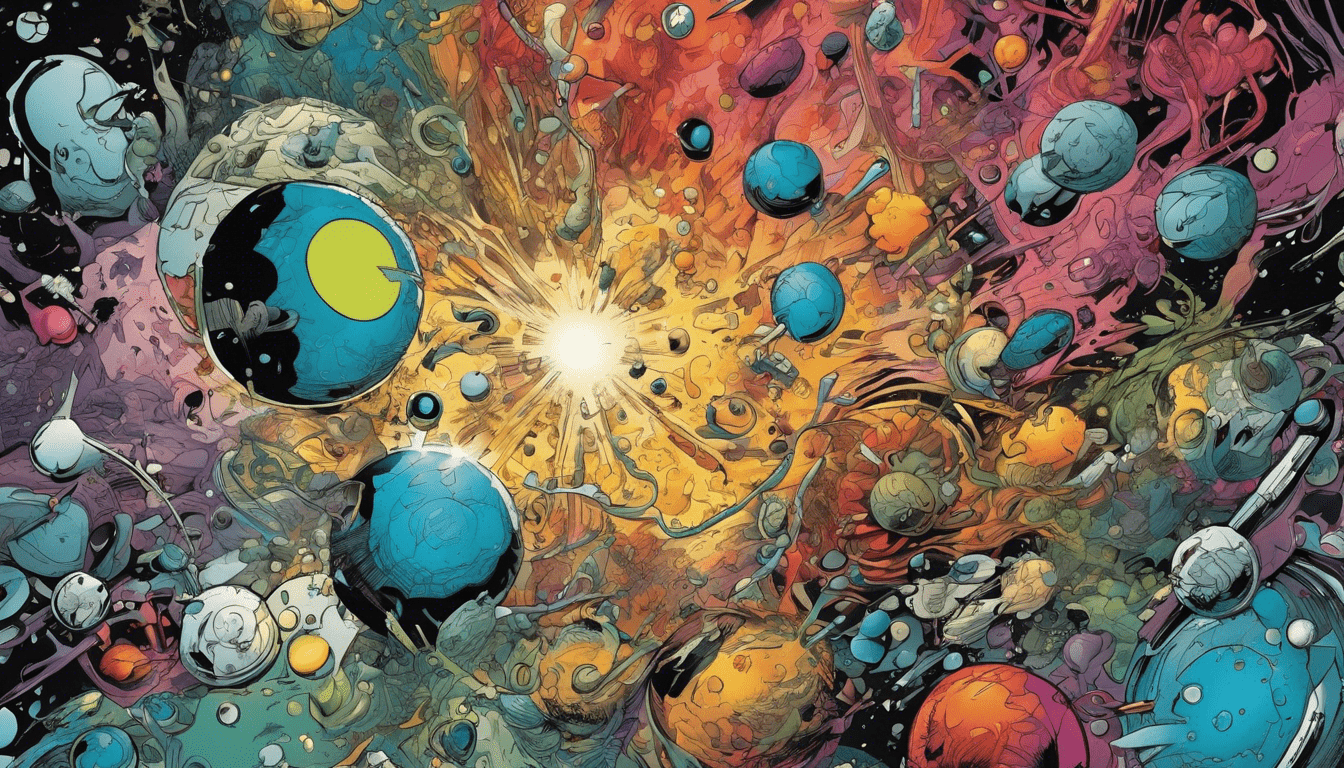
Python and Image Processing: Basics
Python is a versatile programming language that can be used for a wide range of applications, including image processing. Image processing is a technique that allows us to manipulate and analyze digital images. It’s widely used in various fields such as computer vision, medical imaging, and graphic design.
There are several Python libraries available for image processing, but two of the most popular ones are PIL (Python Imaging Library) and OpenCV (Open Source Computer Vision Library). In this article, we will discuss the basics of image processing using these libraries.
PIL
PIL is a library that provides a set of tools for opening, manipulating, and saving different image file formats. It is a straightforward library that’s uncomplicated to manage for beginners.
To use PIL, you first need to install it using pip:
pip install pillow
Once installed, you can start working with images. Here is an example of how to open an image using PIL:
from PIL import Image # Open an image image = Image.open('example.jpg') # Display the image image.show()
PIL also enables you to perform various operations on images, such as rotating, resizing, and cropping. Here is an example of how to rotate an image:
# Rotate the image 90 degrees rotated_image = image.rotate(90) # Save the rotated image rotated_image.save('rotated_image.jpg')
OpenCV
OpenCV is another popular library for image processing this is more advanced than PIL. It provides a wide range of tools for image and video analysis, including object detection, face recognition, and motion tracking.
To use OpenCV, you first need to install it using pip:
pip install opencv-python
Once installed, you can start working with images. Here is an example of how to read and display an image using OpenCV:
import cv2 # Read an image image = cv2.imread('example.jpg') # Display the image cv2.imshow('Image', image) cv2.waitKey(0) cv2.destroyAllWindows()
OpenCV also enables you to perform various operations on images, such as converting them to grayscale, blurring, and edge detection. Here is an example of how to convert an image to grayscale:
# Convert the image to grayscale gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY) # Save the grayscale image cv2.imwrite('gray_image.jpg', gray_image)
In conclusion, Python offers powerful libraries like PIL and OpenCV that make image processing easy and accessible for beginners. With just a few lines of code, you can perform complex operations on images and create amazing results.
It’s great to see an article highlighting the capabilities of Python for image processing. I’d like to add that in addition to PIL and OpenCV, there are other libraries such as scikit-image and imageio that are also commonly used in this field. Scikit-image, for example, is built on top of SciPy and is designed for more complex image processing tasks, while imageio is focused on reading and writing a wide range of image data. It’s worth exploring these libraries as well depending on the specific needs of your project.