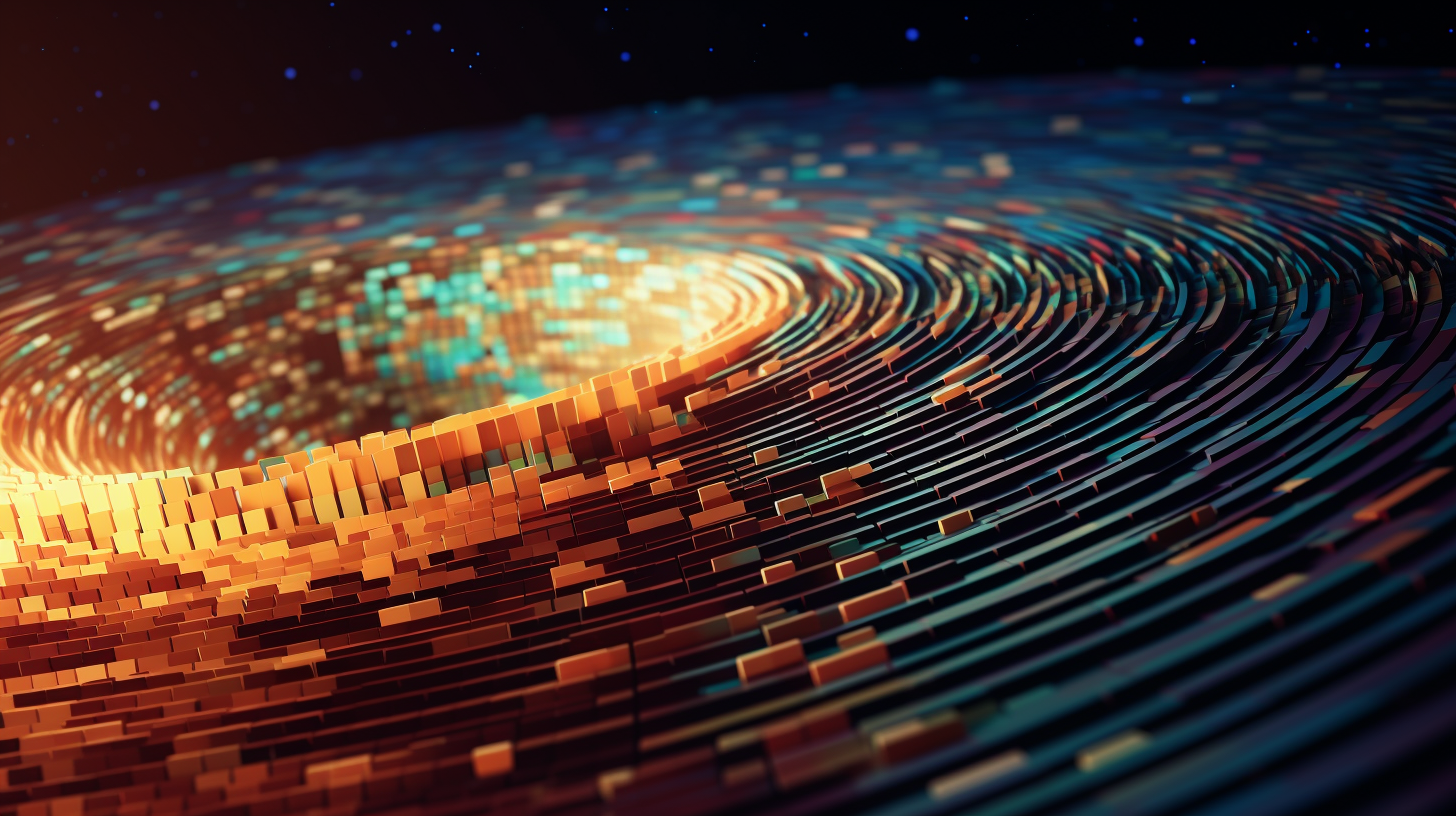
JavaScript and Geolocation
Understanding Geolocation in JavaScript
Geolocation is a powerful feature in contemporary web browsers that allows developers to retrieve the geographical location of a user. This capability can be used in a variety of applications, such as mapping, location-based services, and more. In JavaScript, the geolocation functionality is accessed through the navigator.geolocation
object.
The navigator.geolocation
object provides access to the location of the device. It contains three main methods:
- getCurrentPosition() – Retrieves the current position of the device.
- watchPosition() – Returns the current position of the device when it changes.
- clearWatch() – Stops the
watchPosition()
method from continuing to return the device’s updated position.
To use the geolocation API, you need to call the getCurrentPosition()
method, which is an asynchronous operation. This method takes three parameters: a success callback, an error callback, and an optional options object.
navigator.geolocation.getCurrentPosition(success, error, options); function success(position) { var latitude = position.coords.latitude; var longitude = position.coords.longitude; // Do something with the retrieved coordinates. } function error(err) { console.warn('ERROR(' + err.code + '): ' + err.message); } var options = { enableHighAccuracy: true, timeout: 5000, maximumAge: 0 };
The success callback is invoked when the location is successfully retrieved, and it receives a position
object as its argument. This object contains various properties, including coords
which is an object that holds the latitude and longitude among other things.
If the operation fails, the error callback is called with an error
object that contains two properties: code
and message
. The code property will be one of the following:
- 1 – PERMISSION_DENIED
- 2 – POSITION_UNAVAILABLE
- 3 – TIMEOUT
The options object allows you to control the behavior of the getCurrentPosition()
method. The enableHighAccuracy
property, for example, indicates whether to improve the accuracy of the position at the cost of longer response times and increased power consumption.
By understanding these basics of the geolocation API in JavaScript, developers can start to integrate location-based functionality into their web applications, improving the user experience by providing context-aware content and services.
Implementing Geolocation in Web Applications
Implementing geolocation in web applications involves a few key steps. First, you must ensure that the user’s browser supports geolocation. You can do this by checking if the navigator.geolocation
object is available. If it is, you can then proceed to use the geolocation methods to retrieve the user’s location.
if ("geolocation" in navigator) { // Geolocation is available } else { // Geolocation is not supported by this browser }
Once you have confirmed that geolocation is supported, you can call the getCurrentPosition()
method to get the user’s current location. This method is asynchronous and takes a success callback, an error callback, and an optional options object, as discussed earlier.
navigator.geolocation.getCurrentPosition(success, error, options);
In the success callback, you can use the latitude and longitude to display the user’s location on a map or use it for other location-based services. Here’s an example of how to use the success callback to display the user’s coordinates:
function success(position) { var latitude = position.coords.latitude; var longitude = position.coords.longitude; console.log('Latitude is ' + latitude + '°'); console.log('Longitude is ' + longitude + '°'); }
It’s also important to handle errors gracefully. If the geolocation request fails, the error callback will be executed. You can use this callback to inform the user of the issue and provide alternative instructions.
function error(err) { console.warn('ERROR(' + err.code + '): ' + err.message); // Handle errors here }
For a more interactive experience, you might want to continuously track the user’s position as it changes. This can be achieved using the watchPosition()
method. This method is similar to getCurrentPosition()
, but it returns a watch ID that can be used to stop the watch operation using the clearWatch()
method.
var watchID = navigator.geolocation.watchPosition(success, error, options); // To stop watching the user's position navigator.geolocation.clearWatch(watchID);
By implementing geolocation in your web applications, you can create a more dynamic and personalized user experience. Whether it’s for providing location-based recommendations, tracking movement, or simply displaying a map, geolocation is a powerful tool that can enhance the functionality of your web applications.
Handling Geolocation Errors
When implementing geolocation in your web application, it very important to handle errors effectively to ensure a smooth user experience. Errors can occur for various reasons, such as the user denying permission to access their location, the device’s location being unavailable, or a timeout occurring while attempting to retrieve the location.
The error callback function is designed to handle these situations. When an error occurs, the geolocation API passes an error object to this callback. This object includes two properties: code
and message
. The code
property will be a numerical value corresponding to one of the following error types:
- The user has denied the request for geolocation.
- The device’s location information is unavailable.
- The attempt to obtain the device’s location has timed out.
Here’s an example of a detailed error callback function that handles each type of error:
function error(err) { switch(err.code) { case err.PERMISSION_DENIED: console.error("User denied the request for Geolocation."); break; case err.POSITION_UNAVAILABLE: console.error("Location information is unavailable."); break; case err.TIMEOUT: console.error("The request to get user location timed out."); break; default: console.error("An unknown error occurred."); break; } }
It’s good practice to inform the user of the error and provide guidance on how to resolve it. For example, if the error is PERMISSION_DENIED
, you might suggest that the user check their browser’s location settings. If the error is POSITION_UNAVAILABLE
, you could suggest the user move to an area with better reception. In the case of a TIMEOUT
, you could ask the user to try the request again.
Note: Always respect the user’s privacy and preferences when using geolocation. If the user denies permission, do not attempt to circumvent their choice by using other means to determine their location.
By properly handling geolocation errors, you can provide a better experience for your users and avoid unnecessary frustrations that could arise from unhandled errors.
Best Practices for Using Geolocation in JavaScript
When using geolocation in JavaScript, it’s important to follow best practices to ensure a positive user experience and the privacy of your users. Here are some of the best practices you should consider:
- Always request the user’s permission to access their location in a way that makes it clear why you need it. Avoid asking for location access immediately upon page load; instead, wait until it is contextually relevant.
- If a user denies permission to access their location, respect their choice and provide alternative ways for them to use your application without geolocation.
- The
options
object in the geolocation API allows you to specify preferences for the location request. UseenableHighAccuracy
only when necessary, as it can lead to longer response times and higher power consumption. - When accessing a user’s location, provide visual feedback such as a loader or a message to inform them that their location is being retrieved. This helps manage user expectations and reduces the perception of unresponsiveness.
- To avoid unnecessary location requests, ponder caching the location data for a certain period using the
maximumAge
option. This can improve performance and reduce power usage. - Use HTTPS to ensure that location data is transmitted securely over the network and to prevent man-in-the-middle attacks.
- Test your geolocation implementation under various conditions, including when the location is unavailable or when the user is on the move. This will help you ensure that your application behaves as expected in real-world scenarios.
Here’s an example of how to implement these best practices in your code:
function getLocation() { if ("geolocation" in navigator) { // Provide a button or other UI element to request location access document.getElementById('get-location-btn').addEventListener('click', function() { navigator.geolocation.getCurrentPosition(success, error, { enableHighAccuracy: true, timeout: 10000, maximumAge: 60000 }); }); } else { console.log('Geolocation is not supported by this browser.'); } } function success(position) { // Provide feedback to the user console.log('Location retrieved: ', position.coords); // Use the location data } function error(err) { // Handle errors and inform the user console.warn('ERROR(' + err.code + '): ' + err.message); } // Call the getLocation function when it is contextually relevant // getLocation();
By following these best practices, you can create a more trustworthy and easy to use geolocation experience in your JavaScript applications.