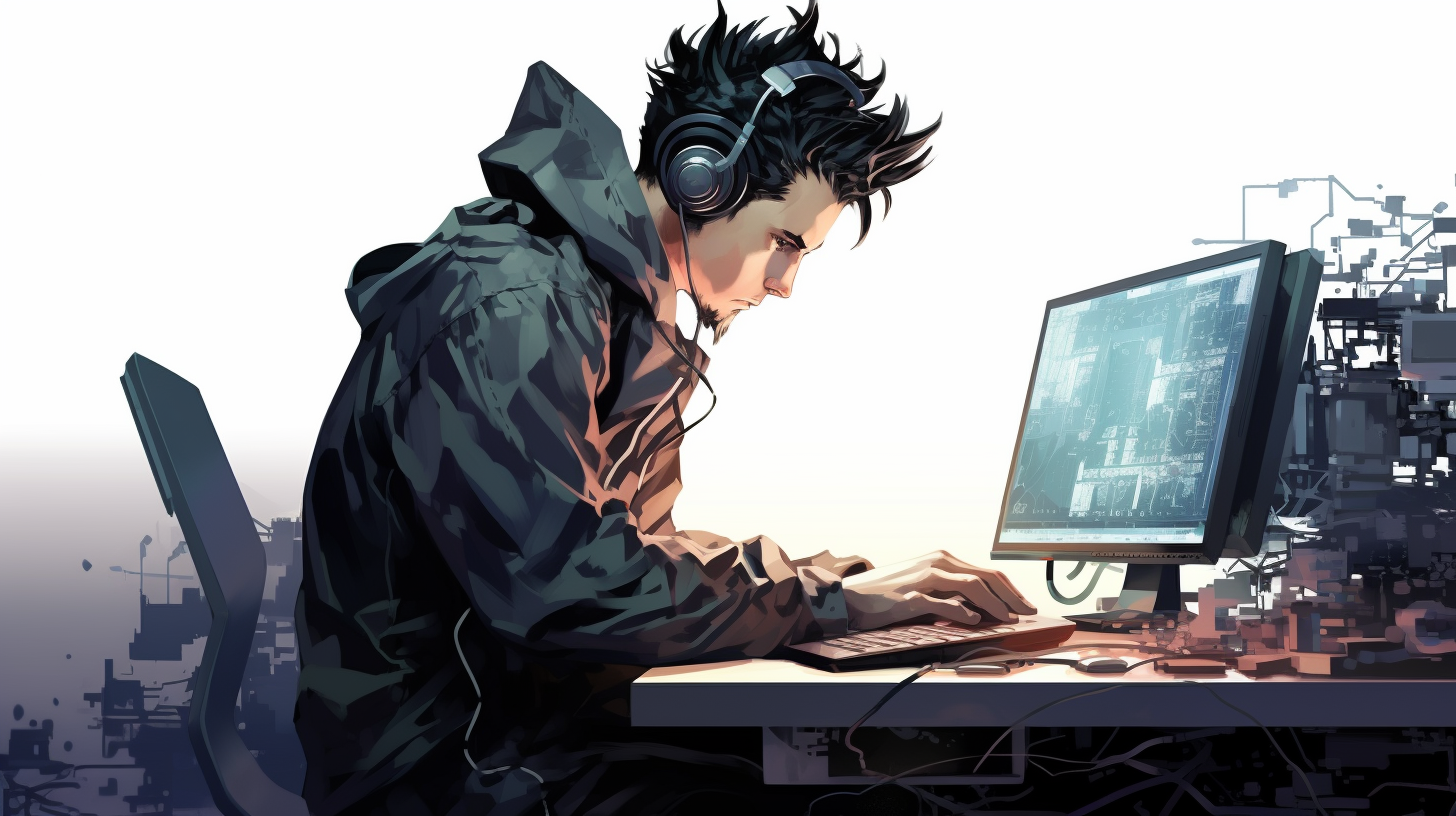
PHP and Payment Gateways: Integrating Transactions
When it comes to online transactions, integrating a payment gateway is a critical step in ensuring a smooth and secure checkout process for customers. PHP, being a popular server-side scripting language, provides various options for integrating payment gateways into your website. Two of the most commonly used payment gateways are Stripe and PayPal. In this tutorial, we will take a look at how to integrate these payment gateways using PHP.
Stripe Integration
To integrate Stripe with PHP, you will need to have a Stripe account and obtain your API keys from the Stripe dashboard. Once you have your API keys, you can use the Stripe PHP library to start processing payments.
First, you need to install the Stripe PHP library. You can use Composer to do so by running the following command:
composer require stripe/stripe-php
Next, include the Stripe PHP library in your script and set your API key:
<?php require_once('vendor/autoload.php'); StripeStripe::setApiKey('YOUR_SECRET_KEY'); ?>
You can now create a charge by providing the necessary details like amount, currency, and payment method details. Here’s an example of how to create a charge:
<?php // Token is created using Checkout or Elements! // Get the payment token ID submitted by the form: $token = $_POST['stripeToken']; $charge = StripeCharge::create([ 'amount' => 999, 'currency' => 'usd', 'description' => 'Example charge', 'source' => $token, ]); ?>
In the above example, $_POST[‘stripeToken’] is the token obtained from the frontend after the user enters their payment details.
PayPal Integration
To integrate PayPal with PHP, you need to set up a PayPal Developer account and create an app to obtain your API credentials.
Once you have your credentials, you can use the PayPal REST API SDK to make API calls. First, install the SDK using Composer:
composer require paypal/rest-api-sdk-php
Now, you can create an instance of the PayPal APIContext and provide your client ID and secret:
<?php require __DIR__ . '/vendor/autoload.php'; use PayPalRestApiContext; use PayPalAuthOAuthTokenCredential; $apiContext = new ApiContext( new OAuthTokenCredential( 'YOUR_CLIENT_ID', // ClientID 'YOUR_CLIENT_SECRET' // ClientSecret ) ); // OR if you want to configure per request: $apiContext->setConfig([...]); ?>
With the ApiContext configured, you can now create a payment using PayPal SDK classes:
<?php use PayPalApiAmount; use PayPalApiPayer; use PayPalApiPayment; use PayPalApiRedirectUrls; use PayPalApiTransaction; $payer = new Payer(); $payer->setPaymentMethod('paypal'); $amount = new Amount(); $amount->setCurrency('USD') ->setTotal('10.00'); $transaction = new Transaction(); $transaction->setAmount($amount) ->setDescription('Payment description'); $redirectUrls = new RedirectUrls(); $redirectUrls->setReturnUrl('http://yourwebsite.com/success') ->setCancelUrl('http://yourwebsite.com/cancel'); $payment = new Payment(); $payment->setIntent('sale') ->setPayer($payer) ->setTransactions([$transaction]) ->setRedirectUrls($redirectUrls); try { $payment->create($apiContext); } catch (Exception $ex) { // handle exception } $approvalUrl = $payment->getApprovalLink(); ?>
In this code snippet, we set up a Payer with PayPal as the payment method, provide the transaction Amount, define succes and cancel URLs with RedirectUrls, and finally create a Payment with intent as ‘sale’. If successful, getApprovalLink() returns a URL where the user needs to be redirected to complete the payment on PayPal’s site.
To wrap it up, integrating payment gateways with PHP requires setting up credentials on the respective gateway’s platform, including necessary libraries or SDKs in your PHP code, and creating an instance to handle transactions. Both Stripe and PayPal provide detailed documentation and support to assist developers in integrating their services effectively.