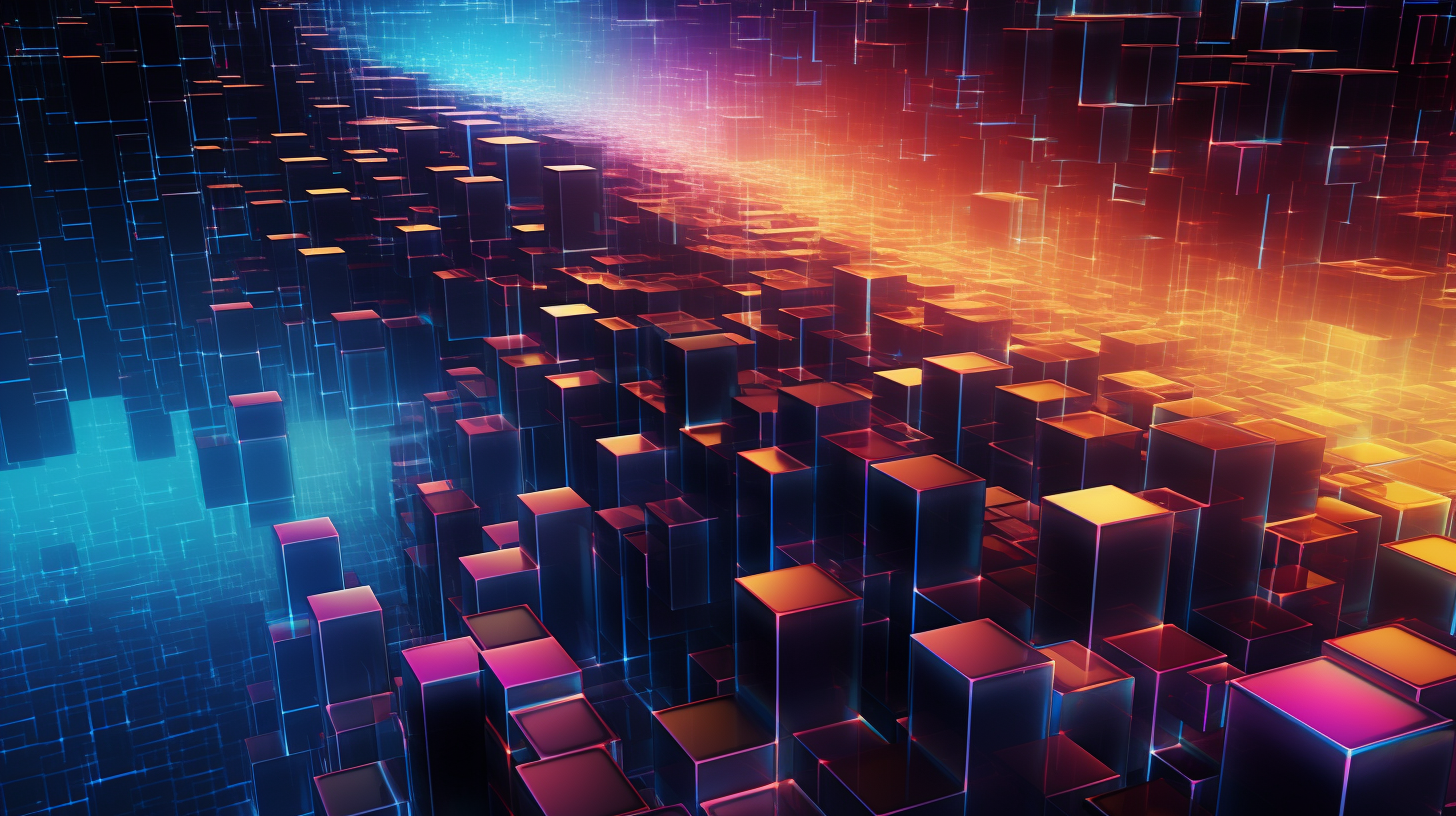
PHP and Security: Protecting Web Applications
Common Security Risks in PHP Web Applications
When developing PHP web applications, it’s crucial to be aware of the common security risks that can lead to vulnerabilities. Some of the most prevalent issues include:
- This occurs when an attacker is able to insert or manipulate SQL queries by inputting malicious code into a form or URL parameter. To prevent this, always use prepared statements with parameterized queries. For example:
$stmt = $pdo->prepare('SELECT * FROM users WHERE username = :username AND password = :password'); $stmt->execute(['username' => $username, 'password' => $password]);
- XSS attacks happen when an attacker injects malicious scripts into webpages viewed by other users. To mitigate this risk, always escape output and use functions like
htmlspecialchars()
orhtmlentities()
. For instance:
echo htmlspecialchars($user_input, ENT_QUOTES, 'UTF-8');
- CSRF attacks trick a user into performing actions they didn’t intend to, often by exploiting authenticated sessions. Use anti-CSRF tokens and validate them on the server-side to protect against this. A simple example:
if ($_POST['csrf_token'] !== $_SESSION['csrf_token']) { // Handle the error }
- Attackers might steal or predict a user’s session ID to impersonate them. Regenerate session IDs and use secure, HTTP-only cookies. For example:
session_regenerate_id(true); setcookie(session_name(), session_id(), null, '/', null, null, true);
- If your application allows file uploads, ensure strict validation on file types and sizes. Additionally, store files in a non-executable directory or use secure handlers like:
if (in_array($file_extension, $allowed_extensions)) { move_uploaded_file($_FILES['file']['tmp_name'], $destination); }
- Prevent attackers from executing arbitrary code by validating all user inputs and avoiding functions that can execute system commands unless absolutely necessary. If you must use them, carefully sanitize the inputs like so:
$clean_input = escapeshellcmd($user_input); system($clean_input);
By understanding these common risks and how to mitigate them, developers can significantly improve the security of their PHP web applications.
Best Practices for Secure PHP Development
When it comes to secure PHP development, adhering to best practices is essential. The following guidelines will help you strengthen the security of your web applications:
- Keep PHP and its components up to date: Regularly update your PHP version and all related libraries and frameworks to ensure you have the latest security patches.
- Use secure communication: Implement SSL/TLS to encrypt data transmitted between the client and server. This helps protect sensitive information from being intercepted by attackers.
- Limit error reporting: While detailed error messages are valuable for debugging, they should not be displayed to users, as they can reveal potential vulnerabilities. Instead, log errors to a secure file and show generic error messages to users.
- Enforce strong passwords: Encourage or enforce the use of strong, complex passwords for user accounts to reduce the risk of brute force attacks.
-
Use secure session management: Store session data securely and avoid exposing session IDs in URLs. Regenerate session IDs after login to prevent session fixation.
session_start(); if (!isset($_SESSION['initiated'])) { session_regenerate_id(); $_SESSION['initiated'] = true; }
- Implement access controls: Ensure that users can only access the resources they’re authorized to. Check user permissions before executing sensitive operations.
- Conduct security audits: Regularly review your code and use automated tools to check for vulnerabilities. Consider hiring external experts to perform penetration testing.
By following these best practices, you will create a more secure environment for your PHP web applications, reducing the risk of attacks and data breaches.
Implementing Input Validation and Sanitization
Input validation and sanitization are critical components of web application security. They ensure that the data your application receives is what it expects and is safe to use. Without proper validation and sanitization, your application could be vulnerable to various attacks such as SQL injection, XSS, and more.
Input Validation is the process of ensuring that a program operates on clean, correct and useful data. It involves checking if the input meets certain criteria before processing it. For example:
if (isset($_POST['age']) && is_numeric($_POST['age'])) { $age = $_POST['age']; } else { // Handle the error }
However, validation alone isn’t enough. You also need to sanitize the input to ensure it’s safe to use in your application. Sanitization is the process of removing or encoding unsafe characters from the input. For instance, when dealing with HTML content, you can use the htmlspecialchars()
function:
$user_input = htmlspecialchars($_POST['comment'], ENT_QUOTES, 'UTF-8');
It is also important to validate and sanitize file uploads. You should check the file size, type, and even content before allowing it to be uploaded to your server:
if ($_FILES['userfile']['size'] > 400000) { die('File is too large.'); } $allowed_types = array('image/jpeg', 'image/png', 'image/gif'); if (!in_array($_FILES['userfile']['type'], $allowed_types)) { die('Invalid file type.'); }
For more complex or custom validation rules, consider using a validation library or framework component that provides a wide range of validation functions and methods for different types of data.
Always remember that
“All input is evil until proven otherwise.”
By rigorously validating and sanitizing user input, you can protect your PHP web applications from a high number of potential threats.
Using Secure Authentication and Authorization Methods
Secure authentication and authorization are the cornerstones of protecting web applications from unauthorized access. In PHP, there are several methods and practices you can implement to ensure that only legitimate users can access your application’s resources.
Use Strong Hashing Algorithms
Passwords should never be stored in plain text. Always hash passwords using strong algorithms like bcrypt, which is available in PHP through the password_hash()
and password_verify()
functions. Here’s an example of how to hash a password and then verify it:
$hashed_password = password_hash($password, PASSWORD_BCRYPT); if (password_verify($input_password, $hashed_password)) { // The password is correct }
Implement Two-Factor Authentication (2FA)
Two-factor authentication adds an extra layer of security by requiring users to provide a second form of identification, often a one-time code sent to their phone or email. PHP developers can integrate 2FA using third-party services or libraries.
Use Secure Cookie Flags
When dealing with sessions and cookies, it is important to set secure flags. Use the httponly
flag to prevent access to the cookie via JavaScript, and the secure
flag to ensure the cookie is only sent over HTTPS.
setcookie('PHPSESSID', session_id(), 0, '/', '', isset($_SERVER["HTTPS"]), true);
Limit Login Attempts
To protect against brute force attacks, limit the number of login attempts a user can make. If the limit is reached, lock the account for a period of time or until the user can verify their identity.
if ($_SESSION['login_attempts'] > 3) { die('Account locked due to too many login attempts. Please try again later.'); }
Role-Based Access Control (RBAC)
Implementing RBAC ensures that users can only perform actions that their role allows. Define roles and permissions clearly, and check them before executing sensitive operations.
if ($user_role !== 'admin') { die('You do not have permission to access this resource.'); }
By using these secure authentication and authorization methods, you can significantly reduce the risk of unauthorized access and protect your PHP web applications from potential threats.
Regular Maintenance and Updates for PHP Security
Regular maintenance and updates are crucial for maintaining the security of your PHP web applications. Neglecting this aspect can leave your applications vulnerable to new threats and exploits. Here are some key practices to ensure your PHP security is up-to-date:
- Regularly check for and install updates for PHP and any third-party libraries or frameworks you’re using. These updates often contain security patches that address known vulnerabilities. For example:
// Updating PHP via the command line sudo apt-get update sudo apt-get upgrade php
- As PHP evolves, certain functions may become deprecated and eventually removed. Using outdated functions can lead to security risks, so it is important to review and update your code accordingly. For example:
Using
mysql_*
functions is no longer recommended. Instead, switch tomysqli_*
orPDO
for database interactions.
- Keep an eye on security advisories for PHP and related technologies. This can be done by subscribing to mailing lists, following relevant blogs, or using security plugins that notify you of vulnerabilities.
- Establish a routine for conducting security audits. This can help identify and rectify any security issues before they can be exploited. Automated tools can aid in this process, but manual code review by advanced developers is also beneficial.
// Example of a security audit tool command phpcs --standard=Security /path/to/your/php/files
- Regular backups can be a lifesaver in case of a security breach. Ensure that you have automated backups in place and that they are stored securely.
It is not enough to just have backups; you must also test them to ensure they can be restored successfully.
- Using a version control system like Git can help track changes, manage updates, and revert to previous versions if an update causes issues.
// Reverting to a previous commit in Git git checkout [commit_id]
By adhering to these maintenance and update practices, you’ll ensure that your PHP applications remain secure against the ever-evolving landscape of web security threats. Remember, security is not a one-time task but an ongoing process.