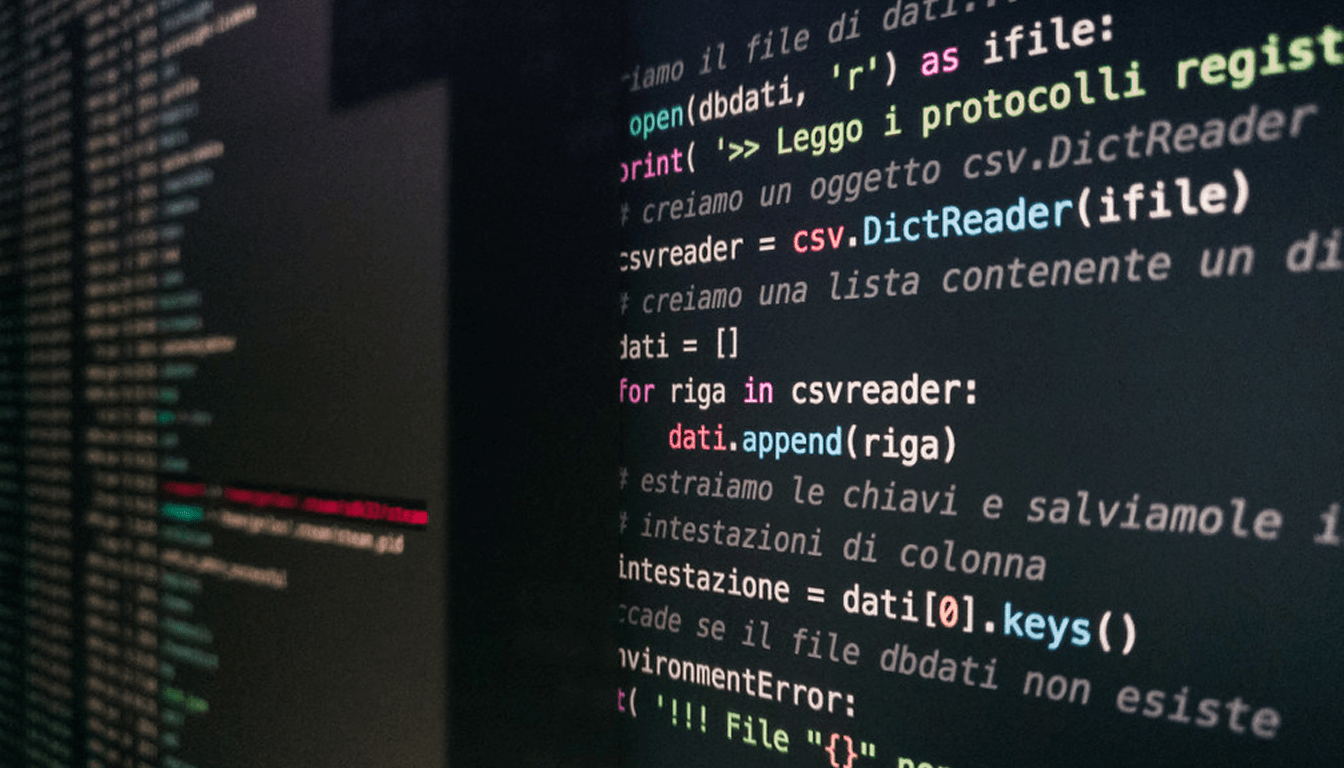
Python and Construction: Project Management and Analysis
With its simplicity, readability, and powerful libraries, Python can streamline project management and enable data analysis in the construction field. This article will introduce you to the concepts of project management and analysis in construction, explain their relevance to Python programming, and provide a step-by-step guide for beginners to implement these concepts using Python.
Detailed Explanation of Concepts
1. Project Management: Project management involves planning, organizing, and controlling resources and tasks to achieve specific project goals within constraints such as time, budget, and quality. In construction, project management is important for successful execution and delivery. Python can facilitate project management by automating repetitive tasks, tracking progress, and generating reports.
# Example: Automating project task allocation import pandas as pd def allocate_tasks(employees, tasks): employee_ids = range(len(employees)) task_ids = range(len(tasks)) allocations = [] for task_id, employee_id in zip(task_ids, employee_ids): allocations.append({ 'Task': tasks[task_id], 'Employee': employees[employee_id] }) df_allocations = pd.DataFrame(allocations) return df_allocations employees = ['John', 'Jane', 'Mike'] tasks = ['Excavation', 'Foundation', 'Framing', 'Roofing'] allocated_tasks = allocate_tasks(employees, tasks) print(allocated_tasks)
2. Data Analysis: Data analysis in construction involves collecting, analyzing, and interpreting project-related data to gain insights and improve decision-making. Python provides libraries like Pandas and NumPy for data processing, Matplotlib and Seaborn for data visualization, and Scikit-learn for predictive modeling. These libraries enable data-driven decision-making and help identify potential risks and opportunities.
# Example: Analyzing project cost overruns import pandas as pd import matplotlib.pyplot as plt def analyze_cost_overruns(costs): df_costs = pd.DataFrame(costs, columns=['Month', 'Actual Cost', 'Planned Cost']) df_costs['Cost Overrun'] = df_costs['Actual Cost'] - df_costs['Planned Cost'] plt.plot(df_costs['Month'], df_costs['Cost Overrun'], marker='o') plt.xlabel('Month') plt.ylabel('Cost Overrun') plt.title('Project Cost Overruns') plt.show() costs = [ ['January', 10000, 8000], ['February', 12000, 9000], ['March', 15000, 10000] ] analyze_cost_overruns(costs)
Step-by-Step Guide
Follow these steps to implement project management and analysis concepts using Python:
- Install Python and necessary libraries such as Pandas, NumPy, Matplotlib, and Scikit-learn.
- Import the required libraries into your Python script.
- Define functions for automating project management tasks or analyzing project data.
- Use Python data structures like lists or dictionaries to store and manipulate project-related information.
- Utilize built-in Python functions or library functions for data processing, visualization, and modeling.
- Execute your Python script and observe the results.
Common Pitfalls and Troubleshooting Tips
As a beginner working with Python in construction project management and analysis, you may encounter the following common pitfalls:
- Ensure that you have a clear understanding of the project requirements before implementing any project management or analysis code.
- Garbage in, garbage out. Ensure that your project data is accurate, complete, and consistent for reliable analysis.
- Make sure to read the documentation of each library you use and understand how to properly utilize its functions and methods.
- To avoid unexpected errors, implement robust error handling techniques such as try-except blocks and input validation.
Python is a valuable tool for project management and data analysis in the construction industry. By leveraging Python’s simplicity and powerful libraries, you can automate tasks, track project progress, analyze data, and make informed decisions. Understanding these concepts and continuously learning and practicing Python will enhance your skills and make you a more efficient professional in the construction field. So, embrace Python programming and unlock new possibilities for project management and analysis!
Great article! One thing I would like to add is the importance of considering the scalability of Python scripts for larger and more complex construction projects. As projects grow in size, the volume of data and the complexity of project management tasks also increase. It would be beneficial to discuss how to structure Python code and utilize libraries like Dask for distributed computing to handle larger datasets and parallel processing for efficiency. Additionally, incorporating techniques for data validation and cleaning can ensure the integrity of the analysis results. Overall, considering scalability and data quality can help maximize the benefits of using Python in construction project management and analysis.