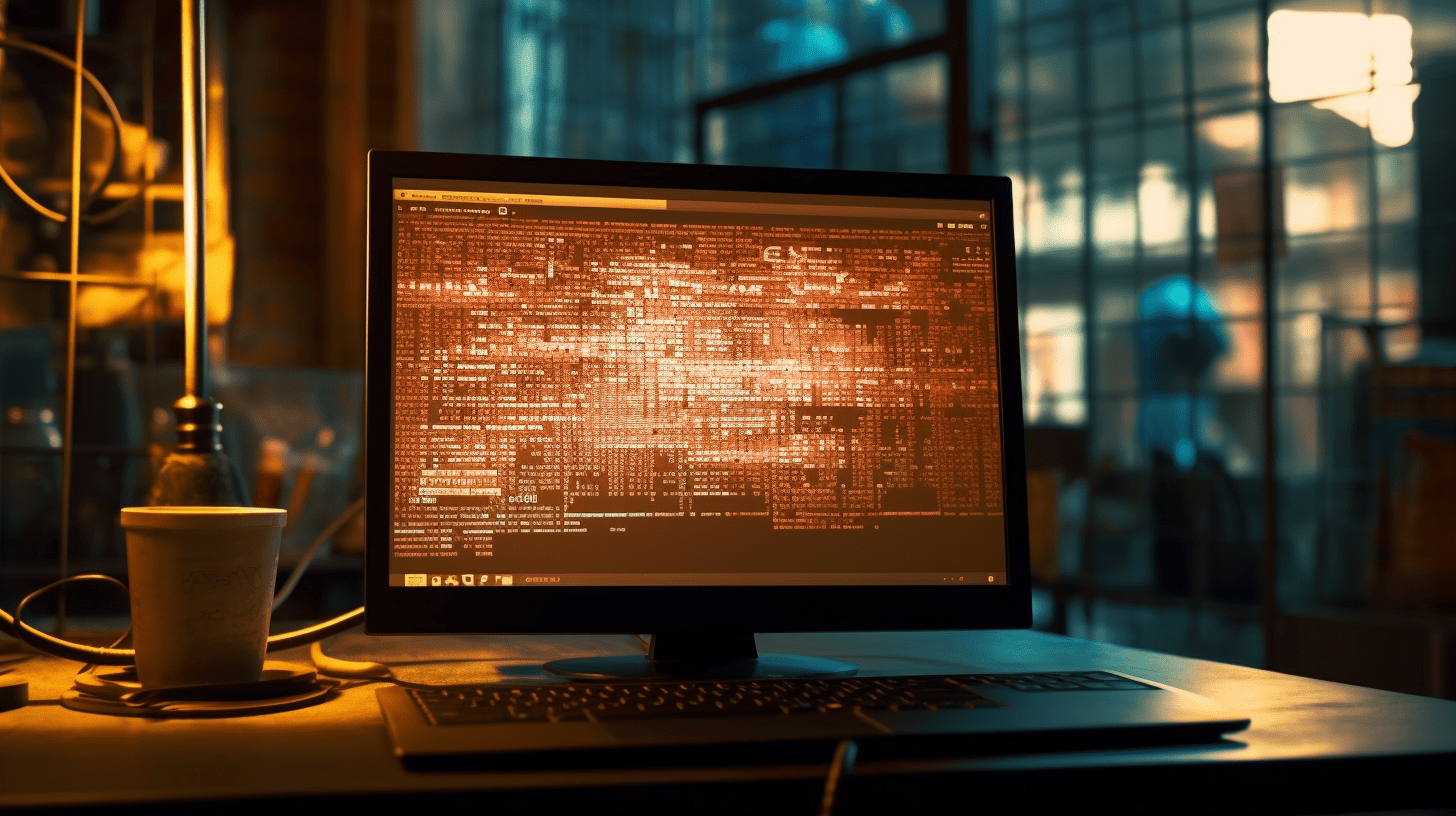
Python for Animation: Creating Graphics and Effects
The art of algorithmic motion is a fascinating intersection of creativity and mathematics, where we wield Python as our brush and canvas to paint dynamic, intricate animations. By understanding the principles of motion and employing algorithms, we can create visual experiences that are not only captivating but also deeply engaging. In this realm, every movement can be calculated, every transition programmed, and every element positioned with precision.
At its core, algorithmic motion involves defining a set of rules or parameters that dictate how objects within a scene behave over time. This can be as simple as moving an object in a straight line or as complex as simulating the physics of a bouncing ball. The beauty lies in the ability to manipulate these algorithms to yield a variety of visual effects.
Let’s ponder a basic example of animating a circle moving across the screen. The circle will change its position based on a simple linear motion algorithm. Here’s how you might implement this using Python:
import pygame import sys # Initialize Pygame pygame.init() # Set up display width, height = 800, 600 screen = pygame.display.set_mode((width, height)) pygame.display.set_caption("Algorithmic Motion Example") # Define colors white = (255, 255, 255) blue = (0, 0, 255) # Circle properties x, y = 50, height // 2 radius = 20 speed = 5 while True: for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.quit() sys.exit() # Clear the screen screen.fill(white) # Update circle position x += speed if x > width: x = 0 # Reset position # Draw the circle pygame.draw.circle(screen, blue, (x, y), radius) # Update the display pygame.display.flip() pygame.time.delay(30)
In this snippet, we set up a basic Pygame window and create a circle that moves horizontally across the screen. The circle’s position is updated in each iteration of the game loop, demonstrating a simple yet effective form of algorithmic motion.
Crafting Visual Narratives with Python
In the context of crafting visual narratives with Python, we take a deeper dive into storytelling through animation. Rather than just moving shapes for the sake of movement, we aim to convey a message or evoke emotions through our graphics. This requires a thoughtful approach, merging artistic vision with technical execution.
When we ponder about visual storytelling, it often involves a sequence of events that unfold over time. This sequence can be represented through animations where objects interact, transform, and evolve. To achieve this, we can leverage Python’s capabilities to define timelines, events, and character behaviors.
Imagine a simple narrative where a character—a rectangle—journeys across the screen, encountering obstacles in its path. The rectangle might start slowly, accelerate upon encountering an obstacle, and finally bounce back, demonstrating a series of interactions. We can implement this narrative using basic physics principles encapsulated within our code.
Here’s a Python example using Pygame that illustrates this concept:
import pygame import sys # Initialize Pygame pygame.init() # Set up display width, height = 800, 600 screen = pygame.display.set_mode((width, height)) pygame.display.set_caption("Visual Narrative Example") # Define colors white = (255, 255, 255) green = (0, 255, 0) red = (255, 0, 0) # Rectangle properties rect_x = 50 rect_y = height // 2 rect_width = 50 rect_height = 30 speed = 2 obstacles = [(400, height // 2 - 15, 100, 30)] # List of obstacles (x, y, width, height) while True: for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.quit() sys.exit() # Clear the screen screen.fill(white) # Move the rectangle rect_x += speed # Check for collision with obstacles for obs in obstacles: obs_x, obs_y, obs_width, obs_height = obs if (rect_x + rect_width > obs_x and rect_x < obs_x + obs_width and rect_y + rect_height > obs_y and rect_y < obs_y + obs_height): speed = -speed # Reverse direction on collision # Draw the rectangle and obstacle pygame.draw.rect(screen, green, (rect_x, rect_y, rect_width, rect_height)) for obs in obstacles: pygame.draw.rect(screen, red, obs) # Update the display pygame.display.flip() pygame.time.delay(30)
In this example, we create a rectangle that moves towards an obstacle. Upon collision, it reverses direction, simulating a reaction to the environment. This method of defining interactions not only builds suspense but also creates a logical flow within the animation. By manipulating the rectangle's speed and direction based on its encounters, we craft a simple yet engaging narrative.
As we progress in complexity, we can introduce more characters, intricate obstacles, and even varying backgrounds to enhance the storytelling experience. Each frame becomes a part of the narrative, allowing viewers to immerse themselves in the unfolding story driven by the underlying code.
Using Libraries for Dynamic Graphics
When we explore the world of using libraries for dynamic graphics in Python, we find ourselves standing at the threshold of vast possibilities. Python boasts a rich ecosystem of libraries specially designed to facilitate the creation of stunning graphics and animations. These libraries not only simplify the process but also extend our capabilities beyond what we could achieve with raw code alone.
One of the most popular libraries for graphics in Python is Pygame, which we’ve already seen in action. However, there are other powerful tools at our disposal, such as Matplotlib for plotting, Turtle for simple drawings, and Manim for mathematical animations. Each library has its own strengths, allowing us to choose the right one based on the specific requirements of our project.
Let’s explore how we can use Matplotlib to create dynamic animations. Matplotlib is primarily known for static plotting, but it also offers an animation module that enables us to create moving graphics. This can be particularly useful for visualizing data or creating artistic representations of functions.
Here's a simple example that demonstrates how to animate a sine wave using Matplotlib:
import numpy as np import matplotlib.pyplot as plt import matplotlib.animation as animation # Set up the figure and axis fig, ax = plt.subplots() x = np.linspace(0, 2 * np.pi, 100) line, = ax.plot(x, np.sin(x)) # Set limits ax.set_ylim(-1.5, 1.5) # Animation function def animate(i): line.set_ydata(np.sin(x + i / 10.0)) # Update the data of the line return line, # Create an animation ani = animation.FuncAnimation(fig, animate, frames=100, interval=50, blit=True) # Show the animation plt.show()
In this snippet, we first set up our axes and create a sine wave using NumPy. The animate function updates the y-data of our line for each frame, creating the illusion of motion. By calling FuncAnimation, we instruct Matplotlib to continuously update our plot, resulting in a smooth animation of the sine wave.
Another library worth mentioning is Turtle. It’s an excellent choice for beginners, providing a simpler interface to create drawings and simple animations. Turtle graphics can be particularly engaging for younger audiences. Here’s an example of a simple spinning square:
import turtle # Set up the screen screen = turtle.Screen() screen.bgcolor("white") # Create a turtle spiral_turtle = turtle.Turtle() spiral_turtle.speed(10) # Fastest drawing speed # Draw a spinning square for i in range(36): for _ in range(4): spiral_turtle.forward(100) spiral_turtle.right(90) spiral_turtle.right(10) # Spin the square # Finish turtle.done()
In this example, we create a Turtle graphics window and draw a square that spins around its center by incrementally rotating the turtle after drawing each square. The simplicity of the Turtle library allows for immediate visual feedback, making it perfect for rapid prototyping and learning.
Exploring the Aesthetics of Animation Techniques
As we delve into the aesthetics of animation techniques, we begin to appreciate the subtleties that transform basic movements into a rich, visual language. Aesthetics in animation is not merely about beautiful visuals; it encompasses principles of design, timing, and emotional resonance. In Python, we can harness various techniques to enhance the visual allure and narrative depth of our animations.
One of the fundamental principles of aesthetics is timing. The timing of movements can significantly impact how an audience perceives an animation. For instance, easing functions are commonly used to create more natural and appealing movements. These functions adjust the speed of an object as it moves, allowing for acceleration and deceleration, which can convey a sense of realism. In Python, we can implement easing functions to refine our animations.
Here’s an example demonstrating a simple linear movement versus an ease-in-out movement for a circle in Pygame:
import pygame import sys import math # Initialize Pygame pygame.init() # Set up display width, height = 800, 600 screen = pygame.display.set_mode((width, height)) pygame.display.set_caption("Easing Example") # Define colors white = (255, 255, 255) blue = (0, 0, 255) # Circle properties x, y = 50, height // 2 radius = 20 duration = 100 # Duration of the animation in frames start_time = 0 end_position = width - radius while True: for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.quit() sys.exit() # Clear the screen screen.fill(white) # Calculate the progress of the animation current_time = pygame.time.get_ticks() // 10 # Convert to frames if current_time < duration: progress = current_time / duration eased_progress = 0.5 * (1 - math.cos(progress * math.pi)) # Ease-in-out x = eased_progress * end_position else: x = end_position # Ensure the circle ends at the final position # Draw the circle pygame.draw.circle(screen, blue, (int(x), y), radius) # Update the display pygame.display.flip() pygame.time.delay(30)
In this code snippet, we utilize a basic ease-in-out formula to control the movement of a circle across the screen. By applying this easing function, we achieve a more fluid motion that feels more engaging and lifelike. This principle extends far beyond simple shapes; it can encapsulate the essence of character movements, transitions, and even scene changes.
Another vital aspect of aesthetics in animation is color theory. Colors can evoke emotions and set the mood for an animation. By carefully selecting a color palette and applying it consistently, we can enhance the viewer's experience. In Python, we can use libraries such as Pygame to create vibrant color schemes and visually striking animations. Manipulating colors dynamically can also add an extra layer of depth to our visuals.
Consider a simple example where we animate a gradient color effect on a rectangle as it moves. This showcases how we can blend colors to create a more captivating visual experience:
import pygame import sys import math # Initialize Pygame pygame.init() # Set up display width, height = 800, 600 screen = pygame.display.set_mode((width, height)) pygame.display.set_caption("Color Gradient Example") # Define colors green = (0, 255, 0) red = (255, 0, 0) # Rectangle properties rect_x = 50 rect_y = height // 2 rect_width = 100 rect_height = 50 speed = 2 while True: for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.quit() sys.exit() # Clear the screen screen.fill((255, 255, 255)) # Update rectangle position rect_x += speed if rect_x > width: rect_x = 0 # Reset position # Calculate the color based on position color_value = int(255 * (rect_x / width)) color = (color_value, 0, 255 - color_value) # Gradient from green to red # Draw the rectangle with the gradient color pygame.draw.rect(screen, color, (rect_x, rect_y, rect_width, rect_height)) # Update the display pygame.display.flip() pygame.time.delay(30)
This example demonstrates a rectangle that transitions from green to red as it moves across the screen. By dynamically calculating the color based on the rectangle's position, we create a visually engaging effect that draws the viewer's attention. Such techniques not only enhance the visual quality but also enrich the narrative conveyed through the animation.