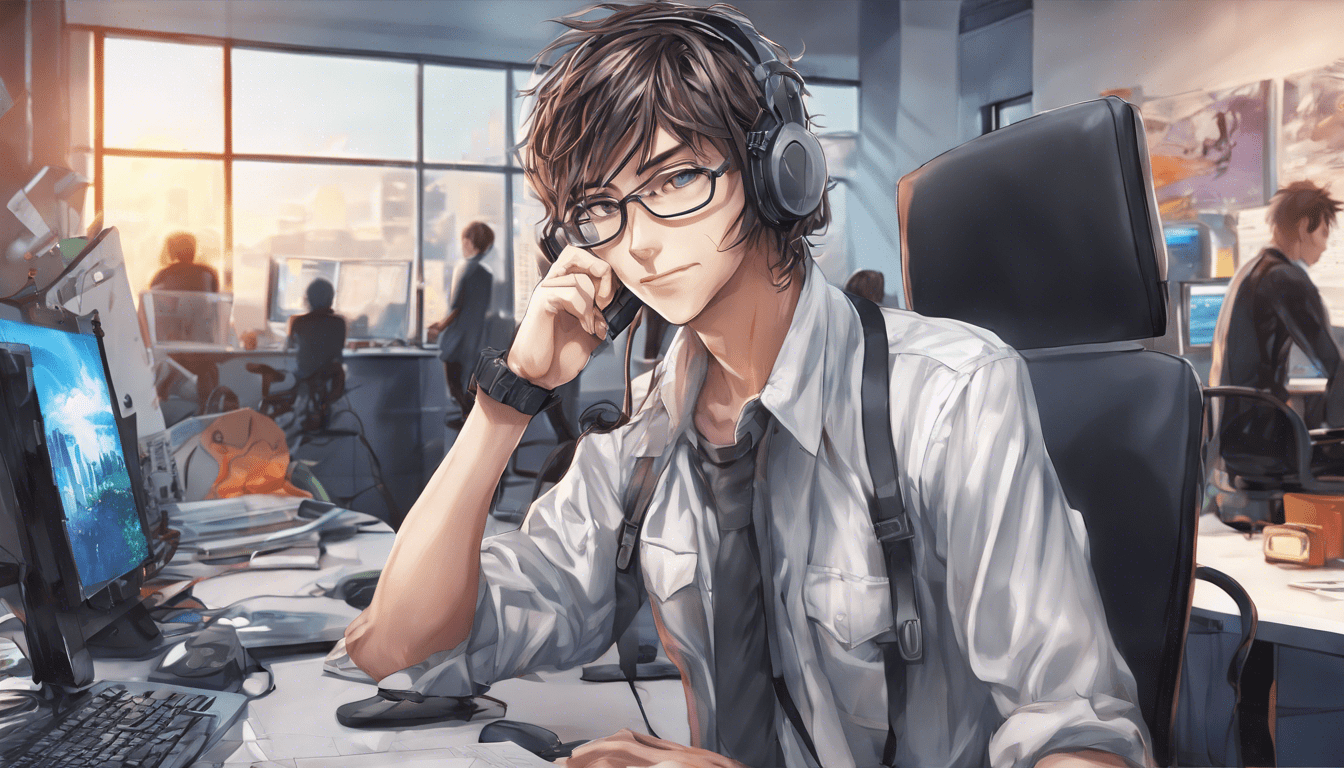
Python GUI Programming with Tkinter
Detailed Explanation of Concepts
Python GUI Programming with Tkinter allows developers to create graphical user interfaces (GUI) for their Python applications. Tkinter is a built-in Python library that provides a set of tools for GUI development. It’s widely used because of its simplicity and compatibility across different platforms.
The key concepts in Python GUI Programming with Tkinter include:
1. Creating a Window
import tkinter as tk window = tk.Tk() window.title("My First GUI")
In this example, the tkinter module is imported as “tk”. We create an instance of the Tk class, which represents the main window of our GUI application. We can set the title of the window using the “title” method.
2. Adding Widgets
Widgets are elements like buttons, labels, text boxes, etc., that we can add to our GUI to interact with the user.
label = tk.Label(window, text="Hello, World!") label.pack() button = tk.Button(window, text="Click Me!") button.pack()
In this example, we create a label widget with the text “Hello, World!” and add it to the window using the “pack” method. We also create a button widget with the text “Click Me!” and add it to the window.
3. Layout Management
Tkinter offers different layout managers to arrange widgets within a window, such as “pack”, “grid”, and “place”.
button1 = tk.Button(window, text="Button 1") button2 = tk.Button(window, text="Button 2") button3 = tk.Button(window, text="Button 3") button1.pack(side=tk.LEFT) button2.pack(side=tk.LEFT) button3.pack(side=tk.LEFT)
In this example, we create three buttons and place them side by side using the “pack” method with the “side” parameter.
4. Event Handling
Event handling allows us to respond to user actions like button clicks. We can bind functions to events using the “bind” method.
def button_click(): label.config(text="Button Clicked!") button = tk.Button(window, text="Click Me!") button.config(command=button_click) button.pack()
Step-by-Step Guide
Now let’s create a simple GUI application that greets the user:
import tkinter as tk def greet(): name = entry.get() greeting.config(text=f"Hello, name!") window = tk.Tk() window.title("Greeting App") label = tk.Label(window, text="Enter your name:") label.pack() entry = tk.Entry(window) entry.pack() button = tk.Button(window, text="Greet", command=greet) button.pack() greeting = tk.Label(window, text="") greeting.pack() window.mainloop()
Common Pitfalls and Troubleshooting Tips
Here are some common mistakes or issues beginners might encounter while using Tkinter:
- The mainloop method is necessary to start the event loop and display the window. Make sure to include it at the end of your program.
- Ensure that you import tkinter as “tk” to maintain consistency with the code examples and avoid potential import errors.
- Choose the appropriate layout manager based on your GUI requirements. Experiment with different options like “pack”, “grid”, and “place” until you achieve the desired arrangement.
- If you want to update a widget’s value dynamically, make sure to use its configuration methods like “config” or “config(text=…)” instead of creating a new widget instance.
Python GUI Programming with Tkinter is a valuable skill for developers looking to create user-friendly applications with graphical interfaces. By understanding the key concepts and following the step-by-step guide, beginners can start building their own GUI applications in Python. Remember to practice regularly and explore additional resources to deepen your knowledge and become proficient in GUI development with Tkinter.
Great article on Python GUI Programming with Tkinter! One thing to add would be the importance of handling exceptions and errors gracefully, especially when dealing with user input and interactions. Implementing try-except blocks can help manage unexpected errors. Additionally, mentioning the possibility of customizing the window and widget styles using themes or libraries like ‘ttk’ would provide more flexibility to developers looking to imropve the visual appeal of their applications.