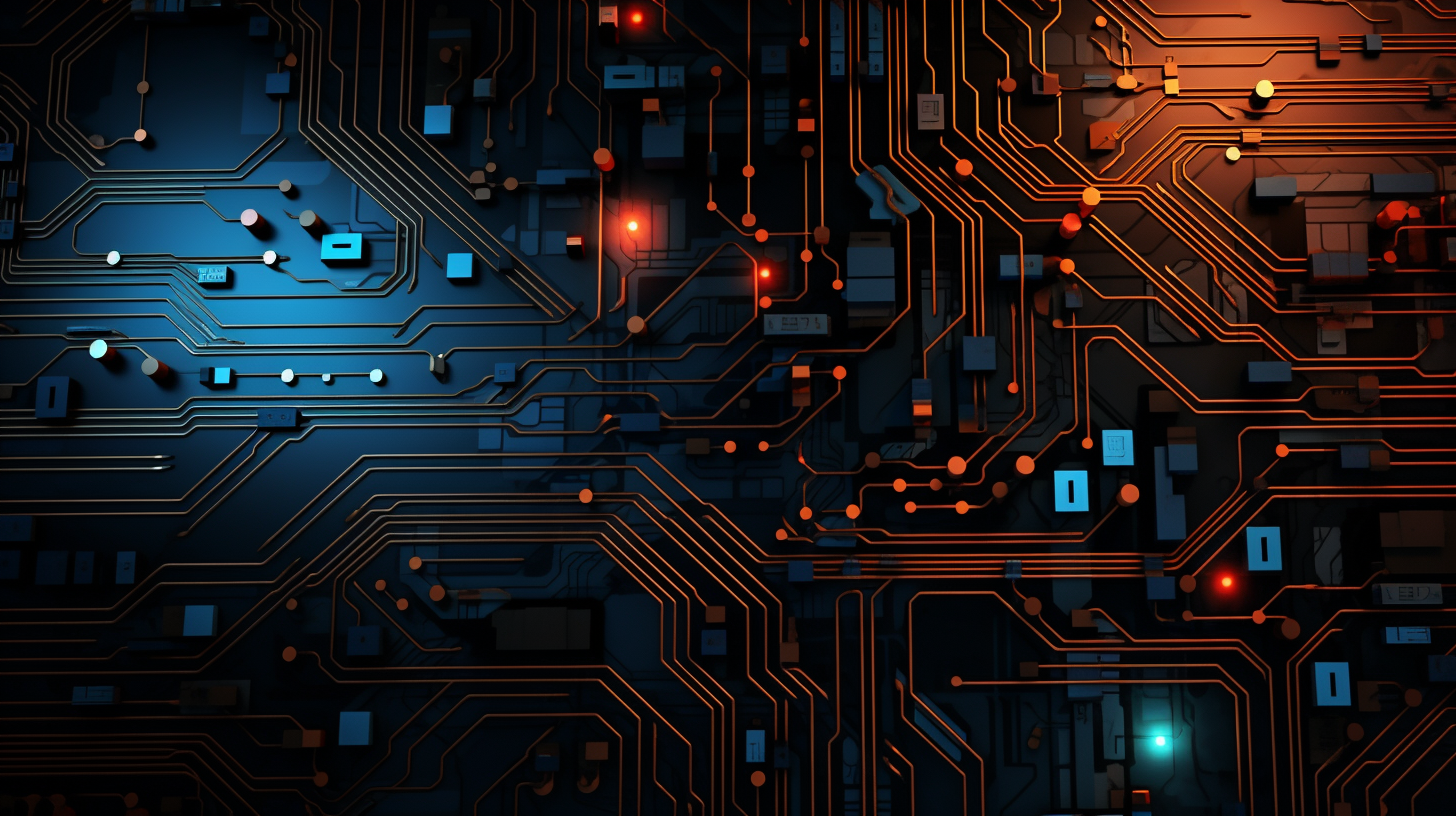
Python Lambda Functions: Anonymous Functions
Python Lambda functions, also known as anonymous functions, provide a concise way to define small and simple functions in-line. They’re particularly useful when you need to create a function without a proper name or define a short function without writing a full-fledged function definition.
Lambda functions are often used in conjunction with higher-order functions, which accept other functions as arguments or return them as results, such as filter(), map(), and reduce(). Understanding how to use lambda functions can greatly enhance your Python experience in coding.
To define a lambda function in Python, you use the lambda keyword followed by the parameters of the function, separated by commas, followed by a colon and the expression to be evaluated:
lambda arguments: expression
The lambda function takes any number of arguments and returns the result of evaluating the expression. Here’s a simple example that adds two numbers:
add = lambda x, y: x + y result = add(5, 3) print(result) # Output: 8
In this example, the lambda function takes two arguments, x and y, and returns their sum. The lambda function is assigned to the variable add, which can then be used as a regular function. When you call add(5, 3), it evaluates the expression x + y and returns the result.
One common use case of lambda functions is in sorting. The built-in sorted() function in Python allows you to sort an iterable based on a specific key. You can use a lambda function as the key parameter to customize the sorting order.
numbers = [5, 2, 8, 1, 9] sorted_numbers = sorted(numbers, key=lambda x: x % 3) print(sorted_numbers) # Output: [9, 2, 5, 8, 1]
In this example, the lambda function takes each element x from the numbers list and returns x % 3. The sorted() function uses this lambda function as the key to determine the sorting order. As a result, the numbers are sorted based on the remainder when divided by 3.
Lambda functions can also be used with higher-order functions like map(), which applies a function to each element of an iterable and returns an iterator with the results. Here’s an example that doubles each number in a list using a lambda function:
numbers = [1, 2, 3, 4, 5] doubled_numbers = list(map(lambda x: x * 2, numbers)) print(doubled_numbers) # Output: [2, 4, 6, 8, 10]
The lambda function takes each element x from the numbers list and returns x * 2. The map() function applies this lambda function to each element of the numbers list and returns an iterator with the doubled values. Finally, the list() function is used to convert the iterator into a list.
Python lambda functions are a powerful tool in your coding arsenal. They allow you to write concise and expressive code by defining small functions in-line. By combining lambda functions with higher-order functions, you can manipulate and transform data in a functional programming style. Take advantage of lambda functions to make your Python code cleaner and more elegant.