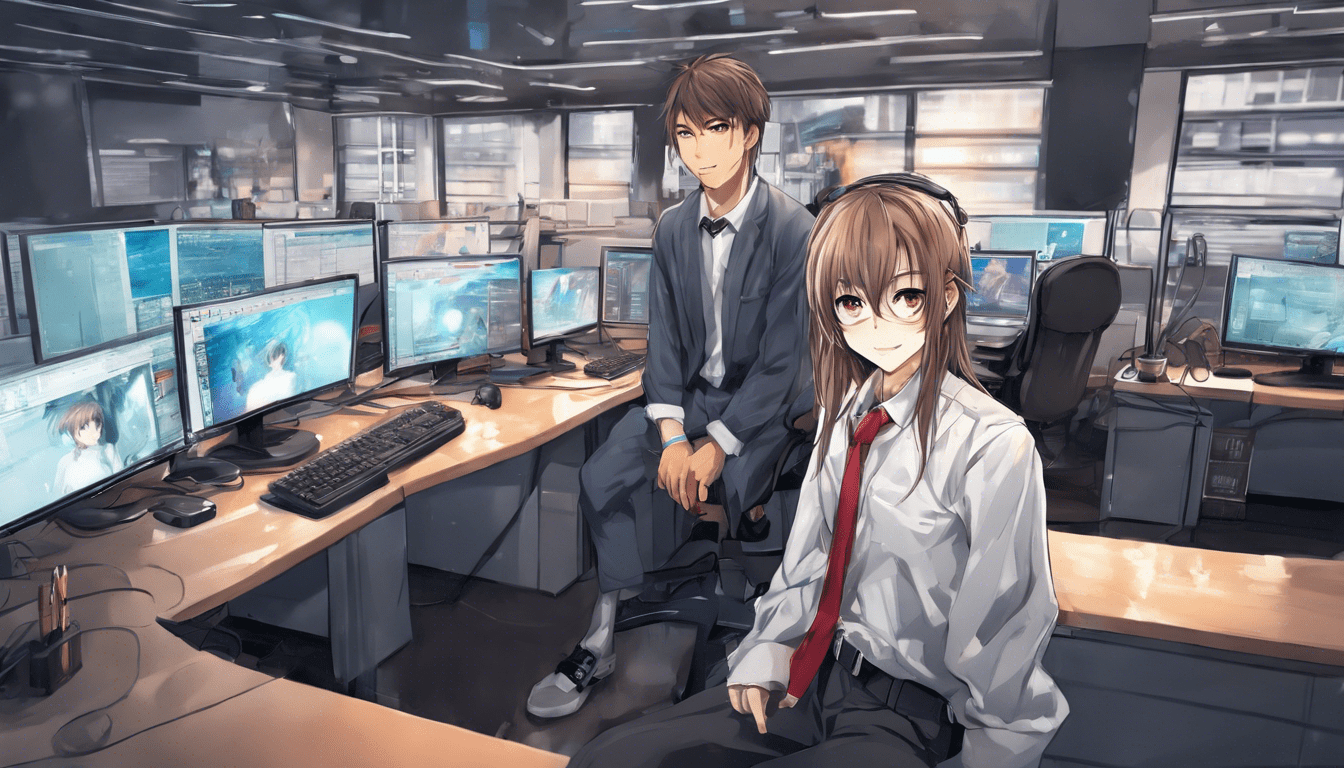
Python Scripting: Automating Simple Tasks
Python is a powerful programming language that can be used for many different purposes, including scripting. Scripting refers to the process of writing small programs that automate simple tasks. In this article, we’ll explore the basics of Python scripting and how it can be used to make your life easier.
One of the great things about Python is that it’s easy to read and write. This makes it an ideal language for beginners who are just getting started with programming. Python also comes with a large standard library that provides many useful functions for automating tasks.
To get started with Python scripting, you’ll need to have Python installed on your computer. You can download Python from python.org. Once you have Python installed, you can begin writing scripts using a text editor like Notepad or a dedicated code editor like Visual Studio Code.
Let’s start with a simple example. Suppose you want to rename all the files in a folder to have a consistent naming scheme. Instead of doing this manually, you can write a Python script to automate the task. Here’s how you might go about it:
import os # Get the list of files in the current directory files = os.listdir(".") # Loop over each file for file in files: # Check if the file is a regular file (not a directory) if os.path.isfile(file): # Split the filename into the name and extension name, ext = os.path.splitext(file) # Rename the file to have a consistent naming scheme new_name = f"file_{name}{ext}" os.rename(file, new_name)
This script uses the os
module from Python’s standard library to list the files in the current directory and rename them. It checks if each file is a regular file (not a directory) and then splits the filename into the name and extension. It then renames the file to have a consistent naming scheme.
Another common task that you might want to automate is downloading files from the internet. You can use the requests
module from Python’s standard library to do this. Here’s an example:
import requests # URL of the file you want to download url = "https://www.example.com/file.txt" # Send a GET request to the URL response = requests.get(url) # If the request was successful, save the content of the response to a file if response.status_code == 200: with open("file.txt", "wb") as file: file.write(response.content)
This script sends a GET request to the specified URL and then saves the content of the response to a file. It checks if the request was successful by checking the status code of the response.
Python scripting can also be used to automate more complex tasks, such as web scraping or data analysis. The possibilities are endless!
In conclusion, Python scripting is a powerful tool that can be used to automate simple tasks and save you time. With a little bit of practice, you’ll be able to write your own scripts and streamline your workflow.