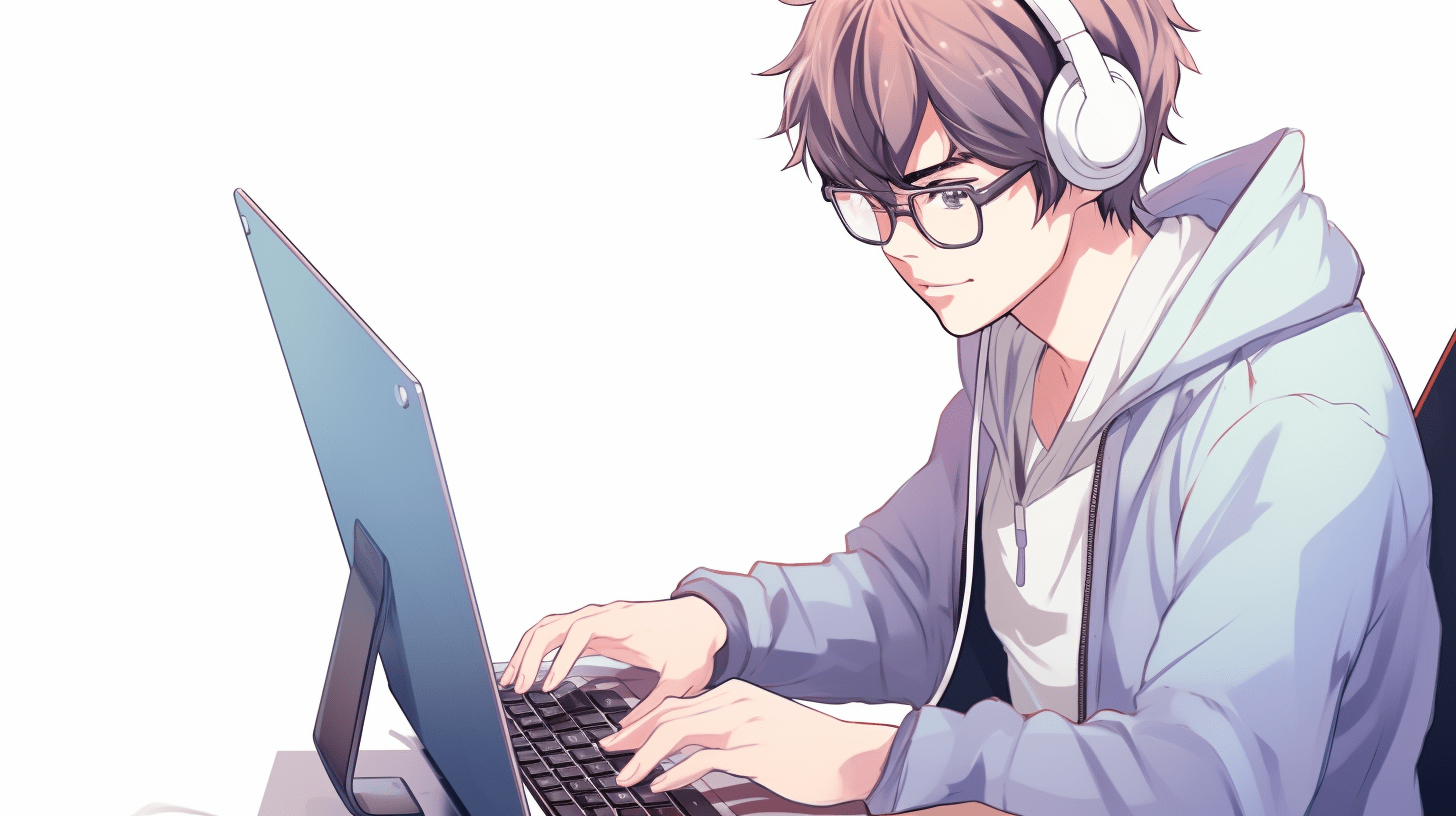
SQL for Data Classification and Organization
Data classification in SQL revolves around the meticulous organization of data into categories that can enhance retrieval, analysis, and overall management. This process involves the systematic grouping of data based on shared characteristics, which can significantly improve the efficiency of queries and the usability of datasets.
At its core, understanding data classification requires recognizing the different types of data and how they interact within a database. For instance, one might classify data into various categories such as customer data, product data, or transaction data. Each of these categories can have subcategories that allow for finer granularity, such as classifying customer data into demographic segments.
To facilitate effective data classification, SQL provides several mechanisms. One of the most fundamental is the use of structured tables where each row represents a unique instance and each column corresponds to a specific attribute of that instance. By carefully designing these tables, we can ensure that data is not only organized but also easily accessible.
Ponder the following SQL example that creates a table for classifying customer information:
CREATE TABLE Customers ( CustomerID INT PRIMARY KEY, FirstName VARCHAR(50), LastName VARCHAR(50), Email VARCHAR(100), Age INT, Gender VARCHAR(10), RegistrationDate DATE );
In this example, the Customers
table is structured to include various attributes that are crucial for classifying customers. Each column represents a specific piece of information that can be used for filtering and querying.
Moreover, data classification can also be enhanced through the use of additional tables that hold categorical data. For example, we may want to classify customers based on segments such as New, Returning, or VIP customers. This could be achieved with a simple classification schema:
CREATE TABLE CustomerSegments ( SegmentID INT PRIMARY KEY, SegmentName VARCHAR(50) ); INSERT INTO CustomerSegments (SegmentID, SegmentName) VALUES (1, 'New'), (2, 'Returning'), (3, 'VIP');
Linking our customers to these segments can be accomplished using foreign keys, providing a clearer structure and enabling more sophisticated queries. For instance, if we want to retrieve all VIP customers, we could execute:
SELECT C.FirstName, C.LastName, S.SegmentName FROM Customers C JOIN CustomerSegments S ON C.SegmentID = S.SegmentID WHERE S.SegmentName = 'VIP';
Schema Design for Optimal Data Organization
When designing a schema for optimal data organization, it is essential to consider critically about the relationships between different entities and how they will be queried. A well-structured schema not only improves the clarity of your data model but can also significantly enhance performance. In SQL, this often involves normalization, which is the process of organizing data to reduce redundancy and improve data integrity.
Normalization typically involves dividing a database into two or more tables and defining relationships between the tables. The primary goal is to eliminate duplicate data and ensure that data dependencies make sense. The first three normal forms (1NF, 2NF, and 3NF) are often the most relevant when designing a schema.
Think enhancing our Customers table by normalizing it. For instance, if we want to separate the contact details into their own table, we can create a new table that holds multiple phone numbers or addresses for each customer. Here’s how this can be implemented:
CREATE TABLE CustomerContacts ( ContactID INT PRIMARY KEY, CustomerID INT, PhoneNumber VARCHAR(15), Address VARCHAR(255), FOREIGN KEY (CustomerID) REFERENCES Customers(CustomerID) );
In this schema, the CustomerContacts table allows each customer to have multiple phone numbers and addresses, thus maintaining flexibility while keeping the Customers table cleaner and more focused on essential customer details.
Next, let’s consider the organization of product data. A well-designed product schema can significantly streamline inventory management. We may want to create a Products table that includes a foreign key to a Categories table, which classifies each product into a specific category. Here’s an example of how this can be structured:
CREATE TABLE Categories ( CategoryID INT PRIMARY KEY, CategoryName VARCHAR(50) ); CREATE TABLE Products ( ProductID INT PRIMARY KEY, ProductName VARCHAR(100), Price DECIMAL(10, 2), CategoryID INT, FOREIGN KEY (CategoryID) REFERENCES Categories(CategoryID) );
In this setup, the Categories table holds the different classifications of products, while the Products table links to it through the CategoryID foreign key. This relationship allows for effective classification and quick retrieval of products based on their category.
To show how to query products by category, consider the following SQL statement:
SELECT P.ProductName, C.CategoryName FROM Products P JOIN Categories C ON P.CategoryID = C.CategoryID WHERE C.CategoryName = 'Electronics';
This query efficiently retrieves all products within the ‘Electronics’ category, showcasing how a well-organized schema can facilitate complex queries without sacrificing performance.
Another aspect of schema design to consider is the use of indexes. Indexes can significantly speed up data retrieval by providing quick access paths to rows in a table. However, over-indexing can slow down write operations, so it especially important to balance the needs of read and write performance when deciding which columns to index.
SQL Queries for Classifying Data Effectively
To effectively classify data using SQL, it is important to leverage the full capabilities of SQL queries. These queries can be tailored to categorize and filter data based on specific requirements. By employing various SQL techniques, one can extract meaningful insights and organize data in a way that enhances overall usability.
A common approach to classifying data is through the use of GROUP BY clauses, which allows you to categorize results based on one or more columns. This can be useful for summarizing data, such as counting the number of customers in each segment or calculating the average age of customers per segment. Here’s an example:
SELECT S.SegmentName, COUNT(C.CustomerID) AS CustomerCount FROM Customers C JOIN CustomerSegments S ON C.SegmentID = S.SegmentID GROUP BY S.SegmentName;
This query groups customers by their segments, providing a count of how many customers fall into each category. Such aggregations are fundamental in understanding the distribution of data across different classifications.
Another powerful SQL feature is the use of CASE statements, which allows for conditional logic within your queries. This can be particularly useful for creating derived classifications or categories based on existing data. For instance, if you want to classify customers into ‘Minor’, ‘Adult’, and ‘Senior’ based on their age, you can use:
SELECT FirstName, LastName, CASE WHEN Age < 18 THEN 'Minor' WHEN Age BETWEEN 18 AND 65 THEN 'Adult' ELSE 'Senior' END AS AgeGroup FROM Customers;
By using the CASE statement, you can generate a new column that classifies each customer into an age group. This approach allows for greater flexibility in how data is presented and can facilitate more targeted marketing strategies, for example.
Moreover, SQL's JOIN operations can be instrumental in classifying data across multiple related tables. By joining tables, you can access and combine related data easily, leading to richer datasets for classification. For example, to retrieve not only customers but also their corresponding segment names and average purchase amounts, you might do the following:
SELECT C.FirstName, C.LastName, S.SegmentName, AVG(T.Amount) AS AvgPurchase FROM Customers C JOIN CustomerSegments S ON C.SegmentID = S.SegmentID LEFT JOIN Transactions T ON C.CustomerID = T.CustomerID GROUP BY C.CustomerID, S.SegmentName;
In this query, we join the Customers table with both the CustomerSegments and Transactions tables, allowing us to classify customers not just by segment but also by their purchasing behavior. This level of detail provides valuable insights into customer behavior and aids in further classification efforts.
Lastly, employing HAVING clauses in conjunction with GROUP BY can filter aggregated results, providing another layer of classification. For instance, if we want to see only those segments with more than 10 customers, we can modify our earlier query:
SELECT S.SegmentName, COUNT(C.CustomerID) AS CustomerCount FROM Customers C JOIN CustomerSegments S ON C.SegmentID = S.SegmentID GROUP BY S.SegmentName HAVING COUNT(C.CustomerID) > 10;
Using the HAVING clause allows us to focus on significant data points, ensuring that we only analyze segments that meet our threshold criteria. This can streamline decision-making and reporting processes.
Using Indexing to improve Data Retrieval
Indexing is a vital technique in SQL that can drastically improve data retrieval times by providing a mechanism for quickly locating rows within a table. When a database table grows large, the performance of queries can significantly degrade if proper indexing strategies are not employed. Understanding how to use indexes effectively can enhance the efficiency of data classification and organization in SQL.
At its simplest, an index is a data structure that improves the speed of data retrieval operations on a database table at the cost of additional space and decreased performance in data modification operations. When designing indexes, one must think which columns will be queried frequently and whether those columns are used in filtering, sorting, or joining operations.
To create an index, SQL provides a simpler command structure. Here’s a simple example of how to create an index on the Email
column of the Customers
table:
CREATE INDEX idx_email ON Customers(Email);
This index allows the database to quickly locate rows in the Customers
table based on the Email
field. It is beneficial in scenarios where queries frequently filter or search for customers by their email addresses.
However, indexing is not without its trade-offs. While it can speed up read operations, it can slow down write operations because the index must be updated whenever data is inserted, updated, or deleted. Therefore, it very important to balance the number and type of indexes you create based on your application's specific read and write patterns.
Additionally, composite indexes can be created to enhance the performance of queries that filter on multiple columns. For instance, if you often query customers by both LastName
and FirstName
, creating a composite index on these columns can be advantageous:
CREATE INDEX idx_name ON Customers(LastName, FirstName);
This composite index will optimize queries that filter by both names, allowing for faster retrieval of customer records. For example, the following query benefits from this index:
SELECT * FROM Customers WHERE LastName = 'Doe' AND FirstName = 'John';
Moreover, SQL Server and other databases provide options for unique indexes, which ensure that the indexed columns do not contain duplicate values. This can be particularly useful for columns like Email
or CustomerID
, where uniqueness is a requirement:
CREATE UNIQUE INDEX idx_unique_email ON Customers(Email);
Another critical aspect of indexing is understanding when to use clustered versus non-clustered indexes. A clustered index determines the physical order of data in the table, which means a table can only have one clustered index. In contrast, non-clustered indexes are separate from the data and can point to the data rows. When performance is a priority, the choice of which type to use depends on how the data is accessed.
For instance, in a transaction-heavy application where new records are frequently added, a non-clustered index might be more appropriate to avoid performance hits during insert operations. On the other hand, for read-heavy applications, a clustered index on frequently accessed columns can yield substantial performance improvements.
Best Practices for Data Validation and Integrity
The integrity and validation of data in SQL databases are paramount to ensuring that the data you analyze is accurate, reliable, and meaningful. Best practices for data validation and integrity involve implementing strategies that prevent errors during data entry, maintain data quality, and enforce business rules. By focusing on these areas, you can foster a solid foundation for effective data classification and organization.
One of the fundamental aspects of ensuring data integrity is to use constraints when designing your tables. Constraints are rules that the SQL database enforces on the data within a table. They can restrict the type of data that can be entered, ensuring that only valid entries are made. Here are some common types of constraints:
- Ensures that a column cannot have a NULL value.
- Ensures that all values in a column are distinct.
- Ensures that all values in a column satisfy a specific condition.
- Maintains referential integrity by ensuring that a value in one table corresponds to a valid entry in another table.
Think the Customers table we previously defined. We can enhance its integrity by enforcing constraints. For example:
CREATE TABLE Customers ( CustomerID INT PRIMARY KEY, FirstName VARCHAR(50) NOT NULL, LastName VARCHAR(50) NOT NULL, Email VARCHAR(100) NOT NULL UNIQUE, Age INT CHECK (Age >= 0), Gender VARCHAR(10), RegistrationDate DATE DEFAULT CURRENT_DATE );
In this example, we have ensured that the FirstName and LastName cannot be NULL, that Email values must be unique, and that Age cannot be negative. Such constraints actively prevent data anomalies and maintain the quality of your dataset.
Beyond constraints, implementing validation rules during data entry can significantly enhance data integrity. That is often achieved through the use of stored procedures or triggers. Stored procedures can encapsulate complex logic for inserting or updating records. Triggers, on the other hand, can automatically enforce rules whenever data is modified.
For instance, if you want to ensure that an email address entered into the Customers table is of a valid format, you could create a trigger:
CREATE TRIGGER trg_validate_email BEFORE INSERT OR UPDATE ON Customers FOR EACH ROW BEGIN IF NOT NEW.Email LIKE '%_@__%.__%' THEN SIGNAL SQLSTATE '45000' SET MESSAGE_TEXT = 'Invalid email format'; END IF; END;
This trigger checks the email format before any insert or update operation and raises an error if the format is invalid, thus preserving the integrity of the data.
Another essential practice is to perform regular audits of your data. By periodically checking for anomalies, duplicates, and discrepancies, you can proactively identify and rectify issues. SQL provides various tools for this, such as the COUNT function to find duplicates or using GROUP BY along with HAVING to identify entries that do not conform to expected norms.
SELECT Email, COUNT(*) FROM Customers GROUP BY Email HAVING COUNT(*) > 1;
This query helps identify duplicate email entries in the Customers table, which will allow you to take corrective action swiftly.
Lastly, documenting your database schema, including the constraints and validation rules you have implemented, is a best practice that can foster understanding among team members and ensure consistency in data handling over time. Clear documentation serves as a guide for future development and maintenance, ensuring everyone involved in the database's lifecycle knows how to interact with it safely and effectively.
Advanced Techniques for Dynamic Data Classification
-- To demonstrate dynamic data classification, we can create a procedure that adjusts the customer segment based on their purchase behavior. CREATE PROCEDURE UpdateCustomerSegments() BEGIN -- Update customers to 'VIP' if their total purchase exceeds a certain threshold UPDATE Customers C SET C.SegmentID = (SELECT SegmentID FROM CustomerSegments WHERE SegmentName = 'VIP') WHERE C.CustomerID IN ( SELECT CustomerID FROM Transactions GROUP BY CustomerID HAVING SUM(Amount) > 1000 -- Threshold for being classified as VIP ); -- Update customers to 'Returning' if they have made more than one purchase UPDATE Customers C SET C.SegmentID = (SELECT SegmentID FROM CustomerSegments WHERE SegmentName = 'Returning') WHERE C.CustomerID IN ( SELECT CustomerID FROM Transactions GROUP BY CustomerID HAVING COUNT(*) > 1 -- Threshold for being classified as Returning ); -- Default to 'New' if no other classification applies UPDATE Customers C SET C.SegmentID = (SELECT SegmentID FROM CustomerSegments WHERE SegmentName = 'New') WHERE C.CustomerID NOT IN ( SELECT CustomerID FROM Transactions ); END;
Dynamic data classification enables adaptability within your database, allowing for classifications that evolve based on the underlying data. This very important, especially in environments where customer behaviors and interactions constantly change. One of the most effective ways to implement dynamic classification is through the use of stored procedures that can be triggered based on specific events or at regular intervals.
The preceding SQL example showcases a stored procedure designed to update customer segments based on their purchasing behavior. By classifying customers dynamically, we can ensure that the segments remain relevant and actionable. The procedure not only elevates high-value customers to the 'VIP' segment based on cumulative purchases but also identifies 'Returning' customers who show loyalty through repeat transactions. Those who have made no purchases are defaulted to 'New', ensuring that every customer is categorized appropriately.
To invoke this procedure after significant transactional changes, one could set it to run automatically via a scheduled job or trigger it after each batch of transactions. For instance, if new transactions are added to the database, executing this procedure would immediately re-evaluate the segments of all customers, ensuring timely updates.
Another advanced technique for dynamic data classification employs the use of views. Views can present a curated dataset to users while encapsulating complex logic that determines how data is categorized. By creating a view that incorporates classification logic, you can simplify the querying process for the end-user while maintaining a robust classification scheme in the background.
Below is an example of how you could create a view that dynamically categorizes customers based on their purchase frequency and average spending:
CREATE VIEW CustomerClassification AS SELECT C.CustomerID, C.FirstName, C.LastName, CASE WHEN COUNT(T.TransactionID) > 10 THEN 'Frequent' WHEN AVG(T.Amount) > 100 THEN 'High Spender' ELSE 'Occasional' END AS CustomerCategory FROM Customers C LEFT JOIN Transactions T ON C.CustomerID = T.CustomerID GROUP BY C.CustomerID, C.FirstName, C.LastName;
This view aggregates customers and classifies them into categories such as 'Frequent', 'High Spender', or 'Occasional' based on their transaction history. This classification can be beneficial for targeting marketing efforts or making data-driven decisions regarding customer engagement strategies.
When dealing with dynamic data classification, it is also crucial to ponder performance. Complex queries that involve aggregations and classification logic can become resource-intensive. Techniques such as indexing on frequently queried columns and optimizing your SQL queries for better execution plans become vital. Regular monitoring and performance tuning should accompany any dynamic classification strategy.