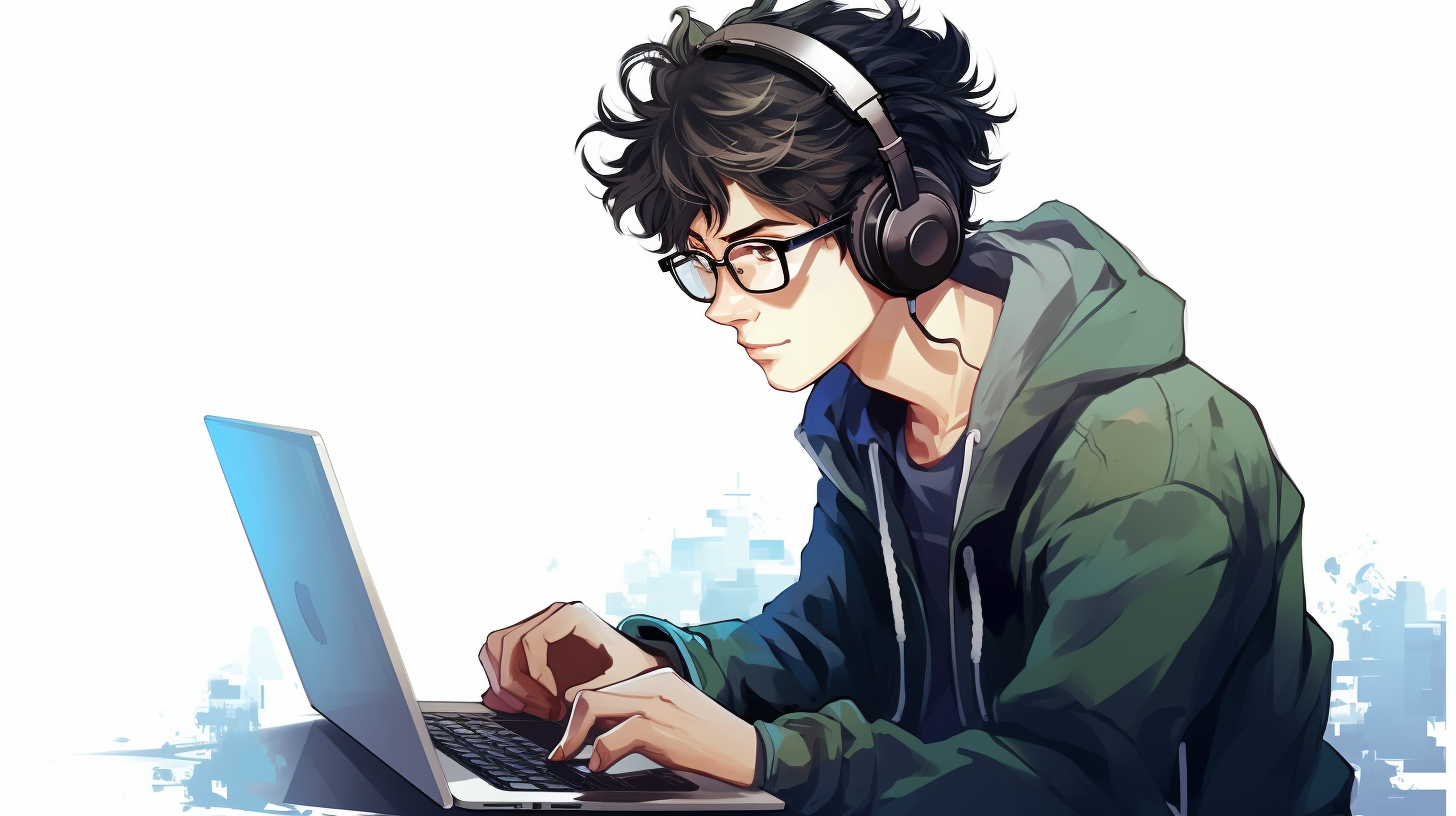
SQL for Data Redundancy Elimination
Data redundancy is a common issue in database management that can lead to inconsistencies, storage inefficiencies, and increased complexity in data retrieval. In order to maintain data integrity and optimize database performance, it’s important to implement strategies for eliminating data redundancy through SQL.
One of the primary techniques for data redundancy elimination in SQL is normalization. Normalization involves breaking down tables into smaller, more focused tables, and establishing relationships between them using foreign keys. This process reduces duplication of data and ensures that each piece of data is stored only once in the database.
Let’s take a look at an example of normalization:
CREATE TABLE Customers ( CustomerID INT PRIMARY KEY, FirstName VARCHAR(50), LastName VARCHAR(50), Address VARCHAR(100), City VARCHAR(50), PostalCode VARCHAR(10), Country VARCHAR(50) ); CREATE TABLE Orders ( OrderID INT PRIMARY KEY, CustomerID INT, OrderDate DATE, TotalAmount DECIMAL(10, 2), FOREIGN KEY (CustomerID) REFERENCES Customers(CustomerID) );
In the above example, we have separated the customer information from the orders by creating two tables: Customers and Orders. The Customers table holds the customer information, while the Orders table holds the order details. The CustomerID column in the Orders table is a foreign key that references the CustomerID in the Customers table, creating a relationship between the two tables and eliminating data redundancy.
Another technique for redundancy elimination is the use of unique constraints and indexes. By ensuring that certain columns or combinations of columns are unique within a table, we can prevent duplicate entries and maintain data integrity.
An example of adding a unique constraint:
ALTER TABLE Customers ADD CONSTRAINT UC_Customer UNIQUE (FirstName, LastName, Address);
In this example, we’ve added a unique constraint named UC_Customer to the Customers table. This constraint ensures that the combination of FirstName, LastName, and Address is unique across all records in the table, which helps to prevent redundant data.
SQL also provides tools to identify and remove redundant data. The DISTINCT keyword, for example, can be used in a SELECT statement to return only unique records from a query result:
SELECT DISTINCT FirstName, LastName, Address FROM Customers;
In this query, the DISTINCT keyword will return only unique combinations of FirstName, LastName, and Address, eliminating any redundancy in the result set.
To wrap it up, SQL provides various mechanisms to eliminate data redundancy, such as normalization, use of unique constraints and indexes, and the distinct keyword. By implementing these strategies in your database design and queries, you can maintain data integrity, reduce storage requirements, and improve query performance.