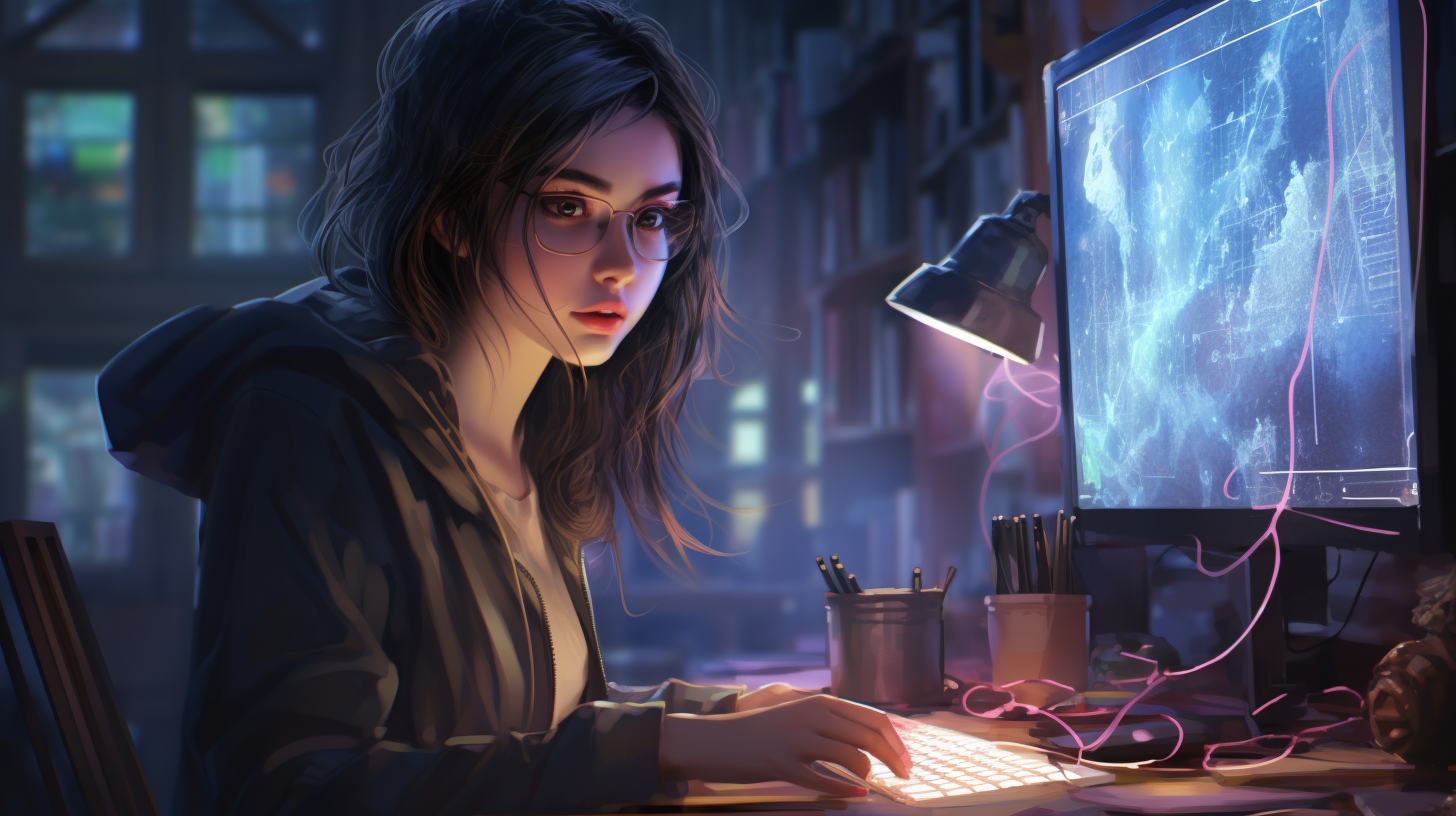
SQL for Time Series Data Analysis
Time series data analysis is an essential part of understanding trends, patterns, and anomalies in datasets that are indexed by time. SQL is a powerful tool for managing and analyzing this type of data. In this article, we will explore some of the key techniques for working with time series data in SQL, including storing, querying, aggregating, and window functions.
Storing Time Series Data
The first step in working with time series data is to store it in a way that makes it easy to query and analyze. A typical time series table structure in SQL might look like this:
CREATE TABLE sales ( sale_id INT AUTO_INCREMENT PRIMARY KEY, sale_date DATETIME NOT NULL, sale_amount DECIMAL(10, 2) NOT NULL );
This table has a primary key `sale_id`, a `sale_date` column that records the time when each sale occurred, and a `sale_amount` column that records the amount of each sale.
Querying Time Series Data
Once you have stored your time series data, you can start querying it. Some common time-based queries might include selecting entries within a certain date range or at a specific time interval. Here’s an example:
SELECT * FROM sales WHERE sale_date BETWEEN '2021-01-01' AND '2021-01-31';
This query selects all sales that occurred in January 2021.
Aggregating Time Series Data
Aggregating time series data by days, weeks, months, or other intervals is a common way to look for trends. Here is an example of how to aggregate sales data by month:
SELECT DATE_FORMAT(sale_date, '%Y-%m') AS sale_month, SUM(sale_amount) AS total_sales FROM sales GROUP BY sale_month ORDER BY sale_month;
This query groups sales by month and calculates the total sales for each month.
Window Functions
Window functions are essential in time series data analysis as they allow for calculations across a set of rows related to the current row. An example of a window function is calculating a rolling average. Here’s a simple rolling average over three days:
SELECT sale_date, sale_amount, AVG(sale_amount) OVER ( ORDER BY sale_date ROWS BETWEEN 2 PRECEDING AND CURRENT ROW ) AS rolling_average FROM sales;
This will provide the average sales amount for the current day plus the previous two days for each row in our sales table.
Working with time series data in SQL requires understanding how to store, query, aggregate, and use window functions on your data based on time elements. With the right techniques, SQL can be an incredibly powerful tool for making sense of time series data and extracting meaningful insights from it.