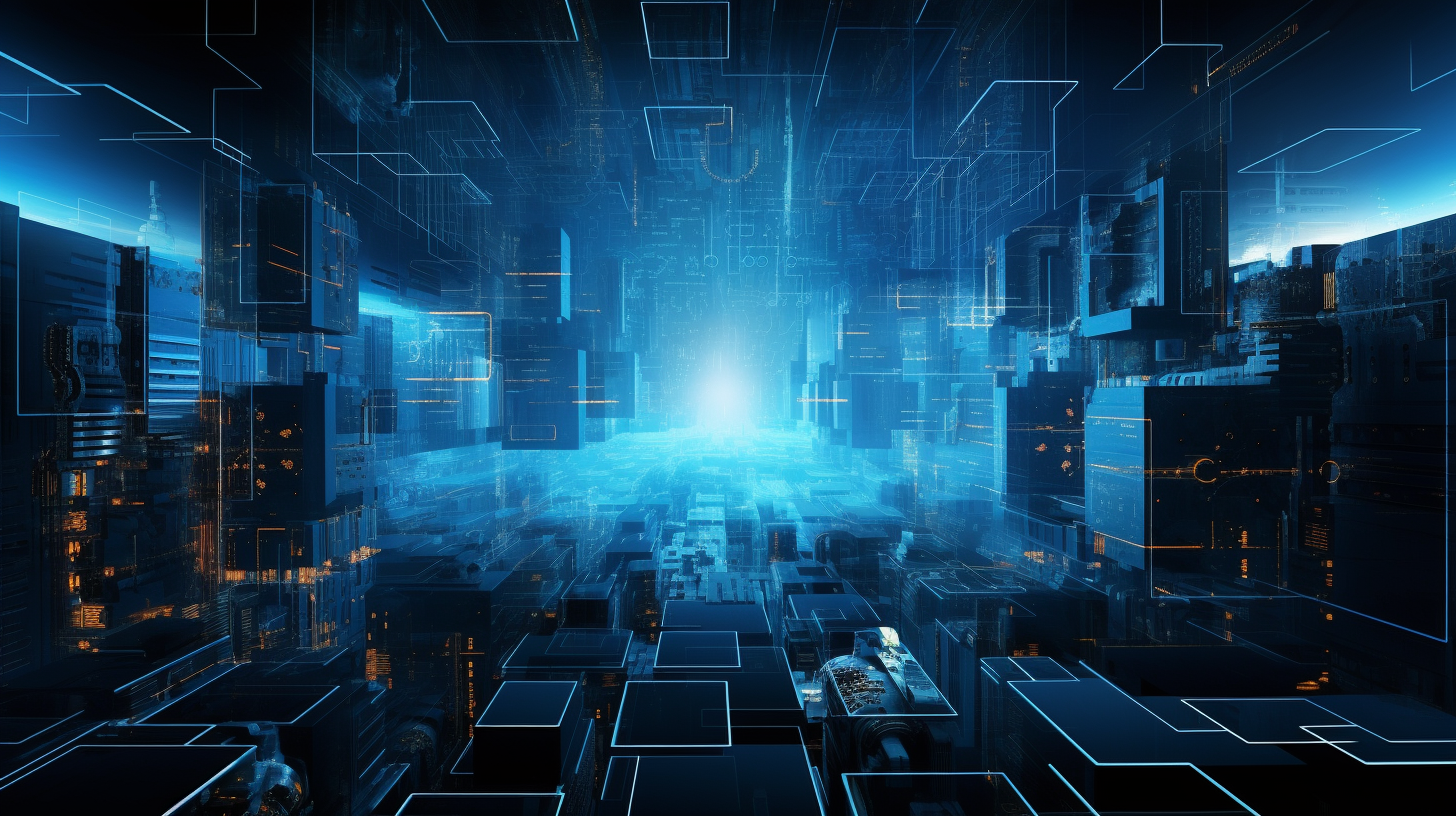
Python in Healthcare: Applications and Case Studies
Python is a widely used programming language in various industries, including healthcare. Its versatility and extensive libraries allow developers to build applications and analyze medical data efficiently. In this article, we will explore the applications and case studies of Python in healthcare, highlighting its significance in this field.
Medical Data Processing and Analysis
Python provides a robust platform for processing and analyzing medical data, including electronic health records (EHR), medical images, and genomics data. With libraries like NumPy, Pandas, and SciPy, developers can easily manipulate and analyze large datasets.
Let’s think an example of analyzing patient data using Python:
import pandas as pd # Load the dataset data = pd.read_csv('patient_data.csv') # Calculate basic statistics average_age = data['Age'].mean() max_blood_pressure = data['Blood Pressure'].max() # Visualize the data data['Age'].plot(kind='hist')
With just a few lines of code, we can load a dataset, calculate basic statistics, and visualize the data using Python libraries.
Machine Learning in Healthcare
Python’s extensive libraries for machine learning, such as scikit-learn and TensorFlow, make it a popular choice for developing healthcare applications that leverage artificial intelligence (AI) algorithms.
Here’s an example of using machine learning to predict patient readmission:
from sklearn.model_selection import train_test_split from sklearn.linear_model import LogisticRegression # Prepare the dataset X = data[['Age', 'Blood Pressure', 'Cholesterol']] y = data['Readmission'] X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2) # Train the model model = LogisticRegression() model.fit(X_train, y_train) # Make predictions predictions = model.predict(X_test)
In this example, we split the dataset into training and testing sets, train a logistic regression model, and make predictions on the test set. Python’s machine learning libraries simplify the implementation of such algorithms.
Healthcare Data Visualization
Visualizing healthcare data is important for better understanding and decision-making. Python provides various libraries, including Matplotlib and Plotly, for creating insightful visualizations.
Let’s create a simple bar chart to visualize patient demographics:
import matplotlib.pyplot as plt # Prepare the data age_groups = data['Age'].value_counts() age_groups = age_groups.sort_index() # Create a bar chart plt.bar(age_groups.index, age_groups.values) plt.xlabel('Age') plt.ylabel('Number of Patients') plt.title('Patient Demographics') plt.show()
In this code snippet, we create a bar chart showing the number of patients in different age groups using Matplotlib. Python’s visualization libraries offer flexibility and customization options for creating impactful healthcare visualizations.
Case Study: Predicting Disease Outcomes with Python
One real-world application of Python in healthcare is predicting disease outcomes based on patient information. Let’s think a case study where we predict the progression of heart disease using machine learning.
First, we need to prepare the dataset and split it into training and testing sets:
import pandas as pd from sklearn.model_selection import train_test_split # Load the dataset data = pd.read_csv('heart_disease.csv') # Prepare the dataset X = data.drop('target', axis=1) y = data['target'] # Split the dataset X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2)
Next, we train a Random Forest classifier and evaluate its performance:
from sklearn.ensemble import RandomForestClassifier from sklearn.metrics import accuracy_score # Train the model model = RandomForestClassifier() model.fit(X_train, y_train) # Make predictions predictions = model.predict(X_test) # Evaluate the model accuracy = accuracy_score(y_test, predictions) print(f"Accuracy: {accuracy}")
By using Python’s machine learning libraries, we can build predictive models to assist in disease outcome prediction. The Random Forest classifier in this case study offers high accuracy in predicting heart disease progression.
It’s interesting to note the emphasis on Python’s role in healthcare. However, it would be beneficial to also discuss the ethical considerations and challenges of using Python in medical data analysis, such as data privacy concerns, the need for transparency in AI algorithms, and the potential for bias in machine learning models. These aspects are critical for ensuring that the technology not only advances healthcare but does so responsibly and equitably.