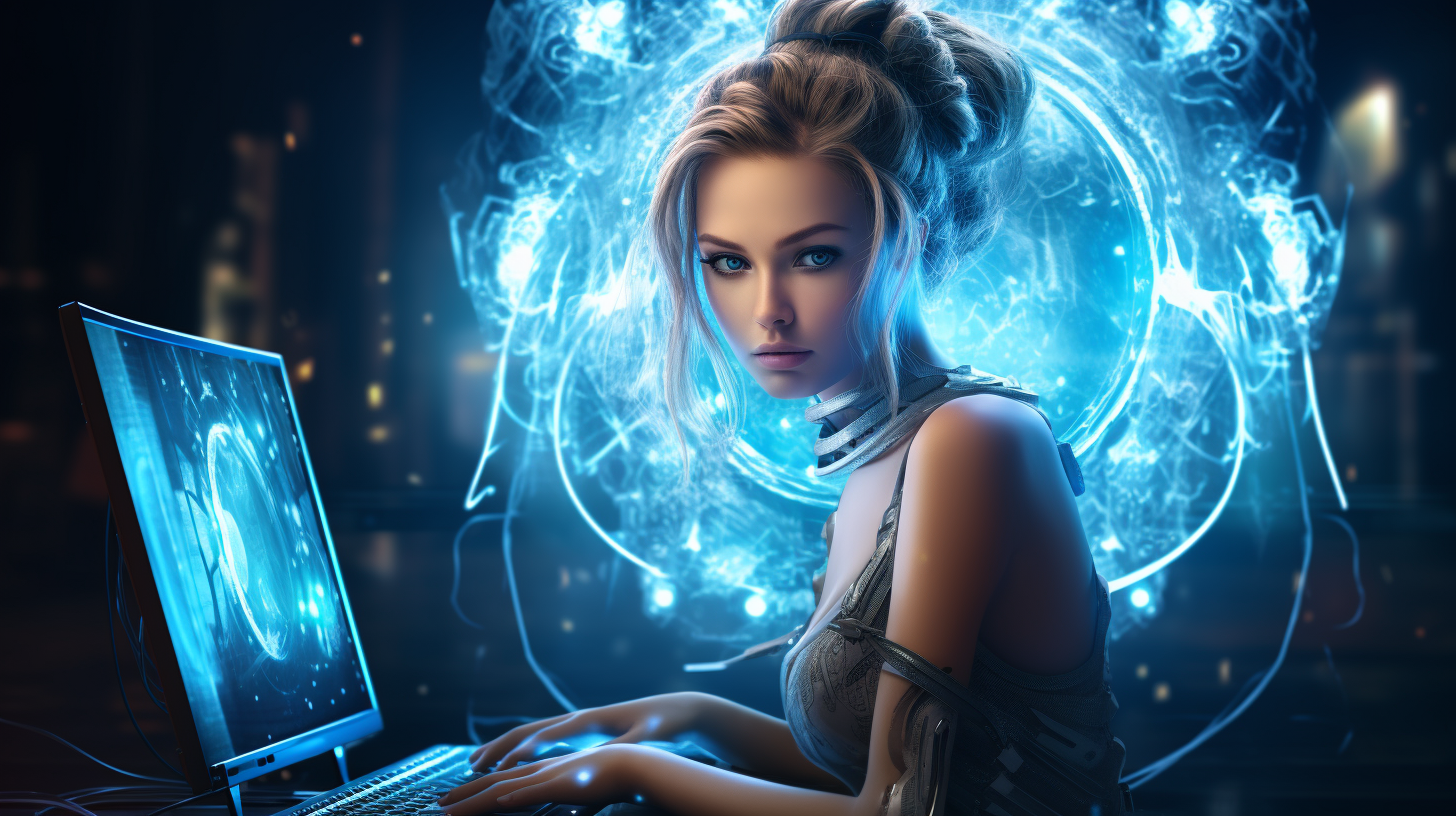
Swift and CoreBluetooth Advanced
Exploring Advanced CoreBluetooth Features
CoreBluetooth is a powerful framework that allows iOS developers to interact with Bluetooth Low Energy (BLE) devices. While the basic features of CoreBluetooth are simpler to use, there are advanced features that can provide a more robust and efficient interaction with BLE devices.
One such feature is the use of background execution for Bluetooth apps. This allows an app to continue to interact with BLE devices even when it’s not the foreground app. To enable background execution, you must set the UIBackgroundModes
key in your app’s Info.plist file to include bluetooth-central
.
<key>UIBackgroundModes</key> <array> <string>bluetooth-central</string> </array>
Another advanced feature is the ability to connect to multiple peripherals at once. This allows your app to interact with several BLE devices concurrently. To do this, you can use the retrieveConnectedPeripherals(withServices:)
method to find peripherals that are already connected to the system and meet certain criteria.
let services = [CBUUID(string: "YOUR_SERVICE_UUID")] let connectedPeripherals = centralManager.retrieveConnectedPeripherals(withServices: services)
Additionally, state preservation and restoration is an advanced feature that allows your app to be relaunched into the same state it was in before it was terminated by the system. That’s particularly useful for Bluetooth apps that need to maintain a connection to a peripheral. To use this feature, you must opt in by setting the CBPeripheralManagerOptionRestoreIdentifierKey
and CBCentralManagerOptionRestoreIdentifierKey
options when creating your CBPeripheralManager
and CBCentralManager
objects.
let options = [CBCentralManagerOptionRestoreIdentifierKey: "yourCentralManagerIdentifier"] let centralManager = CBCentralManager(delegate: self, queue: nil, options: options)
Finally, CoreBluetooth allows for customizing the scan mode for peripherals. This can be done by setting the CBCentralManagerScanOptionAllowDuplicatesKey
option to true
when calling the scanForPeripherals(withServices:options:)
method. This will allow your app to receive multiple advertisements from the same peripheral, which can be useful for certain use cases.
let scanOptions = [CBCentralManagerScanOptionAllowDuplicatesKey: true] centralManager.scanForPeripherals(withServices: nil, options: scanOptions)
These advanced features of CoreBluetooth can greatly enhance the capabilities of your Bluetooth-enabled iOS app. By using background execution, simultaneous connections, state preservation and restoration, and customized scan modes, you can create a more seamless and powerful experience for your users.
Implementing Swift Best Practices for Bluetooth Development
When developing Bluetooth applications in Swift, it’s essential to implement best practices to ensure the app is reliable, efficient, and effortless to handle. One of the key practices is to manage the Bluetooth state and permissions effectively. It is important to check if Bluetooth is powered on and if the app has the necessary permissions to use Bluetooth features. This can be done by implementing the centralManagerDidUpdateState
method of the CBCentralManagerDelegate
.
func centralManagerDidUpdateState(_ central: CBCentralManager) { switch central.state { case .poweredOn: // Bluetooth is on // Start scanning for devices case .poweredOff, .resetting, .unauthorized, .unknown, .unsupported: // Handle each case appropriately } }
Another best practice is to keep the UI responsive during Bluetooth operations. Since Bluetooth tasks can take an indeterminate amount of time, it’s advisable to perform these tasks on a background thread. Using DispatchQueue
to handle Bluetooth tasks can prevent blocking the main thread and keep the UI interactive.
DispatchQueue.global(qos: .background).async { // Perform Bluetooth tasks }
Proper error handling is also crucial in Bluetooth development. Swift provides a robust error handling system with do-catch
blocks, which can be utilized to gracefully handle any errors that may occur during Bluetooth operations.
do { // Attempt a Bluetooth operation that may throw an error } catch let error { // Handle the error }
Moreover, to maintain a clean and maintainable codebase, it’s recommended to encapsulate Bluetooth functionality into separate classes or structs. This separation of concerns can make the code easier to read and debug. It is also beneficial to use protocols and delegation to communicate between the Bluetooth classes and the rest of the app.
- Delegate Protocol
protocol BluetoothManagerDelegate: AnyObject { func didDiscoverPeripheral(_ peripheral: CBPeripheral) // Other delegate methods }
- Bluetooth Manager
class BluetoothManager: NSObject, CBCentralManagerDelegate { weak var delegate: BluetoothManagerDelegate? // Bluetooth related methods }
Lastly, it is essential to clean up resources when they’re no longer needed. For instance, when the app is done interacting with a BLE device, it is important to call cancelPeripheralConnection
to disconnect from the peripheral and free up any resources used during the connection.
centralManager.cancelPeripheralConnection(peripheral)
By following these Swift best practices for Bluetooth development, developers can create efficient, reliable, and simple to operate Bluetooth applications that provide a seamless experience for the end-users.
Troubleshooting Common Issues in CoreBluetooth Integration
Troubleshooting common issues in CoreBluetooth integration can be a daunting task. However, with the right approach and understanding of the framework, developers can quickly identify and resolve problems. Here are some common issues and tips on how to troubleshoot them:
-
Peripheral not found: If your app is unable to find a peripheral, ensure that the peripheral is powered on and within range. Also, check if the correct UUIDs are being used in the scanForPeripherals(withServices:options:) method.
let services = [CBUUID(string: "YOUR_SERVICE_UUID")] centralManager.scanForPeripherals(withServices: services, options: nil)
-
Connection issues: If you’re facing problems connecting to a peripheral, verify that you are not already connected to it. Also, check for any error messages provided in the centralManager(_:didFailToConnect:error:) delegate method.
func centralManager(_ central: CBCentralManager, didFailToConnect peripheral: CBPeripheral, error: Error?) { if let error = error { print("Failed to connect to peripheral: (error.localizedDescription)") } }
-
Services or characteristics not found: If the required services or characteristics are not found, make sure that they’re properly advertised by the peripheral and that you have called the discoverServices: and discoverCharacteristics:forService: methods.
peripheral.discoverServices([CBUUID(string: "YOUR_SERVICE_UUID")]) peripheral.discoverCharacteristics([CBUUID(string: "YOUR_CHARACTERISTIC_UUID")], for: service)
-
Receiving incomplete data: BLE devices send data in packets. If you are receiving incomplete data, ensure that you’re properly reassembling the packets in the didUpdateValueFor characteristic delegate method.
func peripheral(_ peripheral: CBPeripheral, didUpdateValueFor characteristic: CBCharacteristic, error: Error?) { if let value = characteristic.value { // Process the received data } }
-
Unexpected disconnections: If the peripheral is disconnecting unexpectedly, check for any error messages in the centralManager(_:didDisconnectPeripheral:error:) delegate method and ensure that the peripheral is not going out of range or powering off.
func centralManager(_ central: CBCentralManager, didDisconnectPeripheral peripheral: CBPeripheral, error: Error?) { if let error = error { print("Peripheral disconnected with error: (error.localizedDescription)") } }
By keeping an eye on these common issues and using the provided code examples, developers can effectively troubleshoot and resolve problems encountered during CoreBluetooth integration. Remember to utilize console logs and error messages to gain insights into what might be going wrong. With patience and persistence, most issues can be addressed allowing for a smooth Bluetooth experience in your Swift applications.