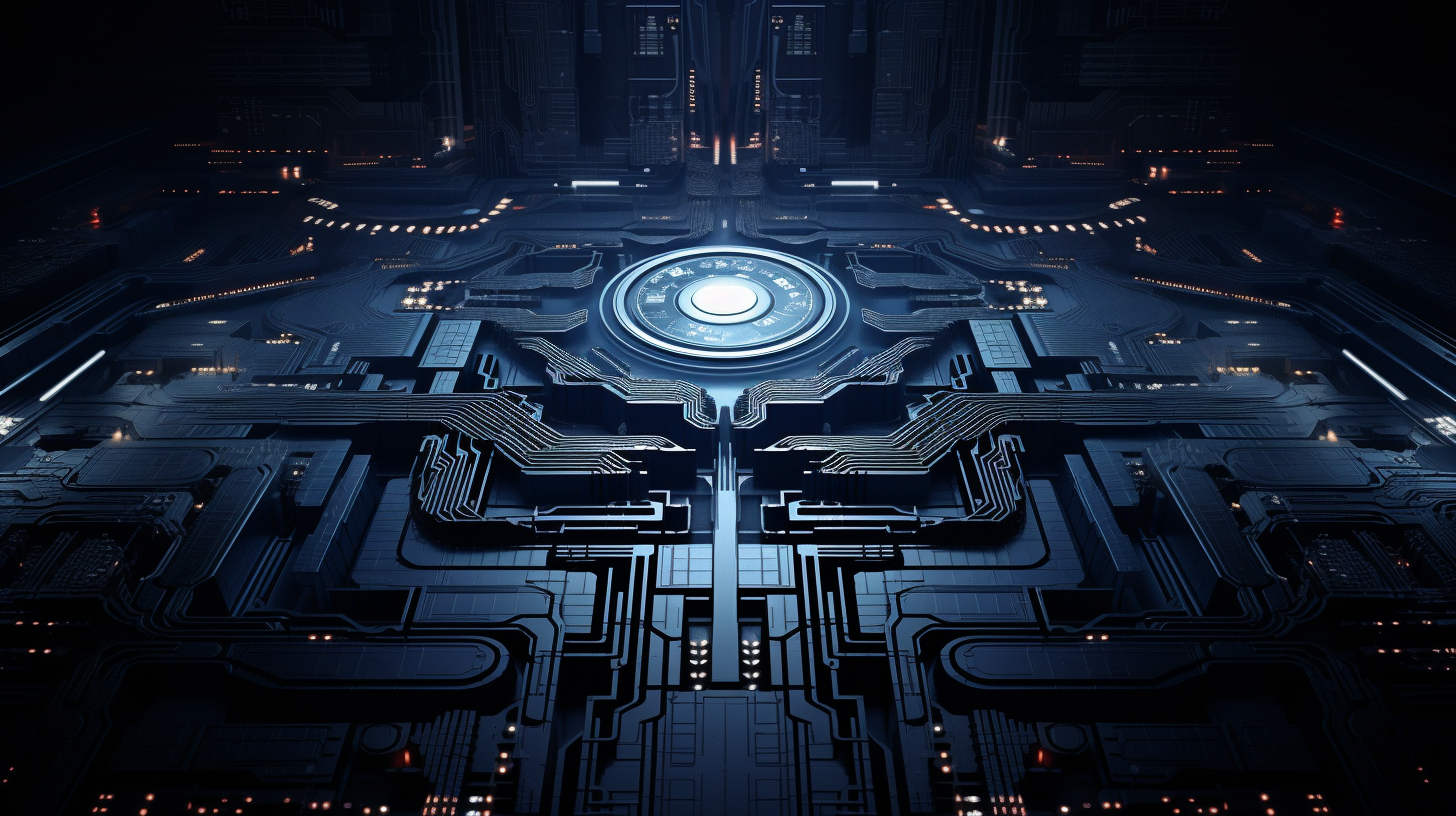
Understanding JavaScript Scopes and Contexts
Understanding the concepts of scope and context in JavaScript is important for writing efficient and bug-free code. In this tutorial, we will delve deep into these concepts, understand how they work and how to use them effectively in your JavaScript programs.
What is Scope?
Scope in JavaScript refers to the accessibility of variables, functions, and objects in some particular part of your code during runtime. In other words, it determines the visibility of variables and functions at various parts of your code. There are two types of scopes in JavaScript:
- Variables declared outside any function or block have a Global scope, which means they can be accessed and modified from any part of the code.
- Variables declared within a function or block have a Local scope, which means they can only be accessed and modified within that function or block.
What is Context?
Context in JavaScript refers to the value of this
keyword in a given part of your code. The value of this
depends on how the function is called. It can refer to the global object, the object that owns the method, or the object that’s created by a constructor.
Let’s see some code examples to understand these concepts better:
// Global scope example var globalVar = 'Hello, World!'; function display() { console.log(globalVar); // Output: Hello, World! } display();
In the above example, globalVar
is declared in the Global scope and is accessible inside the display()
function.
// Local scope example function greet() { var localVar = 'Hello, Local!'; console.log(localVar); // Output: Hello, Local! } greet(); console.log(localVar); // Uncaught ReferenceError: localVar is not defined
In this example, localVar
is declared within the greet()
function and has a Local scope. It cannot be accessed outside the function.
// Context example using method var obj = { value: 'Hello, Context!', showValue: function() { console.log(this.value); // Output: Hello, Context! } }; obj.showValue();
In the context example above, this
inside the showValue()
method refers to the object obj
that owns the method.
To summarize, understanding scope and context in JavaScript will help you manage variables and functions efficiently. Remember that scope deals with the visibility of variables while context deals with the value of this
. With these concepts clear in your mind, you’ll be better equipped to write clean and maintainable JavaScript code.