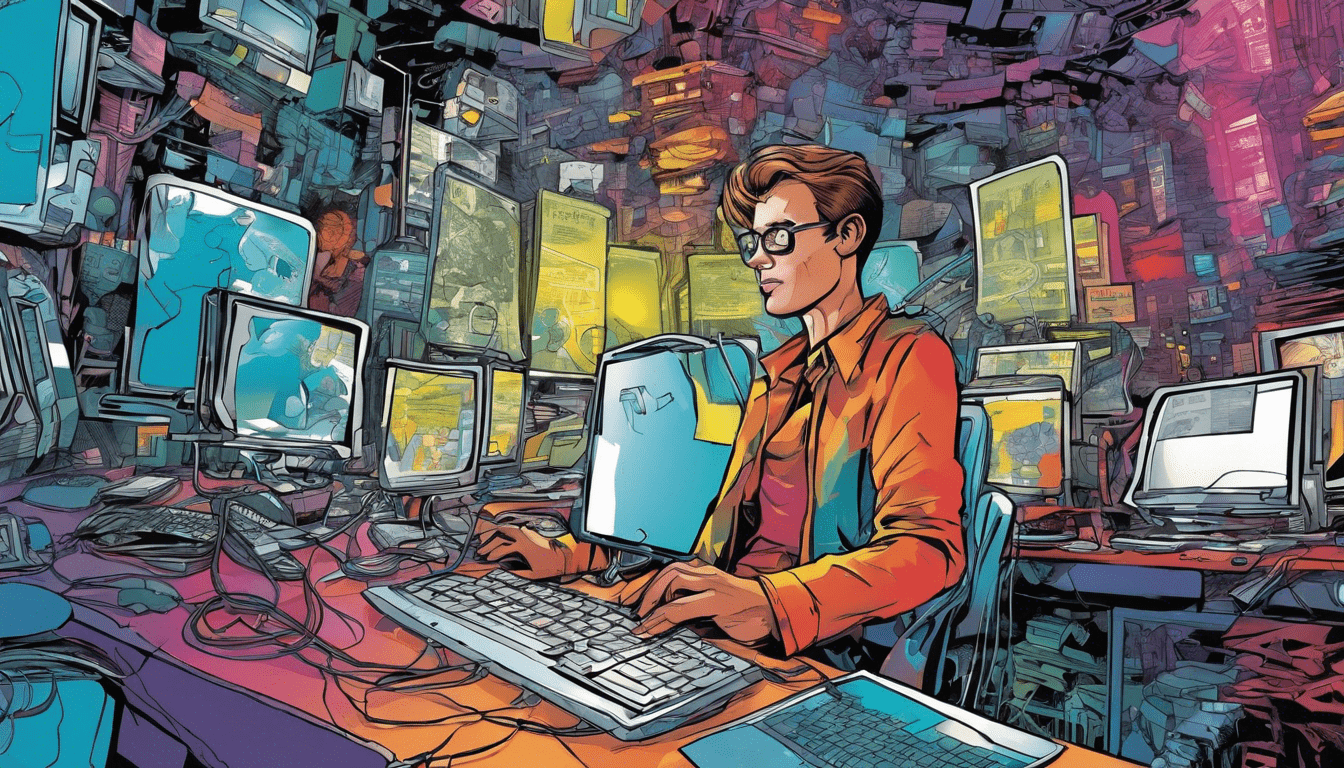
Concurrency in Swift
Concurrency in Swift is a programming concept that allows multiple tasks to run simultaneously within a single program. This can improve the performance and responsiveness of an application, especially when dealing with long-running tasks such as networking or heavy computations. In this article, we will delve into the idea of concurrency in Swift and provide code examples to show how it can be implemented.
In Swift, concurrency is achieved through the use of Async and Await keywords, as well as Threads. The Async/Await pattern allows for asynchronous programming, where a task can be started and then the program can move on to other tasks while waiting for the first task to complete. Threads, on the other hand, allow for multiple tasks to run concurrently on different cores of the CPU.
Let’s start with an example of how to use Async/Await in Swift:
func fetchData() async { let url = URL(string: "https://api.example.com/data")! let (data, _) = try! await URLSession.shared.data(from: url) print("Data fetched: (data)") } Task { await fetchData() }
In the above code, we define a function fetchData
that fetches data from an API. The async
keyword before the function indicates that it’s an asynchronous function. Inside the function, we use await
before calling URLSession.shared.data
, which is an asynchronous function that fetches data from the specified URL. By using await
, we’re telling Swift to suspend the execution of fetchData
until the data is fetched. When the data is fetched, the execution resumes and we print the data.
We then call fetchData
inside a Task
, which is a concurrency primitive in Swift that allows us to run asynchronous code. By using Task
, we’re telling Swift to run fetchData
simultaneously with other tasks.
Now let’s look at an example of using Threads in Swift:
import Foundation func doWorkOnBackgroundThread() { DispatchQueue.global().async { print("Doing work on background thread") // Perform long-running task here } } doWorkOnBackgroundThread() print("Doing work on main thread")
In this example, we define a function doWorkOnBackgroundThread
that performs a long-running task on a background thread. We use DispatchQueue.global().async
to run the code inside a closure asynchronously on a global background queue. By doing this, we’re allowing the long-running task to run on a different thread than the main thread, which prevents the main thread from being blocked and allows the app to remain responsive.
We then call doWorkOnBackgroundThread
and immediately after that, we print a message indicating that we’re doing work on the main thread. Because doWorkOnBackgroundThread
runs asynchronously on a background thread, the message will be printed immediately, without waiting for the long-running task to complete.
In conclusion, concurrency in Swift allows us to write more efficient and responsive applications by running tasks simultaneously. By using Async/Await and Threads, we can perform long-running tasks without blocking the main thread and keep our app responsive to user input. With practice and understanding of these concepts, you’ll be able to take advantage of concurrency in your Swift applications.