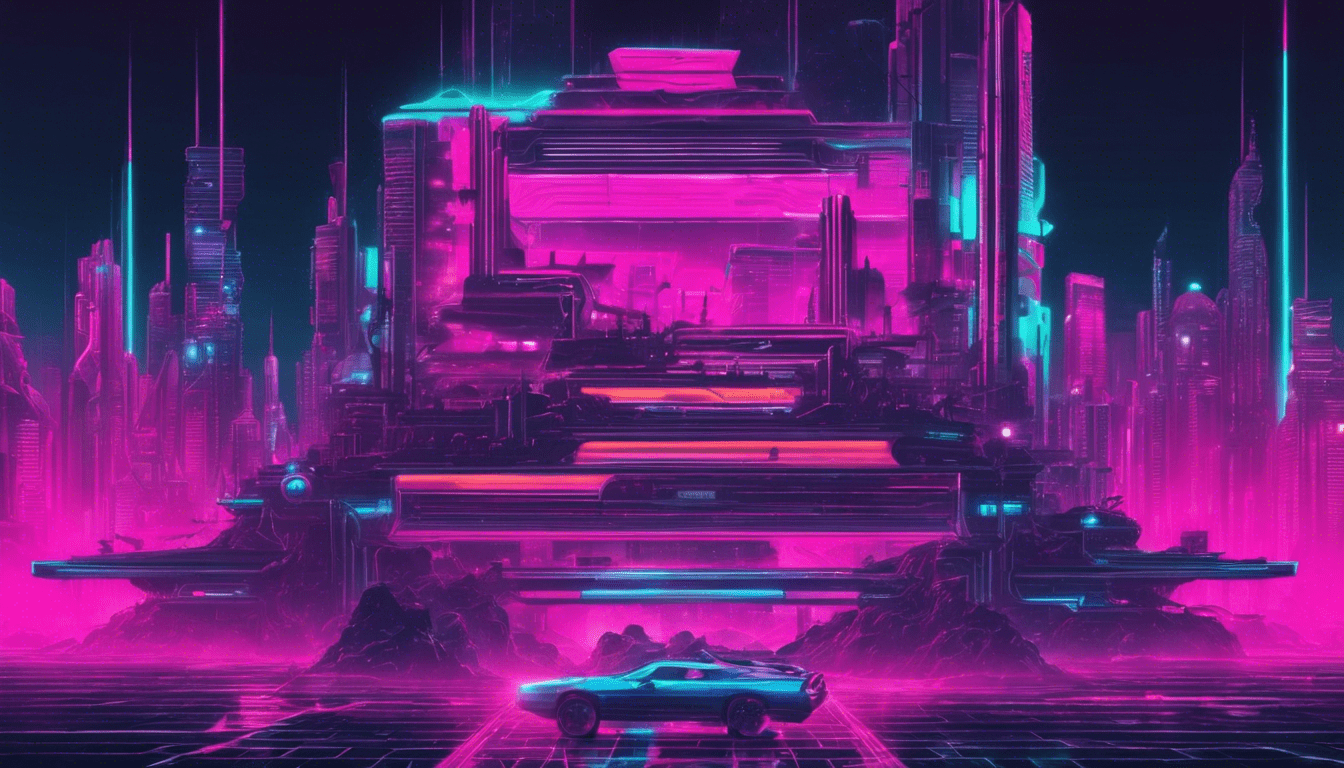
PHP and AJAX: Dynamic Web Pages
PHP and AJAX are two powerful technologies that can be used to create dynamic web pages. AJAX, which stands for Asynchronous JavaScript and XML, allows web pages to be updated asynchronously by exchanging data with a server behind the scenes. This means that it is possible to update parts of a web page without reloading the whole page.
PHP is a server-side scripting language that’s used to create dynamic web content. When combined with AJAX, PHP can be used to process and respond to requests made by the AJAX function in real time, making the web page more interactive and responsive.
To use AJAX with PHP, you need to use the XMLHttpRequest object, which is a JavaScript object that allows you to make HTTP requests to the server without reloading the page. Here is an example of how to use AJAX with PHP:
<script> function loadDoc() { var xhttp = new XMLHttpRequest(); xhttp.onreadystatechange = function() { if (this.readyState == 4 && this.status == 200) { document.getElementById("demo").innerHTML = this.responseText; } }; xhttp.open("GET", "ajax_info.php", true); xhttp.send(); } </script>
In this example, the loadDoc function is called when a button is clicked. The function creates a new XMLHttpRequest object and sends a GET request to the server. The server then processes the request using a PHP script, which might look something like this:
<?php echo "Hello, World!"; ?>
When the server responds, the onreadystatechange event is triggered, and if the readyState is 4 (request finished and response is ready) and the status is 200 (OK), then the innerHTML of the element with the id “demo” is updated with the response from the server.
Using AJAX with PHP allows you to create web pages that are more interactive and responsive, without the need for the whole page to be reloaded. This can lead to a better user experience and more efficient use of server resources.
To further enhance your dynamic web pages, you can also use AJAX to send data to the server. Here is an example of how to send form data to a PHP script using AJAX:
<script> function submitForm() { var xhttp = new XMLHttpRequest(); var formData = new FormData(document.getElementById("myForm")); xhttp.onreadystatechange = function() { if (this.readyState == 4 && this.status == 200) { document.getElementById("demo").innerHTML = this.responseText; } }; xhttp.open("POST", "form_processor.php", true); xhttp.send(formData); } </script> <form id="myForm"> Name: <input type="text" name="name"><br> Email: <input type="text" name="email"><br> <input type="button" value="Submit" onclick="submitForm()"> </form> <div id="demo"></div>
In this example, the submitForm function is called when the submit button is clicked. The function creates a new XMLHttpRequest object and a FormData object, which contains the data from the form. The XMLHttpRequest object is then used to send the form data to a PHP script on the server, which might look something like this:
<?php $name = $_POST['name']; $email = $_POST['email']; echo "Name: ". $name ."<br>"; echo "Email: ". $email; ?>
When the server responds, the onreadystatechange event is triggered and if the readyState is 4 and the status is 200, then the innerHTML of the element with the id “demo” is updated with the response from the server.
To wrap it up, using PHP with AJAX enables you to create dynamic web pages that are more interactive and responsive. With a little bit of JavaScript and PHP knowledge, you can start creating web applications that provide a better user experience.