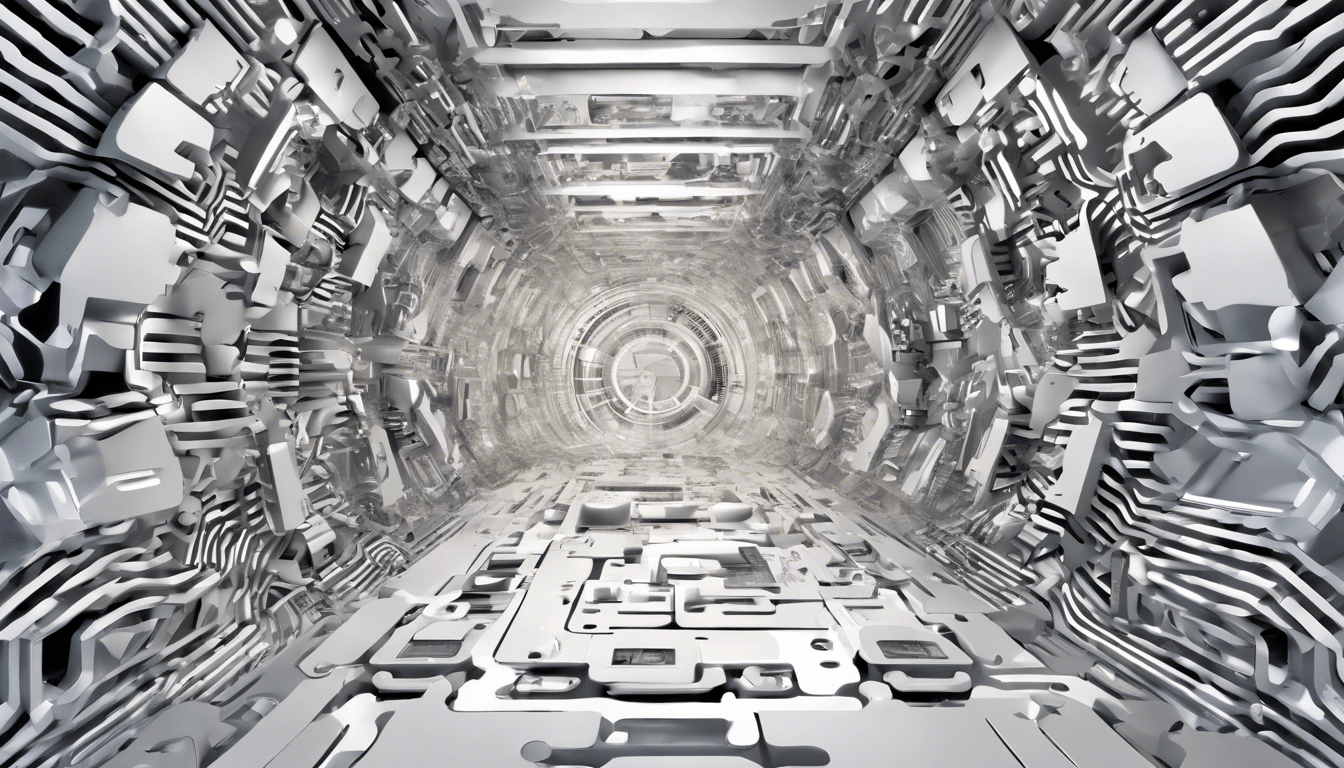
Python and Internet of Things (IoT): Basics
The Internet of Things (IoT) is a network of interconnected physical devices that can communicate and exchange data with each other. Python is widely used in IoT projects due to its simplicity and versatility. In this article, we will discuss the basics of using Python in IoT, with a focus on Raspberry Pi and sensor integration.
Raspberry Pi and IoT
Raspberry Pi is a popular single-board computer that can be used as a central hub for IoT projects. It provides GPIO (General Purpose Input/Output) pins that allow you to connect sensors and actuators directly to the board. Python is the recommended programming language for Raspberry Pi due to its extensive library support and ease of use.
Connecting Sensors to Raspberry Pi
To connect sensors to Raspberry Pi, you need to identify the type of sensor and the method of communication it uses. Common sensor types include temperature, humidity, light, motion, and proximity sensors.
Most sensors communicate via protocols such as I2C, SPI, or UART (Serial). Python libraries exist for each of these protocols, making it simple to interface with the sensors. For example, if you have a temperature and humidity sensor that communicates over I2C, you can use the smbus
library to read data from the sensor.
import smbus bus = smbus.SMBus(1) address = 0x40 def read_temperature(): data = bus.read_i2c_block_data(address, 0xE7, 2) temperature = (data[0]In the above example, we import the
Interacting with Actuatorssmbus
library and initialize the I2C bus. We then define functions to read the temperature and humidity from the sensor by sending appropriate commands and reading the data. Finally, we print the values to the console.An actuator is a device that receives a signal from the Raspberry Pi and performs a physical action. Examples include LED lights, motors, relays, and servo motors.
Similar to sensors, actuators can be controlled using various protocols such as GPIO, I2C, SPI, or UART. Python libraries exist for each protocol, which will allow you to control the actuators using simple code. Let's take an example of controlling an LED using GPIO.
import RPi.GPIO as GPIO import time led_pin = 18 GPIO.setmode(GPIO.BCM) GPIO.setup(led_pin, GPIO.OUT) def blink_led(): for _ in range(5): GPIO.output(led_pin, GPIO.HIGH) time.sleep(1) GPIO.output(led_pin, GPIO.LOW) time.sleep(1) blink_led() GPIO.cleanup()In this example, we import the
RPi.GPIO
library and initialize the GPIO mode. We then set up the LED pin as an output pin. Theblink_led()
function turns the LED on for 1 second and off for 1 second, repeating the process 5 times. Finally, we clean up the GPIO pins to ensure they can be used by other programs.Processing Sensor Data
Once you have read data from sensors, you can perform data processing and analysis using Python. This can range from simple calculations to complex machine learning algorithms. Python provides a rich set of libraries for data analysis and processing, such as NumPy, Pandas, and Matplotlib.
For example, let's say we want to visualize the temperature and humidity data collected from a sensor over time. We can use the Matplotlib library to plot the data on a graph.
import matplotlib.pyplot as plt # Assuming temperature and humidity are collected in lists temperature_data = [25, 26, 27, 28, 29] humidity_data = [40, 45, 42, 47, 50] plt.plot(temperature_data, label='Temperature (°C)') plt.plot(humidity_data, label='Humidity (%)') plt.xlabel('Time') plt.ylabel('Value') plt.title('Temperature and Humidity Data') plt.legend() plt.show()In this example, we import the
matplotlib.pyplot
library and create two lists containing temperature and humidity data. We then use theplot()
function to plot the data on a graph. Thexlabel()
,ylabel()
,title()
, andlegend()
functions are used to add labels and a legend to the graph. Finally, we use theshow()
function to display the graph.Python is a powerful language for developing IoT applications. Its simplicity, extensive library support, and compatibility with Raspberry Pi make it an ideal choice for beginners. By using Python, you can easily connect sensors and actuators to Raspberry Pi, process sensor data, and control physical devices. With the increasing popularity of IoT, learning Python and its integration with IoT is a valuable skill for aspiring programmers.