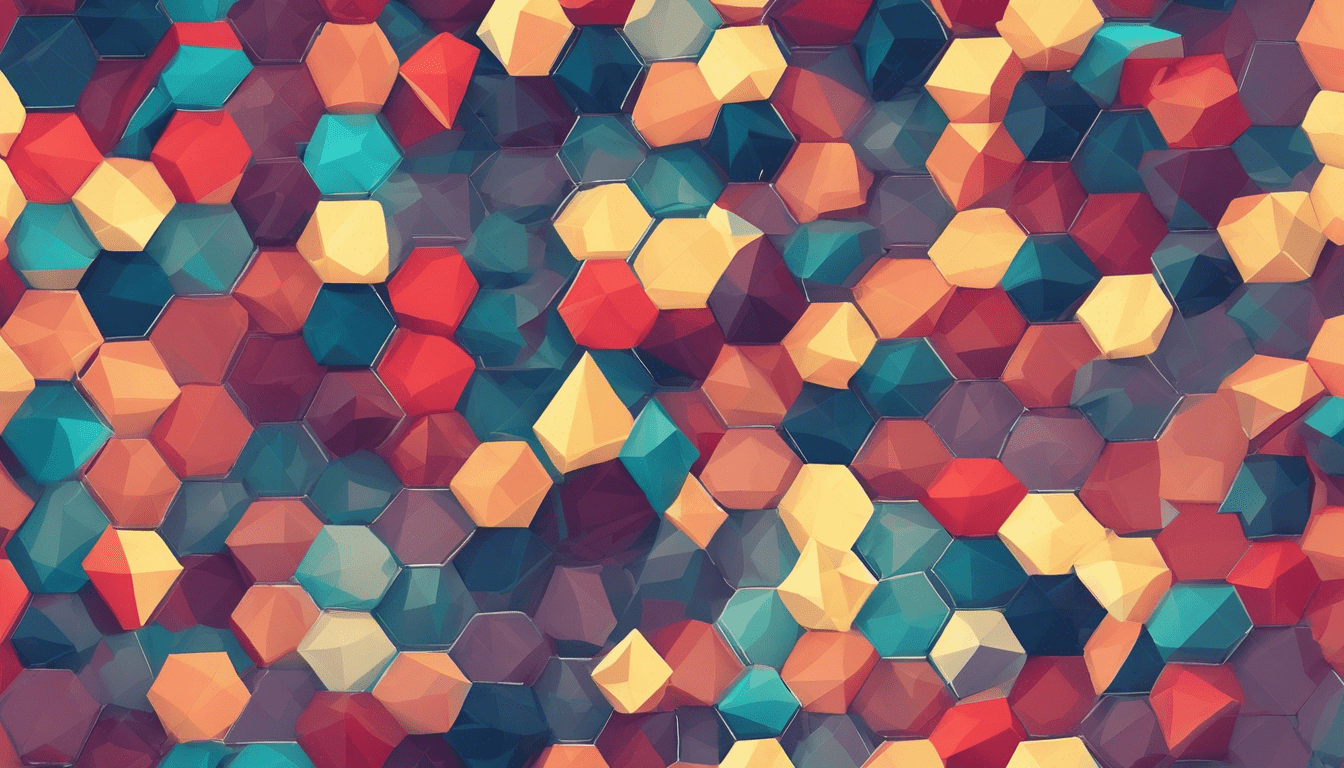
SQL for User Authentication and Authorization
When it comes to managing user access to a system or application, two important concepts come into play: authentication and authorization. In SQL, both of these processes can be managed effectively with a combination of SQL queries and best practices. Let’s explore how this works.
Authentication is the process of verifying the identity of a user. This ensures that the person attempting to gain access to a system is who they say they are. Authentication is usually performed using a username and password, but can also incorporate other methods such as biometrics or security tokens.
Authorization, on the other hand, is about permissions. Once a user’s identity has been verified, authorization determines what resources the user has access to and what actions they’re allowed to perform. In terms of SQL, this could mean which databases, tables or rows a user can read or write to.
To handle user authentication in SQL, you create tables that store user information and credentials. Here’s a simple example of what a users table could look like:
CREATE TABLE users ( id INT AUTO_INCREMENT PRIMARY KEY, username VARCHAR(50) UNIQUE NOT NULL, password VARCHAR(50) NOT NULL, email VARCHAR(100) UNIQUE NOT NULL, created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP );
To add a new user, you would insert their information into the users table:
INSERT INTO users (username, password, email) VALUES ('johndoe', 'password123', '[email protected]');
When a user tries to log in, you check if the provided credentials match a record in the database:
SELECT * FROM users WHERE username = 'johndoe' AND password = 'password123';
If the query returns a record, the authentication process is successful. Otherwise, the login is denied. However, storing passwords in plain text is not secure. Instead, passwords should be hashed before they’re stored in the database, and compared in their hashed form during the login process.
Once a user is authenticated, you need to handle authorization. Within SQL, this can be achieved by assigning roles to users and then granting permissions to those roles.
First, you would create a table for roles:
CREATE TABLE roles ( id INT AUTO_INCREMENT PRIMARY KEY, name VARCHAR(50) UNIQUE NOT NULL );
Then you would create a table to link users and roles:
CREATE TABLE user_roles ( user_id INT NOT NULL, role_id INT NOT NULL, FOREIGN KEY (user_id) REFERENCES users(id), FOREIGN KEY (role_id) REFERENCES roles(id), PRIMARY KEY (user_id, role_id) );
Add roles and assign them to users as necessary:
INSERT INTO roles (name) VALUES ('admin'), ('editor'), ('viewer'); INSERT INTO user_roles (user_id, role_id) VALUES (1, 1); -- Assuming 1 is the id of johndoe
And finally, grant permissions to roles on specific SQL operations:
GRANT SELECT, INSERT, UPDATE ON databasename.tablename TO 'admin'; GRANT SELECT ON databasename.tablename TO 'viewer';
This way, you control who has access to what data and what they can do with it. Remember that user authentication and authorization are critical components of SQL database security. Implementing proper checks controls access and helps safeguard against unauthorized use or breaches.