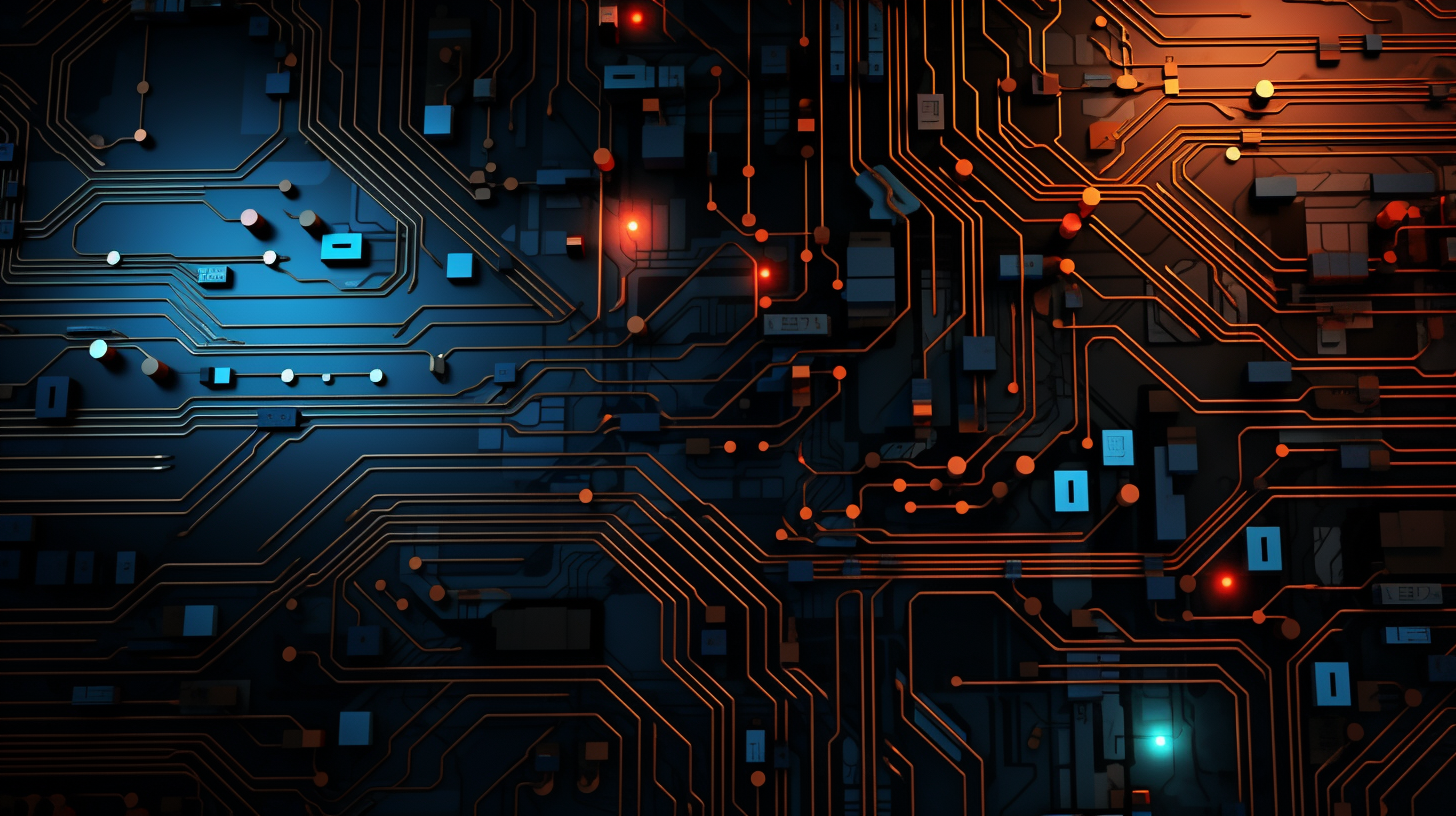
PHP and Image Processing
Introduction to PHP and Image Processing
PHP, a server-side scripting language, has become a powerful tool for web development and is widely used to create dynamic web pages. Among its many capabilities, PHP also offers functionalities for image processing, which is the method of performing operations on images to enhance them or extract information.
Image processing in PHP can be achieved with the help of the GD library, which is an open-source code library for the dynamic creation of images. PHP’s GD library allows for image creation and manipulation, including tasks such as resizing, cropping, rotating, and filtering images.
To begin working with image processing in PHP, you first need to ensure that the GD library is installed and enabled on your server. You can check this by creating a PHP file with the following code:
<?php phpinfo(); ?>
Once you have confirmed that GD library is enabled, you can start processing images. For example, to create a simple image with a rectangle drawn on it, you would use the following code:
<?php // Create a 100*100 image $im = imagecreatetruecolor(100, 100); // Allocate colors $white = imagecolorallocate($im, 255, 255, 255); $red = imagecolorallocate($im, 255, 0, 0); // Draw a red rectangle imagerectangle($im, 10, 10, 90, 90, $red); // Save the image imagepng($im, 'rectangle.png'); imagedestroy($im); ?>
That is just a basic example of what can be done with PHP’s image processing capabilities. The GD library provides a wide range of functions for more complex tasks such as creating thumbnails, generating images from text, applying filters and effects, and much more.
With PHP and image processing, developers can build applications that handle media-rich content effectively. Whether it is for creating CAPTCHA images, resizing user-uploaded photos or generating charts and graphs, PHP’s image processing features are robust and versatile, making it an invaluable skill for any web developer.
Image Manipulation Techniques in PHP
Image manipulation in PHP is not only powerful but also relatively straightforward. With the GD library, developers have a suite of tools at their disposal to perform various image manipulation techniques. Here are some common techniques you can use to manipulate images in PHP:
- Changing the dimensions of an image is one of the most common operations in image processing. PHP’s GD library provides the imagecopyresampled() function to help with this. Here’s an example of how to resize an image:
<?php // Load the image $source = imagecreatefromjpeg('source.jpg'); // Get the original dimensions list($width, $height) = getimagesize('source.jpg'); // Set the new dimensions $new_width = 200; $new_height = 150; // Create a new true color image $thumb = imagecreatetruecolor($new_width, $new_height); // Copy and resize the old image into the new image imagecopyresampled($thumb, $source, 0, 0, 0, 0, $new_width, $new_height, $width, $height); // Save the resized image imagejpeg($thumb, 'thumbnail.jpg'); // Clean up imagedestroy($source); imagedestroy($thumb); ?>
- To crop an image, you can use the imagecrop() function. This enables you to specify a rectangular portion of the source image to retain while discarding the rest:
<?php // Load the image $source = imagecreatefromjpeg('source.jpg'); // Define the crop rectangle $crop_rectangle = ['x' => 50, 'y' => 50, 'width' => 200, 'height' => 200]; // Crop image $cropped_image = imagecrop($source, $crop_rectangle); // Save the cropped image imagejpeg($cropped_image, 'cropped.jpg'); // Clean up imagedestroy($source); imagedestroy($cropped_image); ?>
- To rotate an image, PHP offers the imagerotate() function. You can specify the rotation angle and the background color that will fill any exposed areas after rotation:
<?php // Load the image $source = imagecreatefromjpeg('source.jpg'); // Rotate the image $rotated_image = imagerotate($source, 90, 0); // Save the rotated image imagejpeg($rotated_image, 'rotated.jpg'); // Clean up imagedestroy($source); imagedestroy($rotated_image); ?>
- PHP’s GD library comes with a range of filters that can be applied to images for effects like grayscale, brightness adjustment, contrast adjustment, etc. The imagefilter() function is used for this purpose:
<?php // Load the image $source = imagecreatefromjpeg('source.jpg'); // Apply grayscale filter imagefilter($source, IMG_FILTER_GRAYSCALE); // Save the filtered image imagejpeg($source, 'grayscale.jpg'); // Clean up imagedestroy($source); ?>
These are just a few examples of what you can achieve with PHP’s image manipulation capabilities. By combining these techniques, you can perform complex operations and create dynamic images that enhance your web applications.
Advanced Image Processing Features in PHP
Advanced image processing features in PHP allow developers to take their image manipulation skills to the next level. These features include creating complex shapes, handling transparency, working with text, and applying advanced filters. Let’s delve into some of these advanced features:
- While the GD library allows for basic shapes like rectangles and ellipses, you can also create complex polygons using the imagefilledpolygon() function. Here’s an example:
<?php // Create a 200*200 image $im = imagecreatetruecolor(200, 200); // Allocate colors $white = imagecolorallocate($im, 255, 255, 255); $blue = imagecolorallocate($im, 0, 0, 255); // Define points for the polygon $values = array( 50, 50, // Point 1 (x, y) 150, 50, // Point 2 (x, y) 100, 200 // Point 3 (x, y) ); // Draw a blue polygon imagefilledpolygon($im, $values, 3, $blue); // Save the image imagepng($im, 'polygon.png'); imagedestroy($im); ?>
- To work with image transparency in PHP, you can use the imagesavealpha() and imagealphablending() functions. This is particularly useful when working with PNG images that have transparent backgrounds. Here’s how you can preserve transparency when resizing a PNG image:
<?php // Load the image $source = imagecreatefrompng('source.png'); // Enable alpha blending and save alpha imagealphablending($source, false); imagesavealpha($source, true); // Rest of the resizing code as shown in the previous example // ... ?>
- PHP’s GD library enables you to add text to images using imagettftext(). This function gives you control over font size, angle, and color. Here’s how to add text to an image:
<?php // Create a 200*100 image $im = imagecreatetruecolor(200, 100); // Allocate colors $white = imagecolorallocate($im, 255, 255, 255); $black = imagecolorallocate($im, 0, 0, 0); // Set the path to the font file $font_path = 'arial.ttf'; // Add the text imagettftext($im, 20, 0, 10, 50, $black, $font_path, 'Hello World!'); // Save the image imagepng($im, 'text_image.png'); imagedestroy($im); ?>
- Beyond basic filters such as grayscale or brightness adjustment, PHP also allows for custom image filters using imagefilter(). For example, you can create a pixelate effect using the IMG_FILTER_PIXELATE filter. Here’s an example:
<?php // Load the image $source = imagecreatefromjpeg('source.jpg'); // Apply pixelate filter imagefilter($source, IMG_FILTER_PIXELATE, 10, true); // Save the filtered image imagejpeg($source, 'pixelated.jpg'); // Clean up imagedestroy($source); ?>
By mastering these advanced features of PHP’s GD library, you can create more sophisticated and visually appealing images for your web applications. Whether it is generating custom graphics on-the-fly or processing user-uploaded photos with advanced effects, these tools provide a rich set of capabilities for PHP developers.
Best Practices for Efficient Image Processing in PHP
Efficient image processing in PHP requires adhering to best practices that can optimize performance and reduce resource consumption. Here are some important tips to keep in mind while working with images:
- Selecting the appropriate image format is important for both quality and performance. For example, use JPEG for photographs, PNG for images with transparency, and GIF for simple animations.
- Working with smaller images can significantly speed up processing time. Always resize your images to the smallest possible size that still meets your requirements before applying any further processing.
- After you’re done processing an image, use the imagedestroy() function to free up memory. That’s especially important in scripts that process multiple images or large files.
- If you have multiple images to process, try to batch them in a single script run. This can be more efficient than processing each image in a separate request.
- Cache the results of image processing to avoid redoing the same operations. Use unique filenames or a caching mechanism to serve processed images without reprocessing them.
- Write clean and efficient PHP code. Avoid unnecessary computations, and use built-in functions where possible as they’re usually optimized for performance.
Here is an example of optimizing image processing by resizing an image before applying filters:
<?php // Load the image $source = imagecreatefromjpeg('source.jpg'); // Resize the image first list($width, $height) = getimagesize('source.jpg'); $new_width = $width * 0.5; $new_height = $height * 0.5; $resized = imagecreatetruecolor($new_width, $new_height); imagecopyresampled($resized, $source, 0, 0, 0, 0, $new_width, $new_height, $width, $height); // Apply filters to the resized image imagefilter($resized, IMG_FILTER_GRAYSCALE); // Save the processed image imagejpeg($resized, 'processed.jpg'); // Clean up imagedestroy($source); imagedestroy($resized); ?>
By following these best practices, you can ensure that your PHP-based image processing is as efficient and effective as possible.