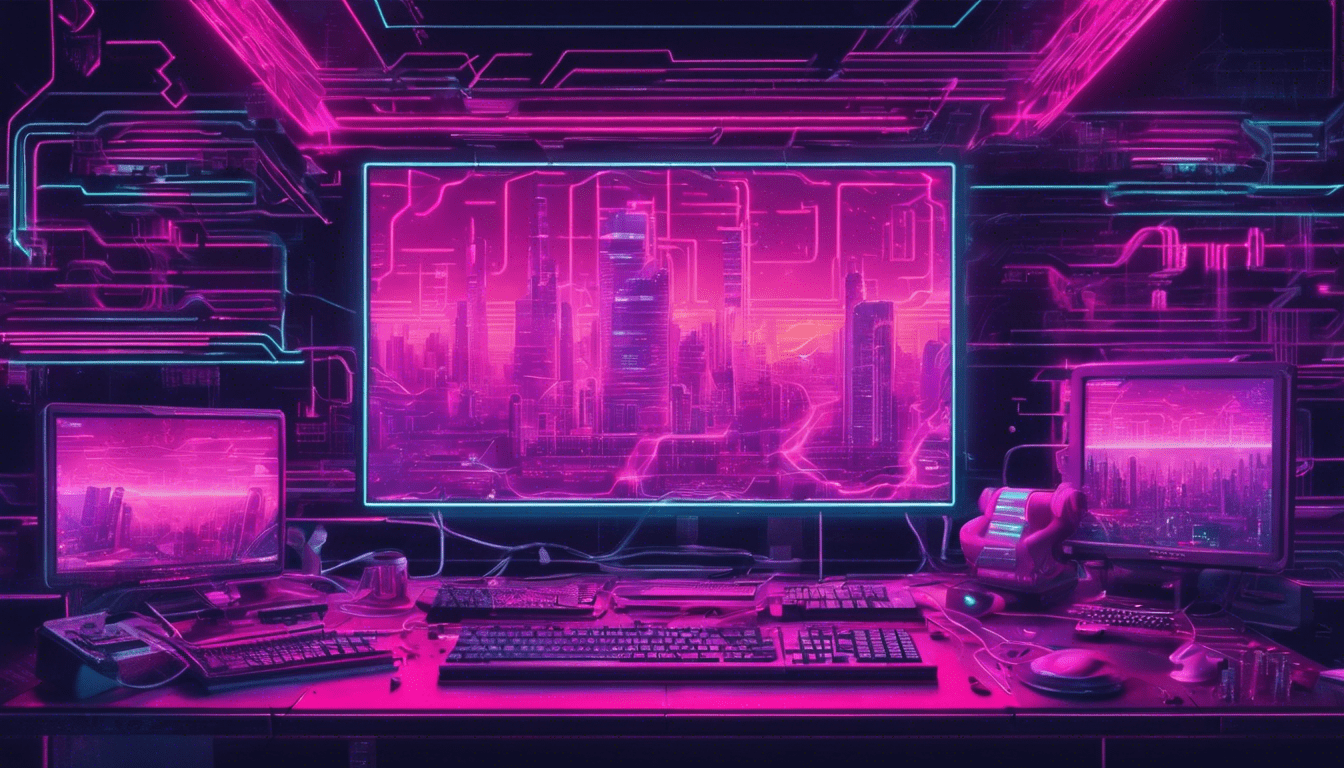
JavaScript Document Object Model (DOM) Manipulation
JavaScript Document Object Model (DOM) Manipulation is an essential concept to grasp for anyone looking to build interactive websites. The DOM is a representation of the HTML document as a tree structure, where each element is a node. JavaScript can be used to manipulate these nodes, which will allow you to change the content, structure, and style of your webpage in real time.
When a web page is loaded, the browser creates a DOM of the page. With DOM manipulation, we can do things like change the text inside an element, add or remove classes, or even create new elements entirely.
The first step in DOM manipulation is selecting the element or elements you want to manipulate. You can do this by using various methods such as document.getElementById()
, document.getElementsByClassName()
, document.getElementsByTagName()
, or document.querySelector()
.
var element = document.getElementById('myElement');
Once you have selected an element, you can manipulate it in various ways. For example, you can change the content using innerHTML
or textContent
:
element.textContent = 'New text content!';
You can also change the HTML structure using appendChild()
, removeChild()
, or replaceChild()
:
var newElement = document.createElement('div'); newElement.textContent = 'I am a new element!'; element.appendChild(newElement);
Another way to manipulate an element is by changing its style. This can be done by accessing the style
property:
element.style.color = 'blue'; element.style.fontSize = '20px';
Beyond modifying individual elements, you can also manipulate their attributes using methods like setAttribute()
and getAttribute()
:
element.setAttribute('class', 'myClass'); var attributeValue = element.getAttribute('class');
Events are another key part of DOM manipulation. By adding event listeners to elements, you can run code in response to user actions like clicks, keyboard input, and more:
element.addEventListener('click', function() { alert('Element clicked!'); });
To wrap it up, DOM manipulation with JavaScript provides a powerful way to build dynamic web pages. By understanding how to select elements and use methods to manipulate them, you can start creating interactive and dynamic user experiences on your website.
One important aspect of DOM manipulation that I think should be mentioned is the concept of “event delegation.” This technique allows you to attach a single event listener to a parent element, rather than individual listeners on multiple child elements. This not only improves performance but also makes your code more manageable and scalable. Additionally, when working with dynamic content that may change or be added to the page after the initial load, event delegation can ensure that event listeners are still effective. Overall, understanding event delegation very important for efficient and effective DOM manipulation.