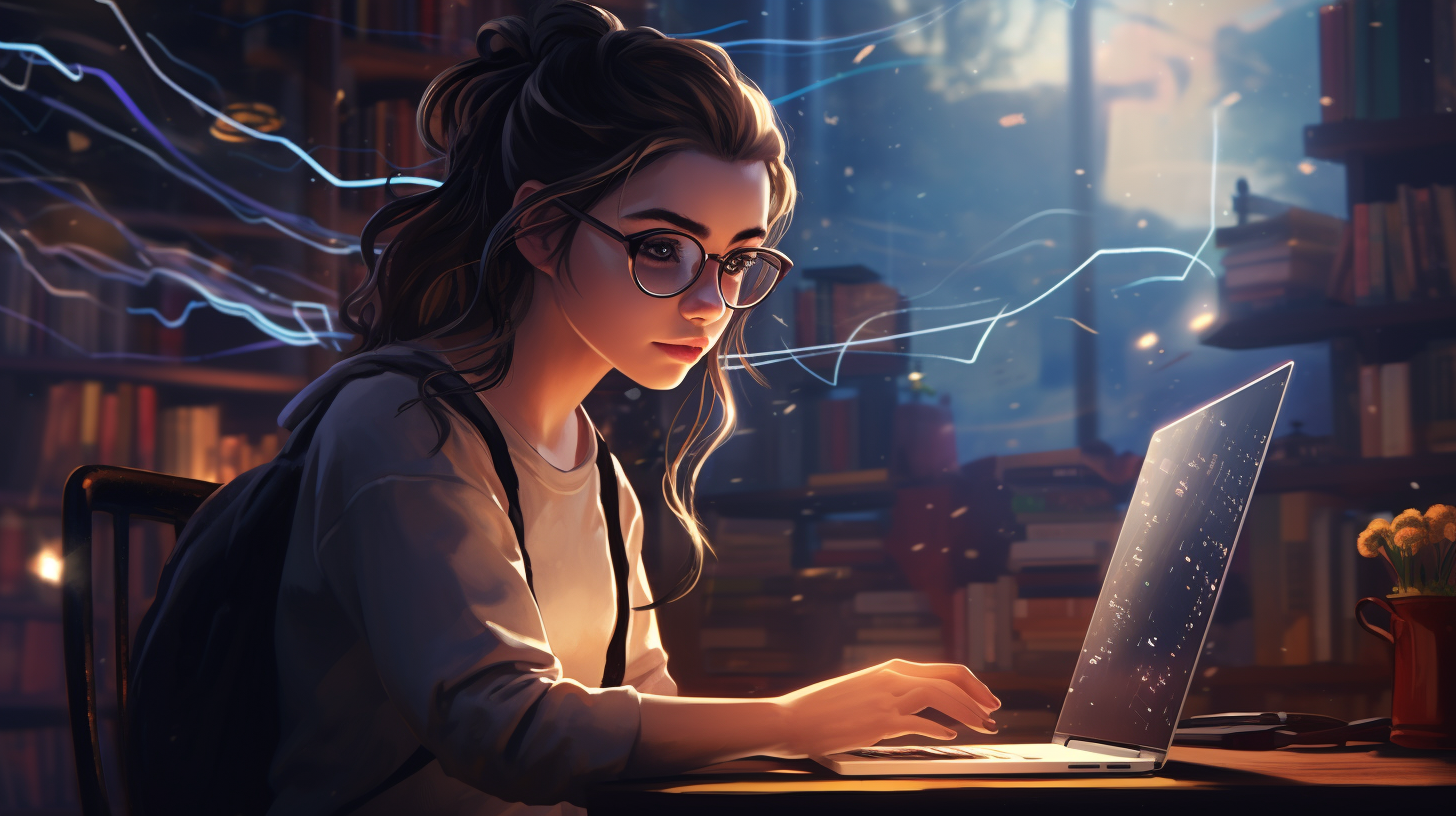
SQL Strategies for Data Optimization
SQL is a powerful tool for managing and manipulating data in databases. One crucial aspect of working with SQL is data optimization. Optimizing your data can lead to improved performance, faster query times, and a more efficient database overall. In this tutorial, we will discuss several strategies for optimizing your data using SQL.
Indexing
One of the most effective strategies for optimizing data in SQL is indexing. Indexes are special lookup tables that the database search engine can use to speed up data retrieval. Creating an index on a table column can drastically reduce the amount of time it takes to query that column. Here’s how you can create an index in SQL:
CREATE INDEX idx_column_name ON table_name(column_name);
It’s important to note that while indexes can speed up data retrieval, they can also slow down data insertion and updates, as the index must be maintained. Therefore, it is essential to use indexes judiciously and update them as necessary.
Query Optimization
Writing efficient queries is another critical aspect of SQL data optimization. Here are a few tips for optimizing your queries:
- Avoid using
SELECT *
; instead, specify the exact columns you need. - Make use of the
WHERE
clause to filter data and reduce the size of the result set. - Use
JOIN
statements wisely, and avoid unnecessary joins that can slow down the query. - Make use of aggregate functions like
COUNT
,SUM
,AVG
to summarize data instead of fetching all details.
For example, instead of writing:
SELECT * FROM employees;
Write:
SELECT id, name, department FROM employees WHERE department='Sales';
Normalization
Normalizing your database is an essential strategy for optimizing data. Normalization involves organizing your tables and their relationships in a way that reduces redundancy and dependency. Proper normalization can lead to a more efficient database structure, making query processing faster and more reliable. Here’s a simple example of normalization:
Before normalization, you might have a table
orders
with columnsorder_id
,customer_name
,product_name
, andproduct_price
. After normalization, you’d separate this into two tables:orders
withorder_id
andcustomer_id
and a newproducts
table withproduct_id
,product_name
, andproduct_price
. Theorders
table would then link to theproducts
table viaproduct_id
.
Partitioning
Partitioning is another strategy that can help optimize data. By dividing large tables into smaller, more manageable pieces, performance can be improved. Partitioning can be done by range, list, or hash. Here’s how you can apply partitioning in SQL:
ALTER TABLE transactions PARTITION BY RANGE(year(transaction_date)) ( PARTITION p0 VALUES LESS THAN (1991), PARTITION p1 VALUES LESS THAN (1992), PARTITION p2 VALUES LESS THAN (1993), PARTITION p3 VALUES LESS THAN MAXVALUE );
Partitioning can make certain types of queries much faster, as the query engine can target only specific partitions instead of scanning the entire table.
Implementing these SQL strategies for data optimization can significantly enhance the performance and efficiency of your database. Indexing, query optimization, normalization, and partitioning are powerful techniques that, when used correctly, can make a substantial difference in how your data is handled and accessed.